Example 5 - Reproducing ArchKalmag14k¶
In this notebook we show how to reproduce the model ArchKalmag14k.r and import it in pymagglobal. We also distribute ArchKalmag14k.r this way. Additional access is provided via a dedicated website.
Set up the data¶
We first set up the data.
[1]:
import io
import requests
import numpy as np
# load the rejection list
rejection_response = requests.get(
'https://nextcloud.gfz-potsdam.de/s/'
'WLxDTddq663zFLP/download/rejection_list.npz',
)
rejection_response.raise_for_status()
with np.load(io.BytesIO(rejection_response.content), allow_pickle=True) as fh:
to_reject = fh['to_reject']
[2]:
from paleokalmag import ChunkedData
from paleokalmag.data_handling import read_data
data_response = requests.get(
'https://nextcloud.gfz-potsdam.de/s/'
'r6YxrrABRJjideS/download/archkalmag_data.csv',
)
data_response.raise_for_status()
# Since we load the data from the web, we read it manually
# and pass the dataframe to ChunkedData
data = read_data(
io.BytesIO(data_response.content),
rejection_lists=to_reject,
)
cdat = ChunkedData(
data,
delta_t=10,
)
Rejected 347 outliers.
/builds/sec23/korte/paleokalmag/paleokalmag/data_handling.py:160: FutureWarning: A value is trying to be set on a copy of a DataFrame or Series through chained assignment using an inplace method.
The behavior will change in pandas 3.0. This inplace method will never work because the intermediate object on which we are setting values always behaves as a copy.
For example, when doing 'df[col].method(value, inplace=True)', try using 'df.method({col: value}, inplace=True)' or df[col] = df[col].method(value) instead, to perform the operation inplace on the original object.
/builds/sec23/korte/paleokalmag/paleokalmag/data_handling.py:166: FutureWarning: A value is trying to be set on a copy of a DataFrame or Series through chained assignment using an inplace method.
The behavior will change in pandas 3.0. This inplace method will never work because the intermediate object on which we are setting values always behaves as a copy.
For example, when doing 'df[col].method(value, inplace=True)', try using 'df.method({col: value}, inplace=True)' or df[col] = df[col].method(value) instead, to perform the operation inplace on the original object.
/builds/sec23/korte/paleokalmag/paleokalmag/data_handling.py:180: UserWarning: Records with indices [ 72 73 74 75 76 77 78 79 80 81 108 109
357 358 1065 1066 1153 2402 2575 2671 2753 2756 2757 3036
3215 3217 3218 3219 3220 3221 3222 3223 3224 3225 3503 3772
3988 4047 4235 4415 4504 4530 4531 4655 5172 5326 5434 5496
5551 5707 5763 5802 5805 5959 6053 6105 6647 6706 6781 6809
7149 7580 7585 7593 7692 7702 7735 7776 7806 7826 7881 7979
8067 8296 8327 8427 8464 8510 8670 8708 8752 9224 9653 10002
10132 10331 10509 10604 10647 11527 11758] contain declination, but not inclination! The errors need special treatment!
To be able to use the provided data, these records have been dropped from the output.
/builds/sec23/korte/paleokalmag/paleokalmag/data_handling.py:189: FutureWarning: A value is trying to be set on a copy of a DataFrame or Series through chained assignment using an inplace method.
The behavior will change in pandas 3.0. This inplace method will never work because the intermediate object on which we are setting values always behaves as a copy.
For example, when doing 'df[col].method(value, inplace=True)', try using 'df.method({col: value}, inplace=True)' or df[col] = df[col].method(value) instead, to perform the operation inplace on the original object.
/builds/sec23/korte/paleokalmag/paleokalmag/data_handling.py:197: FutureWarning: A value is trying to be set on a copy of a DataFrame or Series through chained assignment using an inplace method.
The behavior will change in pandas 3.0. This inplace method will never work because the intermediate object on which we are setting values always behaves as a copy.
For example, when doing 'df[col].method(value, inplace=True)', try using 'df.method({col: value}, inplace=True)' or df[col] = df[col].method(value) instead, to perform the operation inplace on the original object.
/builds/sec23/korte/paleokalmag/paleokalmag/data_handling.py:198: FutureWarning: A value is trying to be set on a copy of a DataFrame or Series through chained assignment using an inplace method.
The behavior will change in pandas 3.0. This inplace method will never work because the intermediate object on which we are setting values always behaves as a copy.
For example, when doing 'df[col].method(value, inplace=True)', try using 'df.method({col: value}, inplace=True)' or df[col] = df[col].method(value) instead, to perform the operation inplace on the original object.
Set up and run the model¶
We next set up a model with the estimated hyperparameters. They have been estimated using the procedure illustrated in example 3.
[3]:
from paleokalmag.corefieldmodel import CoreFieldModel
a14kModel = CoreFieldModel(
lmax=20,
R=2800,
gamma=-38.00,
alpha_dip=39.00,
tau_dip=171.34,
alpha_wodip=118.22,
tau_wodip=379.59,
rho=2.25,
)
Next we run the model:
[4]:
a14kModel.forward(cdat, output_every=5, quiet=False)
a14kModel.save('./ArchKalmag14k_forward.npz')
a14kModel.backward(quiet=False)
a14kModel.save('./ArchKalmag14k_smoothed.npz')
- more-to-come:
- class:
stderr
- 0%| | 0/1400 [00:00<?, ?it/s]
</pre>
- 0%| | 0/1400 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/1400 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/1400 [00:00<03:43, 6.26it/s]
</pre>
- 0%| | 1/1400 [00:00<03:43, 6.26it/s]
end{sphinxVerbatim}
0%| | 1/1400 [00:00<03:43, 6.26it/s]
- more-to-come:
- class:
stderr
- 0%| | 2/1400 [00:00<03:30, 6.65it/s]
</pre>
- 0%| | 2/1400 [00:00<03:30, 6.65it/s]
end{sphinxVerbatim}
0%| | 2/1400 [00:00<03:30, 6.65it/s]
- more-to-come:
- class:
stderr
- 0%| | 3/1400 [00:00<03:21, 6.92it/s]
</pre>
- 0%| | 3/1400 [00:00<03:21, 6.92it/s]
end{sphinxVerbatim}
0%| | 3/1400 [00:00<03:21, 6.92it/s]
- more-to-come:
- class:
stderr
- 0%| | 4/1400 [00:00<03:30, 6.62it/s]
</pre>
- 0%| | 4/1400 [00:00<03:30, 6.62it/s]
end{sphinxVerbatim}
0%| | 4/1400 [00:00<03:30, 6.62it/s]
- more-to-come:
- class:
stderr
- 0%| | 5/1400 [00:00<03:35, 6.48it/s]
</pre>
- 0%| | 5/1400 [00:00<03:35, 6.48it/s]
end{sphinxVerbatim}
0%| | 5/1400 [00:00<03:35, 6.48it/s]
- more-to-come:
- class:
stderr
- 0%| | 6/1400 [00:00<03:35, 6.47it/s]
</pre>
- 0%| | 6/1400 [00:00<03:35, 6.47it/s]
end{sphinxVerbatim}
0%| | 6/1400 [00:00<03:35, 6.47it/s]
- more-to-come:
- class:
stderr
- 0%| | 7/1400 [00:01<03:19, 6.98it/s]
</pre>
- 0%| | 7/1400 [00:01<03:19, 6.98it/s]
end{sphinxVerbatim}
0%| | 7/1400 [00:01<03:19, 6.98it/s]
- more-to-come:
- class:
stderr
- 1%| | 8/1400 [00:01<03:16, 7.07it/s]
</pre>
- 1%| | 8/1400 [00:01<03:16, 7.07it/s]
end{sphinxVerbatim}
1%| | 8/1400 [00:01<03:16, 7.07it/s]
- more-to-come:
- class:
stderr
- 1%| | 9/1400 [00:01<03:16, 7.08it/s]
</pre>
- 1%| | 9/1400 [00:01<03:16, 7.08it/s]
end{sphinxVerbatim}
1%| | 9/1400 [00:01<03:16, 7.08it/s]
- more-to-come:
- class:
stderr
- 1%| | 10/1400 [00:01<03:18, 7.01it/s]
</pre>
- 1%| | 10/1400 [00:01<03:18, 7.01it/s]
end{sphinxVerbatim}
1%| | 10/1400 [00:01<03:18, 7.01it/s]
- more-to-come:
- class:
stderr
- 1%| | 11/1400 [00:01<03:16, 7.08it/s]
</pre>
- 1%| | 11/1400 [00:01<03:16, 7.08it/s]
end{sphinxVerbatim}
1%| | 11/1400 [00:01<03:16, 7.08it/s]
- more-to-come:
- class:
stderr
- 1%| | 12/1400 [00:01<03:18, 7.01it/s]
</pre>
- 1%| | 12/1400 [00:01<03:18, 7.01it/s]
end{sphinxVerbatim}
1%| | 12/1400 [00:01<03:18, 7.01it/s]
- more-to-come:
- class:
stderr
- 1%| | 13/1400 [00:01<03:23, 6.81it/s]
</pre>
- 1%| | 13/1400 [00:01<03:23, 6.81it/s]
end{sphinxVerbatim}
1%| | 13/1400 [00:01<03:23, 6.81it/s]
- more-to-come:
- class:
stderr
- 1%| | 14/1400 [00:02<03:24, 6.76it/s]
</pre>
- 1%| | 14/1400 [00:02<03:24, 6.76it/s]
end{sphinxVerbatim}
1%| | 14/1400 [00:02<03:24, 6.76it/s]
- more-to-come:
- class:
stderr
- 1%| | 15/1400 [00:02<03:32, 6.52it/s]
</pre>
- 1%| | 15/1400 [00:02<03:32, 6.52it/s]
end{sphinxVerbatim}
1%| | 15/1400 [00:02<03:32, 6.52it/s]
- more-to-come:
- class:
stderr
- 1%| | 16/1400 [00:02<03:30, 6.56it/s]
</pre>
- 1%| | 16/1400 [00:02<03:30, 6.56it/s]
end{sphinxVerbatim}
1%| | 16/1400 [00:02<03:30, 6.56it/s]
- more-to-come:
- class:
stderr
- 1%| | 17/1400 [00:02<03:32, 6.50it/s]
</pre>
- 1%| | 17/1400 [00:02<03:32, 6.50it/s]
end{sphinxVerbatim}
1%| | 17/1400 [00:02<03:32, 6.50it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 18/1400 [00:02<03:29, 6.61it/s]
</pre>
- 1%|▏ | 18/1400 [00:02<03:29, 6.61it/s]
end{sphinxVerbatim}
1%|▏ | 18/1400 [00:02<03:29, 6.61it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 19/1400 [00:02<03:23, 6.77it/s]
</pre>
- 1%|▏ | 19/1400 [00:02<03:23, 6.77it/s]
end{sphinxVerbatim}
1%|▏ | 19/1400 [00:02<03:23, 6.77it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 20/1400 [00:02<03:29, 6.58it/s]
</pre>
- 1%|▏ | 20/1400 [00:02<03:29, 6.58it/s]
end{sphinxVerbatim}
1%|▏ | 20/1400 [00:02<03:29, 6.58it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 21/1400 [00:03<03:39, 6.28it/s]
</pre>
- 2%|▏ | 21/1400 [00:03<03:39, 6.28it/s]
end{sphinxVerbatim}
2%|▏ | 21/1400 [00:03<03:39, 6.28it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 22/1400 [00:03<03:43, 6.17it/s]
</pre>
- 2%|▏ | 22/1400 [00:03<03:43, 6.17it/s]
end{sphinxVerbatim}
2%|▏ | 22/1400 [00:03<03:43, 6.17it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 23/1400 [00:03<03:33, 6.44it/s]
</pre>
- 2%|▏ | 23/1400 [00:03<03:33, 6.44it/s]
end{sphinxVerbatim}
2%|▏ | 23/1400 [00:03<03:33, 6.44it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 24/1400 [00:03<03:37, 6.31it/s]
</pre>
- 2%|▏ | 24/1400 [00:03<03:37, 6.31it/s]
end{sphinxVerbatim}
2%|▏ | 24/1400 [00:03<03:37, 6.31it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 25/1400 [00:03<03:58, 5.76it/s]
</pre>
- 2%|▏ | 25/1400 [00:03<03:58, 5.76it/s]
end{sphinxVerbatim}
2%|▏ | 25/1400 [00:03<03:58, 5.76it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 26/1400 [00:03<03:42, 6.18it/s]
</pre>
- 2%|▏ | 26/1400 [00:03<03:42, 6.18it/s]
end{sphinxVerbatim}
2%|▏ | 26/1400 [00:03<03:42, 6.18it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 27/1400 [00:04<03:35, 6.38it/s]
</pre>
- 2%|▏ | 27/1400 [00:04<03:35, 6.38it/s]
end{sphinxVerbatim}
2%|▏ | 27/1400 [00:04<03:35, 6.38it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 28/1400 [00:04<03:33, 6.44it/s]
</pre>
- 2%|▏ | 28/1400 [00:04<03:33, 6.44it/s]
end{sphinxVerbatim}
2%|▏ | 28/1400 [00:04<03:33, 6.44it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 29/1400 [00:04<03:22, 6.78it/s]
</pre>
- 2%|▏ | 29/1400 [00:04<03:22, 6.78it/s]
end{sphinxVerbatim}
2%|▏ | 29/1400 [00:04<03:22, 6.78it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 30/1400 [00:04<03:46, 6.05it/s]
</pre>
- 2%|▏ | 30/1400 [00:04<03:46, 6.05it/s]
end{sphinxVerbatim}
2%|▏ | 30/1400 [00:04<03:46, 6.05it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 31/1400 [00:04<03:36, 6.33it/s]
</pre>
- 2%|▏ | 31/1400 [00:04<03:36, 6.33it/s]
end{sphinxVerbatim}
2%|▏ | 31/1400 [00:04<03:36, 6.33it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 32/1400 [00:04<03:30, 6.50it/s]
</pre>
- 2%|▏ | 32/1400 [00:04<03:30, 6.50it/s]
end{sphinxVerbatim}
2%|▏ | 32/1400 [00:04<03:30, 6.50it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 33/1400 [00:05<03:26, 6.62it/s]
</pre>
- 2%|▏ | 33/1400 [00:05<03:26, 6.62it/s]
end{sphinxVerbatim}
2%|▏ | 33/1400 [00:05<03:26, 6.62it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 34/1400 [00:05<03:30, 6.47it/s]
</pre>
- 2%|▏ | 34/1400 [00:05<03:30, 6.47it/s]
end{sphinxVerbatim}
2%|▏ | 34/1400 [00:05<03:30, 6.47it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 35/1400 [00:05<03:41, 6.15it/s]
</pre>
- 2%|▎ | 35/1400 [00:05<03:41, 6.15it/s]
end{sphinxVerbatim}
2%|▎ | 35/1400 [00:05<03:41, 6.15it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 36/1400 [00:05<03:35, 6.34it/s]
</pre>
- 3%|▎ | 36/1400 [00:05<03:35, 6.34it/s]
end{sphinxVerbatim}
3%|▎ | 36/1400 [00:05<03:35, 6.34it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 37/1400 [00:05<03:29, 6.52it/s]
</pre>
- 3%|▎ | 37/1400 [00:05<03:29, 6.52it/s]
end{sphinxVerbatim}
3%|▎ | 37/1400 [00:05<03:29, 6.52it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 38/1400 [00:05<03:21, 6.76it/s]
</pre>
- 3%|▎ | 38/1400 [00:05<03:21, 6.76it/s]
end{sphinxVerbatim}
3%|▎ | 38/1400 [00:05<03:21, 6.76it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 39/1400 [00:05<03:14, 6.99it/s]
</pre>
- 3%|▎ | 39/1400 [00:05<03:14, 6.99it/s]
end{sphinxVerbatim}
3%|▎ | 39/1400 [00:05<03:14, 6.99it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 40/1400 [00:06<03:13, 7.03it/s]
</pre>
- 3%|▎ | 40/1400 [00:06<03:13, 7.03it/s]
end{sphinxVerbatim}
3%|▎ | 40/1400 [00:06<03:13, 7.03it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 41/1400 [00:06<03:05, 7.33it/s]
</pre>
- 3%|▎ | 41/1400 [00:06<03:05, 7.33it/s]
end{sphinxVerbatim}
3%|▎ | 41/1400 [00:06<03:05, 7.33it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 42/1400 [00:06<03:01, 7.46it/s]
</pre>
- 3%|▎ | 42/1400 [00:06<03:01, 7.46it/s]
end{sphinxVerbatim}
3%|▎ | 42/1400 [00:06<03:01, 7.46it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 43/1400 [00:06<02:59, 7.54it/s]
</pre>
- 3%|▎ | 43/1400 [00:06<02:59, 7.54it/s]
end{sphinxVerbatim}
3%|▎ | 43/1400 [00:06<02:59, 7.54it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 44/1400 [00:06<02:58, 7.61it/s]
</pre>
- 3%|▎ | 44/1400 [00:06<02:58, 7.61it/s]
end{sphinxVerbatim}
3%|▎ | 44/1400 [00:06<02:58, 7.61it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 45/1400 [00:06<03:16, 6.89it/s]
</pre>
- 3%|▎ | 45/1400 [00:06<03:16, 6.89it/s]
end{sphinxVerbatim}
3%|▎ | 45/1400 [00:06<03:16, 6.89it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 46/1400 [00:06<03:18, 6.80it/s]
</pre>
- 3%|▎ | 46/1400 [00:06<03:18, 6.80it/s]
end{sphinxVerbatim}
3%|▎ | 46/1400 [00:06<03:18, 6.80it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 47/1400 [00:07<03:16, 6.89it/s]
</pre>
- 3%|▎ | 47/1400 [00:07<03:16, 6.89it/s]
end{sphinxVerbatim}
3%|▎ | 47/1400 [00:07<03:16, 6.89it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 48/1400 [00:07<03:09, 7.14it/s]
</pre>
- 3%|▎ | 48/1400 [00:07<03:09, 7.14it/s]
end{sphinxVerbatim}
3%|▎ | 48/1400 [00:07<03:09, 7.14it/s]
- more-to-come:
- class:
stderr
- 4%|▎ | 49/1400 [00:07<03:05, 7.30it/s]
</pre>
- 4%|▎ | 49/1400 [00:07<03:05, 7.30it/s]
end{sphinxVerbatim}
4%|▎ | 49/1400 [00:07<03:05, 7.30it/s]
- more-to-come:
- class:
stderr
- 4%|▎ | 50/1400 [00:07<03:09, 7.12it/s]
</pre>
- 4%|▎ | 50/1400 [00:07<03:09, 7.12it/s]
end{sphinxVerbatim}
4%|▎ | 50/1400 [00:07<03:09, 7.12it/s]
- more-to-come:
- class:
stderr
- 4%|▎ | 51/1400 [00:07<03:01, 7.44it/s]
</pre>
- 4%|▎ | 51/1400 [00:07<03:01, 7.44it/s]
end{sphinxVerbatim}
4%|▎ | 51/1400 [00:07<03:01, 7.44it/s]
- more-to-come:
- class:
stderr
- 4%|▎ | 52/1400 [00:07<02:54, 7.71it/s]
</pre>
- 4%|▎ | 52/1400 [00:07<02:54, 7.71it/s]
end{sphinxVerbatim}
4%|▎ | 52/1400 [00:07<02:54, 7.71it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 53/1400 [00:07<02:54, 7.72it/s]
</pre>
- 4%|▍ | 53/1400 [00:07<02:54, 7.72it/s]
end{sphinxVerbatim}
4%|▍ | 53/1400 [00:07<02:54, 7.72it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 54/1400 [00:07<02:53, 7.77it/s]
</pre>
- 4%|▍ | 54/1400 [00:07<02:53, 7.77it/s]
end{sphinxVerbatim}
4%|▍ | 54/1400 [00:07<02:53, 7.77it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 55/1400 [00:08<03:26, 6.50it/s]
</pre>
- 4%|▍ | 55/1400 [00:08<03:26, 6.50it/s]
end{sphinxVerbatim}
4%|▍ | 55/1400 [00:08<03:26, 6.50it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 56/1400 [00:08<03:29, 6.42it/s]
</pre>
- 4%|▍ | 56/1400 [00:08<03:29, 6.42it/s]
end{sphinxVerbatim}
4%|▍ | 56/1400 [00:08<03:29, 6.42it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 57/1400 [00:08<03:23, 6.59it/s]
</pre>
- 4%|▍ | 57/1400 [00:08<03:23, 6.59it/s]
end{sphinxVerbatim}
4%|▍ | 57/1400 [00:08<03:23, 6.59it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 58/1400 [00:08<03:19, 6.74it/s]
</pre>
- 4%|▍ | 58/1400 [00:08<03:19, 6.74it/s]
end{sphinxVerbatim}
4%|▍ | 58/1400 [00:08<03:19, 6.74it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 59/1400 [00:08<03:13, 6.94it/s]
</pre>
- 4%|▍ | 59/1400 [00:08<03:13, 6.94it/s]
end{sphinxVerbatim}
4%|▍ | 59/1400 [00:08<03:13, 6.94it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 60/1400 [00:08<03:34, 6.24it/s]
</pre>
- 4%|▍ | 60/1400 [00:08<03:34, 6.24it/s]
end{sphinxVerbatim}
4%|▍ | 60/1400 [00:08<03:34, 6.24it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 61/1400 [00:09<03:22, 6.61it/s]
</pre>
- 4%|▍ | 61/1400 [00:09<03:22, 6.61it/s]
end{sphinxVerbatim}
4%|▍ | 61/1400 [00:09<03:22, 6.61it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 62/1400 [00:09<03:15, 6.84it/s]
</pre>
- 4%|▍ | 62/1400 [00:09<03:15, 6.84it/s]
end{sphinxVerbatim}
4%|▍ | 62/1400 [00:09<03:15, 6.84it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 63/1400 [00:09<03:10, 7.03it/s]
</pre>
- 4%|▍ | 63/1400 [00:09<03:10, 7.03it/s]
end{sphinxVerbatim}
4%|▍ | 63/1400 [00:09<03:10, 7.03it/s]
- more-to-come:
- class:
stderr
- 5%|▍ | 64/1400 [00:09<03:02, 7.33it/s]
</pre>
- 5%|▍ | 64/1400 [00:09<03:02, 7.33it/s]
end{sphinxVerbatim}
5%|▍ | 64/1400 [00:09<03:02, 7.33it/s]
- more-to-come:
- class:
stderr
- 5%|▍ | 65/1400 [00:09<03:16, 6.79it/s]
</pre>
- 5%|▍ | 65/1400 [00:09<03:16, 6.79it/s]
end{sphinxVerbatim}
5%|▍ | 65/1400 [00:09<03:16, 6.79it/s]
- more-to-come:
- class:
stderr
- 5%|▍ | 66/1400 [00:09<03:06, 7.14it/s]
</pre>
- 5%|▍ | 66/1400 [00:09<03:06, 7.14it/s]
end{sphinxVerbatim}
5%|▍ | 66/1400 [00:09<03:06, 7.14it/s]
- more-to-come:
- class:
stderr
- 5%|▍ | 67/1400 [00:09<03:02, 7.32it/s]
</pre>
- 5%|▍ | 67/1400 [00:09<03:02, 7.32it/s]
end{sphinxVerbatim}
5%|▍ | 67/1400 [00:09<03:02, 7.32it/s]
- more-to-come:
- class:
stderr
- 5%|▍ | 68/1400 [00:10<03:12, 6.90it/s]
</pre>
- 5%|▍ | 68/1400 [00:10<03:12, 6.90it/s]
end{sphinxVerbatim}
5%|▍ | 68/1400 [00:10<03:12, 6.90it/s]
- more-to-come:
- class:
stderr
- 5%|▍ | 69/1400 [00:10<03:08, 7.08it/s]
</pre>
- 5%|▍ | 69/1400 [00:10<03:08, 7.08it/s]
end{sphinxVerbatim}
5%|▍ | 69/1400 [00:10<03:08, 7.08it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 70/1400 [00:10<03:27, 6.40it/s]
</pre>
- 5%|▌ | 70/1400 [00:10<03:27, 6.40it/s]
end{sphinxVerbatim}
5%|▌ | 70/1400 [00:10<03:27, 6.40it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 71/1400 [00:10<03:23, 6.53it/s]
</pre>
- 5%|▌ | 71/1400 [00:10<03:23, 6.53it/s]
end{sphinxVerbatim}
5%|▌ | 71/1400 [00:10<03:23, 6.53it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 72/1400 [00:10<03:18, 6.68it/s]
</pre>
- 5%|▌ | 72/1400 [00:10<03:18, 6.68it/s]
end{sphinxVerbatim}
5%|▌ | 72/1400 [00:10<03:18, 6.68it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 73/1400 [00:10<03:20, 6.61it/s]
</pre>
- 5%|▌ | 73/1400 [00:10<03:20, 6.61it/s]
end{sphinxVerbatim}
5%|▌ | 73/1400 [00:10<03:20, 6.61it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 74/1400 [00:10<03:19, 6.65it/s]
</pre>
- 5%|▌ | 74/1400 [00:10<03:19, 6.65it/s]
end{sphinxVerbatim}
5%|▌ | 74/1400 [00:10<03:19, 6.65it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 75/1400 [00:11<03:30, 6.28it/s]
</pre>
- 5%|▌ | 75/1400 [00:11<03:30, 6.28it/s]
end{sphinxVerbatim}
5%|▌ | 75/1400 [00:11<03:30, 6.28it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 76/1400 [00:11<03:18, 6.67it/s]
</pre>
- 5%|▌ | 76/1400 [00:11<03:18, 6.67it/s]
end{sphinxVerbatim}
5%|▌ | 76/1400 [00:11<03:18, 6.67it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 77/1400 [00:11<03:06, 7.08it/s]
</pre>
- 6%|▌ | 77/1400 [00:11<03:06, 7.08it/s]
end{sphinxVerbatim}
6%|▌ | 77/1400 [00:11<03:06, 7.08it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 78/1400 [00:11<03:09, 6.96it/s]
</pre>
- 6%|▌ | 78/1400 [00:11<03:09, 6.96it/s]
end{sphinxVerbatim}
6%|▌ | 78/1400 [00:11<03:09, 6.96it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 79/1400 [00:11<03:03, 7.19it/s]
</pre>
- 6%|▌ | 79/1400 [00:11<03:03, 7.19it/s]
end{sphinxVerbatim}
6%|▌ | 79/1400 [00:11<03:03, 7.19it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 80/1400 [00:11<03:10, 6.94it/s]
</pre>
- 6%|▌ | 80/1400 [00:11<03:10, 6.94it/s]
end{sphinxVerbatim}
6%|▌ | 80/1400 [00:11<03:10, 6.94it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 81/1400 [00:11<03:02, 7.22it/s]
</pre>
- 6%|▌ | 81/1400 [00:11<03:02, 7.22it/s]
end{sphinxVerbatim}
6%|▌ | 81/1400 [00:11<03:02, 7.22it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 82/1400 [00:12<02:58, 7.37it/s]
</pre>
- 6%|▌ | 82/1400 [00:12<02:58, 7.37it/s]
end{sphinxVerbatim}
6%|▌ | 82/1400 [00:12<02:58, 7.37it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 83/1400 [00:12<02:59, 7.34it/s]
</pre>
- 6%|▌ | 83/1400 [00:12<02:59, 7.34it/s]
end{sphinxVerbatim}
6%|▌ | 83/1400 [00:12<02:59, 7.34it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 84/1400 [00:12<02:57, 7.42it/s]
</pre>
- 6%|▌ | 84/1400 [00:12<02:57, 7.42it/s]
end{sphinxVerbatim}
6%|▌ | 84/1400 [00:12<02:57, 7.42it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 85/1400 [00:12<03:11, 6.88it/s]
</pre>
- 6%|▌ | 85/1400 [00:12<03:11, 6.88it/s]
end{sphinxVerbatim}
6%|▌ | 85/1400 [00:12<03:11, 6.88it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 86/1400 [00:12<03:03, 7.17it/s]
</pre>
- 6%|▌ | 86/1400 [00:12<03:03, 7.17it/s]
end{sphinxVerbatim}
6%|▌ | 86/1400 [00:12<03:03, 7.17it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 87/1400 [00:12<03:01, 7.25it/s]
</pre>
- 6%|▌ | 87/1400 [00:12<03:01, 7.25it/s]
end{sphinxVerbatim}
6%|▌ | 87/1400 [00:12<03:01, 7.25it/s]
- more-to-come:
- class:
stderr
- 6%|▋ | 88/1400 [00:12<03:09, 6.91it/s]
</pre>
- 6%|▋ | 88/1400 [00:12<03:09, 6.91it/s]
end{sphinxVerbatim}
6%|▋ | 88/1400 [00:12<03:09, 6.91it/s]
- more-to-come:
- class:
stderr
- 6%|▋ | 89/1400 [00:13<03:12, 6.80it/s]
</pre>
- 6%|▋ | 89/1400 [00:13<03:12, 6.80it/s]
end{sphinxVerbatim}
6%|▋ | 89/1400 [00:13<03:12, 6.80it/s]
- more-to-come:
- class:
stderr
- 6%|▋ | 90/1400 [00:13<03:21, 6.50it/s]
</pre>
- 6%|▋ | 90/1400 [00:13<03:21, 6.50it/s]
end{sphinxVerbatim}
6%|▋ | 90/1400 [00:13<03:21, 6.50it/s]
- more-to-come:
- class:
stderr
- 6%|▋ | 91/1400 [00:13<03:10, 6.88it/s]
</pre>
- 6%|▋ | 91/1400 [00:13<03:10, 6.88it/s]
end{sphinxVerbatim}
6%|▋ | 91/1400 [00:13<03:10, 6.88it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 92/1400 [00:13<03:01, 7.20it/s]
</pre>
- 7%|▋ | 92/1400 [00:13<03:01, 7.20it/s]
end{sphinxVerbatim}
7%|▋ | 92/1400 [00:13<03:01, 7.20it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 93/1400 [00:13<03:00, 7.24it/s]
</pre>
- 7%|▋ | 93/1400 [00:13<03:00, 7.24it/s]
end{sphinxVerbatim}
7%|▋ | 93/1400 [00:13<03:00, 7.24it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 94/1400 [00:13<02:52, 7.56it/s]
</pre>
- 7%|▋ | 94/1400 [00:13<02:52, 7.56it/s]
end{sphinxVerbatim}
7%|▋ | 94/1400 [00:13<02:52, 7.56it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 95/1400 [00:13<03:02, 7.14it/s]
</pre>
- 7%|▋ | 95/1400 [00:13<03:02, 7.14it/s]
end{sphinxVerbatim}
7%|▋ | 95/1400 [00:13<03:02, 7.14it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 96/1400 [00:14<02:57, 7.35it/s]
</pre>
- 7%|▋ | 96/1400 [00:14<02:57, 7.35it/s]
end{sphinxVerbatim}
7%|▋ | 96/1400 [00:14<02:57, 7.35it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 97/1400 [00:14<02:49, 7.69it/s]
</pre>
- 7%|▋ | 97/1400 [00:14<02:49, 7.69it/s]
end{sphinxVerbatim}
7%|▋ | 97/1400 [00:14<02:49, 7.69it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 98/1400 [00:14<02:49, 7.68it/s]
</pre>
- 7%|▋ | 98/1400 [00:14<02:49, 7.68it/s]
end{sphinxVerbatim}
7%|▋ | 98/1400 [00:14<02:49, 7.68it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 99/1400 [00:14<02:43, 7.94it/s]
</pre>
- 7%|▋ | 99/1400 [00:14<02:43, 7.94it/s]
end{sphinxVerbatim}
7%|▋ | 99/1400 [00:14<02:43, 7.94it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 100/1400 [00:14<02:53, 7.51it/s]
</pre>
- 7%|▋ | 100/1400 [00:14<02:53, 7.51it/s]
end{sphinxVerbatim}
7%|▋ | 100/1400 [00:14<02:53, 7.51it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 101/1400 [00:14<02:47, 7.76it/s]
</pre>
- 7%|▋ | 101/1400 [00:14<02:47, 7.76it/s]
end{sphinxVerbatim}
7%|▋ | 101/1400 [00:14<02:47, 7.76it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 102/1400 [00:14<02:45, 7.84it/s]
</pre>
- 7%|▋ | 102/1400 [00:14<02:45, 7.84it/s]
end{sphinxVerbatim}
7%|▋ | 102/1400 [00:14<02:45, 7.84it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 103/1400 [00:14<02:51, 7.55it/s]
</pre>
- 7%|▋ | 103/1400 [00:14<02:51, 7.55it/s]
end{sphinxVerbatim}
7%|▋ | 103/1400 [00:14<02:51, 7.55it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 104/1400 [00:15<02:51, 7.55it/s]
</pre>
- 7%|▋ | 104/1400 [00:15<02:51, 7.55it/s]
end{sphinxVerbatim}
7%|▋ | 104/1400 [00:15<02:51, 7.55it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 105/1400 [00:15<03:11, 6.78it/s]
</pre>
- 8%|▊ | 105/1400 [00:15<03:11, 6.78it/s]
end{sphinxVerbatim}
8%|▊ | 105/1400 [00:15<03:11, 6.78it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 106/1400 [00:15<03:01, 7.13it/s]
</pre>
- 8%|▊ | 106/1400 [00:15<03:01, 7.13it/s]
end{sphinxVerbatim}
8%|▊ | 106/1400 [00:15<03:01, 7.13it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 107/1400 [00:15<03:13, 6.67it/s]
</pre>
- 8%|▊ | 107/1400 [00:15<03:13, 6.67it/s]
end{sphinxVerbatim}
8%|▊ | 107/1400 [00:15<03:13, 6.67it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 108/1400 [00:15<03:06, 6.95it/s]
</pre>
- 8%|▊ | 108/1400 [00:15<03:06, 6.95it/s]
end{sphinxVerbatim}
8%|▊ | 108/1400 [00:15<03:06, 6.95it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 109/1400 [00:15<02:56, 7.30it/s]
</pre>
- 8%|▊ | 109/1400 [00:15<02:56, 7.30it/s]
end{sphinxVerbatim}
8%|▊ | 109/1400 [00:15<02:56, 7.30it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 110/1400 [00:15<03:14, 6.62it/s]
</pre>
- 8%|▊ | 110/1400 [00:15<03:14, 6.62it/s]
end{sphinxVerbatim}
8%|▊ | 110/1400 [00:15<03:14, 6.62it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 111/1400 [00:16<03:10, 6.77it/s]
</pre>
- 8%|▊ | 111/1400 [00:16<03:10, 6.77it/s]
end{sphinxVerbatim}
8%|▊ | 111/1400 [00:16<03:10, 6.77it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 112/1400 [00:16<03:09, 6.81it/s]
</pre>
- 8%|▊ | 112/1400 [00:16<03:09, 6.81it/s]
end{sphinxVerbatim}
8%|▊ | 112/1400 [00:16<03:09, 6.81it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 113/1400 [00:16<03:15, 6.58it/s]
</pre>
- 8%|▊ | 113/1400 [00:16<03:15, 6.58it/s]
end{sphinxVerbatim}
8%|▊ | 113/1400 [00:16<03:15, 6.58it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 114/1400 [00:16<03:09, 6.79it/s]
</pre>
- 8%|▊ | 114/1400 [00:16<03:09, 6.79it/s]
end{sphinxVerbatim}
8%|▊ | 114/1400 [00:16<03:09, 6.79it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 115/1400 [00:16<03:30, 6.11it/s]
</pre>
- 8%|▊ | 115/1400 [00:16<03:30, 6.11it/s]
end{sphinxVerbatim}
8%|▊ | 115/1400 [00:16<03:30, 6.11it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 116/1400 [00:16<03:14, 6.59it/s]
</pre>
- 8%|▊ | 116/1400 [00:16<03:14, 6.59it/s]
end{sphinxVerbatim}
8%|▊ | 116/1400 [00:16<03:14, 6.59it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 117/1400 [00:17<03:06, 6.86it/s]
</pre>
- 8%|▊ | 117/1400 [00:17<03:06, 6.86it/s]
end{sphinxVerbatim}
8%|▊ | 117/1400 [00:17<03:06, 6.86it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 118/1400 [00:17<02:58, 7.18it/s]
</pre>
- 8%|▊ | 118/1400 [00:17<02:58, 7.18it/s]
end{sphinxVerbatim}
8%|▊ | 118/1400 [00:17<02:58, 7.18it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 119/1400 [00:17<02:52, 7.42it/s]
</pre>
- 8%|▊ | 119/1400 [00:17<02:52, 7.42it/s]
end{sphinxVerbatim}
8%|▊ | 119/1400 [00:17<02:52, 7.42it/s]
- more-to-come:
- class:
stderr
- 9%|▊ | 120/1400 [00:17<03:02, 7.00it/s]
</pre>
- 9%|▊ | 120/1400 [00:17<03:02, 7.00it/s]
end{sphinxVerbatim}
9%|▊ | 120/1400 [00:17<03:02, 7.00it/s]
- more-to-come:
- class:
stderr
- 9%|▊ | 121/1400 [00:17<02:55, 7.30it/s]
</pre>
- 9%|▊ | 121/1400 [00:17<02:55, 7.30it/s]
end{sphinxVerbatim}
9%|▊ | 121/1400 [00:17<02:55, 7.30it/s]
- more-to-come:
- class:
stderr
- 9%|▊ | 122/1400 [00:17<03:00, 7.09it/s]
</pre>
- 9%|▊ | 122/1400 [00:17<03:00, 7.09it/s]
end{sphinxVerbatim}
9%|▊ | 122/1400 [00:17<03:00, 7.09it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 123/1400 [00:17<02:58, 7.15it/s]
</pre>
- 9%|▉ | 123/1400 [00:17<02:58, 7.15it/s]
end{sphinxVerbatim}
9%|▉ | 123/1400 [00:17<02:58, 7.15it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 124/1400 [00:18<03:02, 6.99it/s]
</pre>
- 9%|▉ | 124/1400 [00:18<03:02, 6.99it/s]
end{sphinxVerbatim}
9%|▉ | 124/1400 [00:18<03:02, 6.99it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 125/1400 [00:18<03:13, 6.60it/s]
</pre>
- 9%|▉ | 125/1400 [00:18<03:13, 6.60it/s]
end{sphinxVerbatim}
9%|▉ | 125/1400 [00:18<03:13, 6.60it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 126/1400 [00:18<03:02, 6.97it/s]
</pre>
- 9%|▉ | 126/1400 [00:18<03:02, 6.97it/s]
end{sphinxVerbatim}
9%|▉ | 126/1400 [00:18<03:02, 6.97it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 127/1400 [00:18<02:53, 7.32it/s]
</pre>
- 9%|▉ | 127/1400 [00:18<02:53, 7.32it/s]
end{sphinxVerbatim}
9%|▉ | 127/1400 [00:18<02:53, 7.32it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 128/1400 [00:18<03:12, 6.62it/s]
</pre>
- 9%|▉ | 128/1400 [00:18<03:12, 6.62it/s]
end{sphinxVerbatim}
9%|▉ | 128/1400 [00:18<03:12, 6.62it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 129/1400 [00:18<03:03, 6.91it/s]
</pre>
- 9%|▉ | 129/1400 [00:18<03:03, 6.91it/s]
end{sphinxVerbatim}
9%|▉ | 129/1400 [00:18<03:03, 6.91it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 130/1400 [00:18<03:09, 6.70it/s]
</pre>
- 9%|▉ | 130/1400 [00:18<03:09, 6.70it/s]
end{sphinxVerbatim}
9%|▉ | 130/1400 [00:18<03:09, 6.70it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 131/1400 [00:19<03:00, 7.02it/s]
</pre>
- 9%|▉ | 131/1400 [00:19<03:00, 7.02it/s]
end{sphinxVerbatim}
9%|▉ | 131/1400 [00:19<03:00, 7.02it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 132/1400 [00:19<02:50, 7.44it/s]
</pre>
- 9%|▉ | 132/1400 [00:19<02:50, 7.44it/s]
end{sphinxVerbatim}
9%|▉ | 132/1400 [00:19<02:50, 7.44it/s]
- more-to-come:
- class:
stderr
- 10%|▉ | 133/1400 [00:19<02:54, 7.25it/s]
</pre>
- 10%|▉ | 133/1400 [00:19<02:54, 7.25it/s]
end{sphinxVerbatim}
10%|▉ | 133/1400 [00:19<02:54, 7.25it/s]
- more-to-come:
- class:
stderr
- 10%|▉ | 134/1400 [00:19<02:45, 7.63it/s]
</pre>
- 10%|▉ | 134/1400 [00:19<02:45, 7.63it/s]
end{sphinxVerbatim}
10%|▉ | 134/1400 [00:19<02:45, 7.63it/s]
- more-to-come:
- class:
stderr
- 10%|▉ | 135/1400 [00:19<02:52, 7.35it/s]
</pre>
- 10%|▉ | 135/1400 [00:19<02:52, 7.35it/s]
end{sphinxVerbatim}
10%|▉ | 135/1400 [00:19<02:52, 7.35it/s]
- more-to-come:
- class:
stderr
- 10%|▉ | 136/1400 [00:19<02:45, 7.64it/s]
</pre>
- 10%|▉ | 136/1400 [00:19<02:45, 7.64it/s]
end{sphinxVerbatim}
10%|▉ | 136/1400 [00:19<02:45, 7.64it/s]
- more-to-come:
- class:
stderr
- 10%|▉ | 137/1400 [00:19<02:41, 7.83it/s]
</pre>
- 10%|▉ | 137/1400 [00:19<02:41, 7.83it/s]
end{sphinxVerbatim}
10%|▉ | 137/1400 [00:19<02:41, 7.83it/s]
- more-to-come:
- class:
stderr
- 10%|▉ | 138/1400 [00:19<02:37, 8.02it/s]
</pre>
- 10%|▉ | 138/1400 [00:19<02:37, 8.02it/s]
end{sphinxVerbatim}
10%|▉ | 138/1400 [00:19<02:37, 8.02it/s]
- more-to-come:
- class:
stderr
- 10%|▉ | 139/1400 [00:20<02:42, 7.76it/s]
</pre>
- 10%|▉ | 139/1400 [00:20<02:42, 7.76it/s]
end{sphinxVerbatim}
10%|▉ | 139/1400 [00:20<02:42, 7.76it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 140/1400 [00:20<02:59, 7.03it/s]
</pre>
- 10%|█ | 140/1400 [00:20<02:59, 7.03it/s]
end{sphinxVerbatim}
10%|█ | 140/1400 [00:20<02:59, 7.03it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 141/1400 [00:20<03:05, 6.78it/s]
</pre>
- 10%|█ | 141/1400 [00:20<03:05, 6.78it/s]
end{sphinxVerbatim}
10%|█ | 141/1400 [00:20<03:05, 6.78it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 142/1400 [00:20<03:02, 6.90it/s]
</pre>
- 10%|█ | 142/1400 [00:20<03:02, 6.90it/s]
end{sphinxVerbatim}
10%|█ | 142/1400 [00:20<03:02, 6.90it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 143/1400 [00:20<02:57, 7.06it/s]
</pre>
- 10%|█ | 143/1400 [00:20<02:57, 7.06it/s]
end{sphinxVerbatim}
10%|█ | 143/1400 [00:20<02:57, 7.06it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 144/1400 [00:20<02:53, 7.22it/s]
</pre>
- 10%|█ | 144/1400 [00:20<02:53, 7.22it/s]
end{sphinxVerbatim}
10%|█ | 144/1400 [00:20<02:53, 7.22it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 145/1400 [00:20<03:07, 6.69it/s]
</pre>
- 10%|█ | 145/1400 [00:20<03:07, 6.69it/s]
end{sphinxVerbatim}
10%|█ | 145/1400 [00:20<03:07, 6.69it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 146/1400 [00:21<02:56, 7.09it/s]
</pre>
- 10%|█ | 146/1400 [00:21<02:56, 7.09it/s]
end{sphinxVerbatim}
10%|█ | 146/1400 [00:21<02:56, 7.09it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 147/1400 [00:21<02:53, 7.24it/s]
</pre>
- 10%|█ | 147/1400 [00:21<02:53, 7.24it/s]
end{sphinxVerbatim}
10%|█ | 147/1400 [00:21<02:53, 7.24it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 148/1400 [00:21<02:55, 7.13it/s]
</pre>
- 11%|█ | 148/1400 [00:21<02:55, 7.13it/s]
end{sphinxVerbatim}
11%|█ | 148/1400 [00:21<02:55, 7.13it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 149/1400 [00:21<02:50, 7.34it/s]
</pre>
- 11%|█ | 149/1400 [00:21<02:50, 7.34it/s]
end{sphinxVerbatim}
11%|█ | 149/1400 [00:21<02:50, 7.34it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 150/1400 [00:21<02:53, 7.21it/s]
</pre>
- 11%|█ | 150/1400 [00:21<02:53, 7.21it/s]
end{sphinxVerbatim}
11%|█ | 150/1400 [00:21<02:53, 7.21it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 151/1400 [00:21<02:46, 7.49it/s]
</pre>
- 11%|█ | 151/1400 [00:21<02:46, 7.49it/s]
end{sphinxVerbatim}
11%|█ | 151/1400 [00:21<02:46, 7.49it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 152/1400 [00:21<02:41, 7.72it/s]
</pre>
- 11%|█ | 152/1400 [00:21<02:41, 7.72it/s]
end{sphinxVerbatim}
11%|█ | 152/1400 [00:21<02:41, 7.72it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 153/1400 [00:22<02:42, 7.66it/s]
</pre>
- 11%|█ | 153/1400 [00:22<02:42, 7.66it/s]
end{sphinxVerbatim}
11%|█ | 153/1400 [00:22<02:42, 7.66it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 154/1400 [00:22<02:37, 7.90it/s]
</pre>
- 11%|█ | 154/1400 [00:22<02:37, 7.90it/s]
end{sphinxVerbatim}
11%|█ | 154/1400 [00:22<02:37, 7.90it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 155/1400 [00:22<02:45, 7.54it/s]
</pre>
- 11%|█ | 155/1400 [00:22<02:45, 7.54it/s]
end{sphinxVerbatim}
11%|█ | 155/1400 [00:22<02:45, 7.54it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 156/1400 [00:22<02:42, 7.66it/s]
</pre>
- 11%|█ | 156/1400 [00:22<02:42, 7.66it/s]
end{sphinxVerbatim}
11%|█ | 156/1400 [00:22<02:42, 7.66it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 157/1400 [00:22<02:41, 7.69it/s]
</pre>
- 11%|█ | 157/1400 [00:22<02:41, 7.69it/s]
end{sphinxVerbatim}
11%|█ | 157/1400 [00:22<02:41, 7.69it/s]
- more-to-come:
- class:
stderr
- 11%|█▏ | 158/1400 [00:22<02:46, 7.44it/s]
</pre>
- 11%|█▏ | 158/1400 [00:22<02:46, 7.44it/s]
end{sphinxVerbatim}
11%|█▏ | 158/1400 [00:22<02:46, 7.44it/s]
- more-to-come:
- class:
stderr
- 11%|█▏ | 159/1400 [00:22<02:51, 7.25it/s]
</pre>
- 11%|█▏ | 159/1400 [00:22<02:51, 7.25it/s]
end{sphinxVerbatim}
11%|█▏ | 159/1400 [00:22<02:51, 7.25it/s]
- more-to-come:
- class:
stderr
- 11%|█▏ | 160/1400 [00:22<02:55, 7.05it/s]
</pre>
- 11%|█▏ | 160/1400 [00:22<02:55, 7.05it/s]
end{sphinxVerbatim}
11%|█▏ | 160/1400 [00:22<02:55, 7.05it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 161/1400 [00:23<02:49, 7.33it/s]
</pre>
- 12%|█▏ | 161/1400 [00:23<02:49, 7.33it/s]
end{sphinxVerbatim}
12%|█▏ | 161/1400 [00:23<02:49, 7.33it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 162/1400 [00:23<02:50, 7.26it/s]
</pre>
- 12%|█▏ | 162/1400 [00:23<02:50, 7.26it/s]
end{sphinxVerbatim}
12%|█▏ | 162/1400 [00:23<02:50, 7.26it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 163/1400 [00:23<02:49, 7.29it/s]
</pre>
- 12%|█▏ | 163/1400 [00:23<02:49, 7.29it/s]
end{sphinxVerbatim}
12%|█▏ | 163/1400 [00:23<02:49, 7.29it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 164/1400 [00:23<02:44, 7.54it/s]
</pre>
- 12%|█▏ | 164/1400 [00:23<02:44, 7.54it/s]
end{sphinxVerbatim}
12%|█▏ | 164/1400 [00:23<02:44, 7.54it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 165/1400 [00:23<02:50, 7.25it/s]
</pre>
- 12%|█▏ | 165/1400 [00:23<02:50, 7.25it/s]
end{sphinxVerbatim}
12%|█▏ | 165/1400 [00:23<02:50, 7.25it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 166/1400 [00:23<02:48, 7.31it/s]
</pre>
- 12%|█▏ | 166/1400 [00:23<02:48, 7.31it/s]
end{sphinxVerbatim}
12%|█▏ | 166/1400 [00:23<02:48, 7.31it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 167/1400 [00:23<02:45, 7.45it/s]
</pre>
- 12%|█▏ | 167/1400 [00:23<02:45, 7.45it/s]
end{sphinxVerbatim}
12%|█▏ | 167/1400 [00:23<02:45, 7.45it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 168/1400 [00:24<02:42, 7.60it/s]
</pre>
- 12%|█▏ | 168/1400 [00:24<02:42, 7.60it/s]
end{sphinxVerbatim}
12%|█▏ | 168/1400 [00:24<02:42, 7.60it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 169/1400 [00:24<02:42, 7.55it/s]
</pre>
- 12%|█▏ | 169/1400 [00:24<02:42, 7.55it/s]
end{sphinxVerbatim}
12%|█▏ | 169/1400 [00:24<02:42, 7.55it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 170/1400 [00:24<02:48, 7.31it/s]
</pre>
- 12%|█▏ | 170/1400 [00:24<02:48, 7.31it/s]
end{sphinxVerbatim}
12%|█▏ | 170/1400 [00:24<02:48, 7.31it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 171/1400 [00:24<02:44, 7.48it/s]
</pre>
- 12%|█▏ | 171/1400 [00:24<02:44, 7.48it/s]
end{sphinxVerbatim}
12%|█▏ | 171/1400 [00:24<02:44, 7.48it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 172/1400 [00:24<02:39, 7.71it/s]
</pre>
- 12%|█▏ | 172/1400 [00:24<02:39, 7.71it/s]
end{sphinxVerbatim}
12%|█▏ | 172/1400 [00:24<02:39, 7.71it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 173/1400 [00:24<02:40, 7.62it/s]
</pre>
- 12%|█▏ | 173/1400 [00:24<02:40, 7.62it/s]
end{sphinxVerbatim}
12%|█▏ | 173/1400 [00:24<02:40, 7.62it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 174/1400 [00:24<02:41, 7.59it/s]
</pre>
- 12%|█▏ | 174/1400 [00:24<02:41, 7.59it/s]
end{sphinxVerbatim}
12%|█▏ | 174/1400 [00:24<02:41, 7.59it/s]
- more-to-come:
- class:
stderr
- 12%|█▎ | 175/1400 [00:24<02:51, 7.16it/s]
</pre>
- 12%|█▎ | 175/1400 [00:24<02:51, 7.16it/s]
end{sphinxVerbatim}
12%|█▎ | 175/1400 [00:24<02:51, 7.16it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 176/1400 [00:25<02:42, 7.55it/s]
</pre>
- 13%|█▎ | 176/1400 [00:25<02:42, 7.55it/s]
end{sphinxVerbatim}
13%|█▎ | 176/1400 [00:25<02:42, 7.55it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 177/1400 [00:25<02:41, 7.58it/s]
</pre>
- 13%|█▎ | 177/1400 [00:25<02:41, 7.58it/s]
end{sphinxVerbatim}
13%|█▎ | 177/1400 [00:25<02:41, 7.58it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 178/1400 [00:25<02:50, 7.16it/s]
</pre>
- 13%|█▎ | 178/1400 [00:25<02:50, 7.16it/s]
end{sphinxVerbatim}
13%|█▎ | 178/1400 [00:25<02:50, 7.16it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 179/1400 [00:25<02:46, 7.31it/s]
</pre>
- 13%|█▎ | 179/1400 [00:25<02:46, 7.31it/s]
end{sphinxVerbatim}
13%|█▎ | 179/1400 [00:25<02:46, 7.31it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 180/1400 [00:25<03:01, 6.71it/s]
</pre>
- 13%|█▎ | 180/1400 [00:25<03:01, 6.71it/s]
end{sphinxVerbatim}
13%|█▎ | 180/1400 [00:25<03:01, 6.71it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 181/1400 [00:25<02:50, 7.16it/s]
</pre>
- 13%|█▎ | 181/1400 [00:25<02:50, 7.16it/s]
end{sphinxVerbatim}
13%|█▎ | 181/1400 [00:25<02:50, 7.16it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 182/1400 [00:25<02:46, 7.31it/s]
</pre>
- 13%|█▎ | 182/1400 [00:25<02:46, 7.31it/s]
end{sphinxVerbatim}
13%|█▎ | 182/1400 [00:25<02:46, 7.31it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 183/1400 [00:26<02:41, 7.54it/s]
</pre>
- 13%|█▎ | 183/1400 [00:26<02:41, 7.54it/s]
end{sphinxVerbatim}
13%|█▎ | 183/1400 [00:26<02:41, 7.54it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 184/1400 [00:26<02:45, 7.36it/s]
</pre>
- 13%|█▎ | 184/1400 [00:26<02:45, 7.36it/s]
end{sphinxVerbatim}
13%|█▎ | 184/1400 [00:26<02:45, 7.36it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 185/1400 [00:26<03:04, 6.60it/s]
</pre>
- 13%|█▎ | 185/1400 [00:26<03:04, 6.60it/s]
end{sphinxVerbatim}
13%|█▎ | 185/1400 [00:26<03:04, 6.60it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 186/1400 [00:26<02:56, 6.88it/s]
</pre>
- 13%|█▎ | 186/1400 [00:26<02:56, 6.88it/s]
end{sphinxVerbatim}
13%|█▎ | 186/1400 [00:26<02:56, 6.88it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 187/1400 [00:26<02:50, 7.12it/s]
</pre>
- 13%|█▎ | 187/1400 [00:26<02:50, 7.12it/s]
end{sphinxVerbatim}
13%|█▎ | 187/1400 [00:26<02:50, 7.12it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 188/1400 [00:26<02:49, 7.13it/s]
</pre>
- 13%|█▎ | 188/1400 [00:26<02:49, 7.13it/s]
end{sphinxVerbatim}
13%|█▎ | 188/1400 [00:26<02:49, 7.13it/s]
- more-to-come:
- class:
stderr
- 14%|█▎ | 189/1400 [00:26<02:45, 7.34it/s]
</pre>
- 14%|█▎ | 189/1400 [00:26<02:45, 7.34it/s]
end{sphinxVerbatim}
14%|█▎ | 189/1400 [00:26<02:45, 7.34it/s]
- more-to-come:
- class:
stderr
- 14%|█▎ | 190/1400 [00:27<02:49, 7.14it/s]
</pre>
- 14%|█▎ | 190/1400 [00:27<02:49, 7.14it/s]
end{sphinxVerbatim}
14%|█▎ | 190/1400 [00:27<02:49, 7.14it/s]
- more-to-come:
- class:
stderr
- 14%|█▎ | 191/1400 [00:27<02:45, 7.29it/s]
</pre>
- 14%|█▎ | 191/1400 [00:27<02:45, 7.29it/s]
end{sphinxVerbatim}
14%|█▎ | 191/1400 [00:27<02:45, 7.29it/s]
- more-to-come:
- class:
stderr
- 14%|█▎ | 192/1400 [00:27<02:52, 7.01it/s]
</pre>
- 14%|█▎ | 192/1400 [00:27<02:52, 7.01it/s]
end{sphinxVerbatim}
14%|█▎ | 192/1400 [00:27<02:52, 7.01it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 193/1400 [00:27<02:58, 6.78it/s]
</pre>
- 14%|█▍ | 193/1400 [00:27<02:58, 6.78it/s]
end{sphinxVerbatim}
14%|█▍ | 193/1400 [00:27<02:58, 6.78it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 194/1400 [00:27<02:48, 7.14it/s]
</pre>
- 14%|█▍ | 194/1400 [00:27<02:48, 7.14it/s]
end{sphinxVerbatim}
14%|█▍ | 194/1400 [00:27<02:48, 7.14it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 195/1400 [00:27<02:49, 7.11it/s]
</pre>
- 14%|█▍ | 195/1400 [00:27<02:49, 7.11it/s]
end{sphinxVerbatim}
14%|█▍ | 195/1400 [00:27<02:49, 7.11it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 196/1400 [00:27<02:44, 7.34it/s]
</pre>
- 14%|█▍ | 196/1400 [00:27<02:44, 7.34it/s]
end{sphinxVerbatim}
14%|█▍ | 196/1400 [00:27<02:44, 7.34it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 197/1400 [00:28<02:37, 7.62it/s]
</pre>
- 14%|█▍ | 197/1400 [00:28<02:37, 7.62it/s]
end{sphinxVerbatim}
14%|█▍ | 197/1400 [00:28<02:37, 7.62it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 198/1400 [00:28<02:31, 7.93it/s]
</pre>
- 14%|█▍ | 198/1400 [00:28<02:31, 7.93it/s]
end{sphinxVerbatim}
14%|█▍ | 198/1400 [00:28<02:31, 7.93it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 199/1400 [00:28<02:30, 8.00it/s]
</pre>
- 14%|█▍ | 199/1400 [00:28<02:30, 8.00it/s]
end{sphinxVerbatim}
14%|█▍ | 199/1400 [00:28<02:30, 8.00it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 200/1400 [00:28<02:43, 7.34it/s]
</pre>
- 14%|█▍ | 200/1400 [00:28<02:43, 7.34it/s]
end{sphinxVerbatim}
14%|█▍ | 200/1400 [00:28<02:43, 7.34it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 201/1400 [00:28<02:37, 7.62it/s]
</pre>
- 14%|█▍ | 201/1400 [00:28<02:37, 7.62it/s]
end{sphinxVerbatim}
14%|█▍ | 201/1400 [00:28<02:37, 7.62it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 202/1400 [00:28<02:36, 7.64it/s]
</pre>
- 14%|█▍ | 202/1400 [00:28<02:36, 7.64it/s]
end{sphinxVerbatim}
14%|█▍ | 202/1400 [00:28<02:36, 7.64it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 203/1400 [00:28<02:34, 7.74it/s]
</pre>
- 14%|█▍ | 203/1400 [00:28<02:34, 7.74it/s]
end{sphinxVerbatim}
14%|█▍ | 203/1400 [00:28<02:34, 7.74it/s]
- more-to-come:
- class:
stderr
- 15%|█▍ | 204/1400 [00:28<02:34, 7.75it/s]
</pre>
- 15%|█▍ | 204/1400 [00:28<02:34, 7.75it/s]
end{sphinxVerbatim}
15%|█▍ | 204/1400 [00:28<02:34, 7.75it/s]
- more-to-come:
- class:
stderr
- 15%|█▍ | 205/1400 [00:29<02:41, 7.39it/s]
</pre>
- 15%|█▍ | 205/1400 [00:29<02:41, 7.39it/s]
end{sphinxVerbatim}
15%|█▍ | 205/1400 [00:29<02:41, 7.39it/s]
- more-to-come:
- class:
stderr
- 15%|█▍ | 206/1400 [00:29<02:34, 7.74it/s]
</pre>
- 15%|█▍ | 206/1400 [00:29<02:34, 7.74it/s]
end{sphinxVerbatim}
15%|█▍ | 206/1400 [00:29<02:34, 7.74it/s]
- more-to-come:
- class:
stderr
- 15%|█▍ | 207/1400 [00:29<02:32, 7.81it/s]
</pre>
- 15%|█▍ | 207/1400 [00:29<02:32, 7.81it/s]
end{sphinxVerbatim}
15%|█▍ | 207/1400 [00:29<02:32, 7.81it/s]
- more-to-come:
- class:
stderr
- 15%|█▍ | 208/1400 [00:29<02:30, 7.90it/s]
</pre>
- 15%|█▍ | 208/1400 [00:29<02:30, 7.90it/s]
end{sphinxVerbatim}
15%|█▍ | 208/1400 [00:29<02:30, 7.90it/s]
- more-to-come:
- class:
stderr
- 15%|█▍ | 209/1400 [00:29<02:30, 7.94it/s]
</pre>
- 15%|█▍ | 209/1400 [00:29<02:30, 7.94it/s]
end{sphinxVerbatim}
15%|█▍ | 209/1400 [00:29<02:30, 7.94it/s]
- more-to-come:
- class:
stderr
- 15%|█▌ | 210/1400 [00:29<02:38, 7.51it/s]
</pre>
- 15%|█▌ | 210/1400 [00:29<02:38, 7.51it/s]
end{sphinxVerbatim}
15%|█▌ | 210/1400 [00:29<02:38, 7.51it/s]
- more-to-come:
- class:
stderr
- 15%|█▌ | 211/1400 [00:29<02:32, 7.81it/s]
</pre>
- 15%|█▌ | 211/1400 [00:29<02:32, 7.81it/s]
end{sphinxVerbatim}
15%|█▌ | 211/1400 [00:29<02:32, 7.81it/s]
- more-to-come:
- class:
stderr
- 15%|█▌ | 212/1400 [00:29<02:39, 7.44it/s]
</pre>
- 15%|█▌ | 212/1400 [00:29<02:39, 7.44it/s]
end{sphinxVerbatim}
15%|█▌ | 212/1400 [00:29<02:39, 7.44it/s]
- more-to-come:
- class:
stderr
- 15%|█▌ | 213/1400 [00:30<02:49, 7.00it/s]
</pre>
- 15%|█▌ | 213/1400 [00:30<02:49, 7.00it/s]
end{sphinxVerbatim}
15%|█▌ | 213/1400 [00:30<02:49, 7.00it/s]
- more-to-come:
- class:
stderr
- 15%|█▌ | 215/1400 [00:30<02:21, 8.40it/s]
</pre>
- 15%|█▌ | 215/1400 [00:30<02:21, 8.40it/s]
end{sphinxVerbatim}
15%|█▌ | 215/1400 [00:30<02:21, 8.40it/s]
- more-to-come:
- class:
stderr
- 15%|█▌ | 216/1400 [00:30<02:22, 8.28it/s]
</pre>
- 15%|█▌ | 216/1400 [00:30<02:22, 8.28it/s]
end{sphinxVerbatim}
15%|█▌ | 216/1400 [00:30<02:22, 8.28it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 217/1400 [00:30<02:25, 8.14it/s]
</pre>
- 16%|█▌ | 217/1400 [00:30<02:25, 8.14it/s]
end{sphinxVerbatim}
16%|█▌ | 217/1400 [00:30<02:25, 8.14it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 218/1400 [00:30<02:30, 7.87it/s]
</pre>
- 16%|█▌ | 218/1400 [00:30<02:30, 7.87it/s]
end{sphinxVerbatim}
16%|█▌ | 218/1400 [00:30<02:30, 7.87it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 219/1400 [00:30<02:27, 7.99it/s]
</pre>
- 16%|█▌ | 219/1400 [00:30<02:27, 7.99it/s]
end{sphinxVerbatim}
16%|█▌ | 219/1400 [00:30<02:27, 7.99it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 220/1400 [00:30<02:30, 7.84it/s]
</pre>
- 16%|█▌ | 220/1400 [00:30<02:30, 7.84it/s]
end{sphinxVerbatim}
16%|█▌ | 220/1400 [00:30<02:30, 7.84it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 221/1400 [00:31<02:28, 7.96it/s]
</pre>
- 16%|█▌ | 221/1400 [00:31<02:28, 7.96it/s]
end{sphinxVerbatim}
16%|█▌ | 221/1400 [00:31<02:28, 7.96it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 222/1400 [00:31<02:29, 7.87it/s]
</pre>
- 16%|█▌ | 222/1400 [00:31<02:29, 7.87it/s]
end{sphinxVerbatim}
16%|█▌ | 222/1400 [00:31<02:29, 7.87it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 223/1400 [00:31<02:26, 8.01it/s]
</pre>
- 16%|█▌ | 223/1400 [00:31<02:26, 8.01it/s]
end{sphinxVerbatim}
16%|█▌ | 223/1400 [00:31<02:26, 8.01it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 224/1400 [00:31<02:28, 7.91it/s]
</pre>
- 16%|█▌ | 224/1400 [00:31<02:28, 7.91it/s]
end{sphinxVerbatim}
16%|█▌ | 224/1400 [00:31<02:28, 7.91it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 225/1400 [00:31<02:34, 7.62it/s]
</pre>
- 16%|█▌ | 225/1400 [00:31<02:34, 7.62it/s]
end{sphinxVerbatim}
16%|█▌ | 225/1400 [00:31<02:34, 7.62it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 226/1400 [00:31<02:24, 8.10it/s]
</pre>
- 16%|█▌ | 226/1400 [00:31<02:24, 8.10it/s]
end{sphinxVerbatim}
16%|█▌ | 226/1400 [00:31<02:24, 8.10it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 227/1400 [00:31<02:23, 8.20it/s]
</pre>
- 16%|█▌ | 227/1400 [00:31<02:23, 8.20it/s]
end{sphinxVerbatim}
16%|█▌ | 227/1400 [00:31<02:23, 8.20it/s]
- more-to-come:
- class:
stderr
- 16%|█▋ | 228/1400 [00:31<02:25, 8.04it/s]
</pre>
- 16%|█▋ | 228/1400 [00:31<02:25, 8.04it/s]
end{sphinxVerbatim}
16%|█▋ | 228/1400 [00:31<02:25, 8.04it/s]
- more-to-come:
- class:
stderr
- 16%|█▋ | 229/1400 [00:32<02:23, 8.14it/s]
</pre>
- 16%|█▋ | 229/1400 [00:32<02:23, 8.14it/s]
end{sphinxVerbatim}
16%|█▋ | 229/1400 [00:32<02:23, 8.14it/s]
- more-to-come:
- class:
stderr
- 16%|█▋ | 230/1400 [00:32<02:34, 7.59it/s]
</pre>
- 16%|█▋ | 230/1400 [00:32<02:34, 7.59it/s]
end{sphinxVerbatim}
16%|█▋ | 230/1400 [00:32<02:34, 7.59it/s]
- more-to-come:
- class:
stderr
- 16%|█▋ | 231/1400 [00:32<02:42, 7.20it/s]
</pre>
- 16%|█▋ | 231/1400 [00:32<02:42, 7.20it/s]
end{sphinxVerbatim}
16%|█▋ | 231/1400 [00:32<02:42, 7.20it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 232/1400 [00:32<02:43, 7.14it/s]
</pre>
- 17%|█▋ | 232/1400 [00:32<02:43, 7.14it/s]
end{sphinxVerbatim}
17%|█▋ | 232/1400 [00:32<02:43, 7.14it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 233/1400 [00:32<02:42, 7.18it/s]
</pre>
- 17%|█▋ | 233/1400 [00:32<02:42, 7.18it/s]
end{sphinxVerbatim}
17%|█▋ | 233/1400 [00:32<02:42, 7.18it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 234/1400 [00:32<02:34, 7.54it/s]
</pre>
- 17%|█▋ | 234/1400 [00:32<02:34, 7.54it/s]
end{sphinxVerbatim}
17%|█▋ | 234/1400 [00:32<02:34, 7.54it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 235/1400 [00:32<02:41, 7.23it/s]
</pre>
- 17%|█▋ | 235/1400 [00:32<02:41, 7.23it/s]
end{sphinxVerbatim}
17%|█▋ | 235/1400 [00:32<02:41, 7.23it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 236/1400 [00:33<02:32, 7.62it/s]
</pre>
- 17%|█▋ | 236/1400 [00:33<02:32, 7.62it/s]
end{sphinxVerbatim}
17%|█▋ | 236/1400 [00:33<02:32, 7.62it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 237/1400 [00:33<02:27, 7.91it/s]
</pre>
- 17%|█▋ | 237/1400 [00:33<02:27, 7.91it/s]
end{sphinxVerbatim}
17%|█▋ | 237/1400 [00:33<02:27, 7.91it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 238/1400 [00:33<02:29, 7.76it/s]
</pre>
- 17%|█▋ | 238/1400 [00:33<02:29, 7.76it/s]
end{sphinxVerbatim}
17%|█▋ | 238/1400 [00:33<02:29, 7.76it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 239/1400 [00:33<02:27, 7.87it/s]
</pre>
- 17%|█▋ | 239/1400 [00:33<02:27, 7.87it/s]
end{sphinxVerbatim}
17%|█▋ | 239/1400 [00:33<02:27, 7.87it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 240/1400 [00:33<02:27, 7.88it/s]
</pre>
- 17%|█▋ | 240/1400 [00:33<02:27, 7.88it/s]
end{sphinxVerbatim}
17%|█▋ | 240/1400 [00:33<02:27, 7.88it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 241/1400 [00:33<02:23, 8.09it/s]
</pre>
- 17%|█▋ | 241/1400 [00:33<02:23, 8.09it/s]
end{sphinxVerbatim}
17%|█▋ | 241/1400 [00:33<02:23, 8.09it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 242/1400 [00:33<02:24, 8.03it/s]
</pre>
- 17%|█▋ | 242/1400 [00:33<02:24, 8.03it/s]
end{sphinxVerbatim}
17%|█▋ | 242/1400 [00:33<02:24, 8.03it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 243/1400 [00:33<02:17, 8.40it/s]
</pre>
- 17%|█▋ | 243/1400 [00:33<02:17, 8.40it/s]
end{sphinxVerbatim}
17%|█▋ | 243/1400 [00:33<02:17, 8.40it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 244/1400 [00:34<02:17, 8.40it/s]
</pre>
- 17%|█▋ | 244/1400 [00:34<02:17, 8.40it/s]
end{sphinxVerbatim}
17%|█▋ | 244/1400 [00:34<02:17, 8.40it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 245/1400 [00:34<02:21, 8.18it/s]
</pre>
- 18%|█▊ | 245/1400 [00:34<02:21, 8.18it/s]
end{sphinxVerbatim}
18%|█▊ | 245/1400 [00:34<02:21, 8.18it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 246/1400 [00:34<02:20, 8.22it/s]
</pre>
- 18%|█▊ | 246/1400 [00:34<02:20, 8.22it/s]
end{sphinxVerbatim}
18%|█▊ | 246/1400 [00:34<02:20, 8.22it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 247/1400 [00:34<02:20, 8.23it/s]
</pre>
- 18%|█▊ | 247/1400 [00:34<02:20, 8.23it/s]
end{sphinxVerbatim}
18%|█▊ | 247/1400 [00:34<02:20, 8.23it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 248/1400 [00:34<02:18, 8.32it/s]
</pre>
- 18%|█▊ | 248/1400 [00:34<02:18, 8.32it/s]
end{sphinxVerbatim}
18%|█▊ | 248/1400 [00:34<02:18, 8.32it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 249/1400 [00:34<02:17, 8.37it/s]
</pre>
- 18%|█▊ | 249/1400 [00:34<02:17, 8.37it/s]
end{sphinxVerbatim}
18%|█▊ | 249/1400 [00:34<02:17, 8.37it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 250/1400 [00:34<02:23, 7.99it/s]
</pre>
- 18%|█▊ | 250/1400 [00:34<02:23, 7.99it/s]
end{sphinxVerbatim}
18%|█▊ | 250/1400 [00:34<02:23, 7.99it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 251/1400 [00:34<02:39, 7.22it/s]
</pre>
- 18%|█▊ | 251/1400 [00:34<02:39, 7.22it/s]
end{sphinxVerbatim}
18%|█▊ | 251/1400 [00:34<02:39, 7.22it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 252/1400 [00:35<02:39, 7.21it/s]
</pre>
- 18%|█▊ | 252/1400 [00:35<02:39, 7.21it/s]
end{sphinxVerbatim}
18%|█▊ | 252/1400 [00:35<02:39, 7.21it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 253/1400 [00:35<02:33, 7.48it/s]
</pre>
- 18%|█▊ | 253/1400 [00:35<02:33, 7.48it/s]
end{sphinxVerbatim}
18%|█▊ | 253/1400 [00:35<02:33, 7.48it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 255/1400 [00:35<02:24, 7.95it/s]
</pre>
- 18%|█▊ | 255/1400 [00:35<02:24, 7.95it/s]
end{sphinxVerbatim}
18%|█▊ | 255/1400 [00:35<02:24, 7.95it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 256/1400 [00:35<02:24, 7.92it/s]
</pre>
- 18%|█▊ | 256/1400 [00:35<02:24, 7.92it/s]
end{sphinxVerbatim}
18%|█▊ | 256/1400 [00:35<02:24, 7.92it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 257/1400 [00:35<02:22, 8.04it/s]
</pre>
- 18%|█▊ | 257/1400 [00:35<02:22, 8.04it/s]
end{sphinxVerbatim}
18%|█▊ | 257/1400 [00:35<02:22, 8.04it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 258/1400 [00:35<02:23, 7.98it/s]
</pre>
- 18%|█▊ | 258/1400 [00:35<02:23, 7.98it/s]
end{sphinxVerbatim}
18%|█▊ | 258/1400 [00:35<02:23, 7.98it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 259/1400 [00:35<02:23, 7.95it/s]
</pre>
- 18%|█▊ | 259/1400 [00:35<02:23, 7.95it/s]
end{sphinxVerbatim}
18%|█▊ | 259/1400 [00:35<02:23, 7.95it/s]
- more-to-come:
- class:
stderr
- 19%|█▊ | 260/1400 [00:36<02:24, 7.88it/s]
</pre>
- 19%|█▊ | 260/1400 [00:36<02:24, 7.88it/s]
end{sphinxVerbatim}
19%|█▊ | 260/1400 [00:36<02:24, 7.88it/s]
- more-to-come:
- class:
stderr
- 19%|█▊ | 261/1400 [00:36<02:18, 8.23it/s]
</pre>
- 19%|█▊ | 261/1400 [00:36<02:18, 8.23it/s]
end{sphinxVerbatim}
19%|█▊ | 261/1400 [00:36<02:18, 8.23it/s]
- more-to-come:
- class:
stderr
- 19%|█▊ | 262/1400 [00:36<02:16, 8.34it/s]
</pre>
- 19%|█▊ | 262/1400 [00:36<02:16, 8.34it/s]
end{sphinxVerbatim}
19%|█▊ | 262/1400 [00:36<02:16, 8.34it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 263/1400 [00:36<02:16, 8.32it/s]
</pre>
- 19%|█▉ | 263/1400 [00:36<02:16, 8.32it/s]
end{sphinxVerbatim}
19%|█▉ | 263/1400 [00:36<02:16, 8.32it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 264/1400 [00:36<02:10, 8.70it/s]
</pre>
- 19%|█▉ | 264/1400 [00:36<02:10, 8.70it/s]
end{sphinxVerbatim}
19%|█▉ | 264/1400 [00:36<02:10, 8.70it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 265/1400 [00:36<02:13, 8.52it/s]
</pre>
- 19%|█▉ | 265/1400 [00:36<02:13, 8.52it/s]
end{sphinxVerbatim}
19%|█▉ | 265/1400 [00:36<02:13, 8.52it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 266/1400 [00:36<02:13, 8.50it/s]
</pre>
- 19%|█▉ | 266/1400 [00:36<02:13, 8.50it/s]
end{sphinxVerbatim}
19%|█▉ | 266/1400 [00:36<02:13, 8.50it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 267/1400 [00:36<02:10, 8.65it/s]
</pre>
- 19%|█▉ | 267/1400 [00:36<02:10, 8.65it/s]
end{sphinxVerbatim}
19%|█▉ | 267/1400 [00:36<02:10, 8.65it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 268/1400 [00:36<02:12, 8.56it/s]
</pre>
- 19%|█▉ | 268/1400 [00:36<02:12, 8.56it/s]
end{sphinxVerbatim}
19%|█▉ | 268/1400 [00:36<02:12, 8.56it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 269/1400 [00:37<02:17, 8.20it/s]
</pre>
- 19%|█▉ | 269/1400 [00:37<02:17, 8.20it/s]
end{sphinxVerbatim}
19%|█▉ | 269/1400 [00:37<02:17, 8.20it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 270/1400 [00:37<02:24, 7.82it/s]
</pre>
- 19%|█▉ | 270/1400 [00:37<02:24, 7.82it/s]
end{sphinxVerbatim}
19%|█▉ | 270/1400 [00:37<02:24, 7.82it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 271/1400 [00:37<02:23, 7.84it/s]
</pre>
- 19%|█▉ | 271/1400 [00:37<02:23, 7.84it/s]
end{sphinxVerbatim}
19%|█▉ | 271/1400 [00:37<02:23, 7.84it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 272/1400 [00:37<02:16, 8.27it/s]
</pre>
- 19%|█▉ | 272/1400 [00:37<02:16, 8.27it/s]
end{sphinxVerbatim}
19%|█▉ | 272/1400 [00:37<02:16, 8.27it/s]
- more-to-come:
- class:
stderr
- 20%|█▉ | 273/1400 [00:37<02:19, 8.07it/s]
</pre>
- 20%|█▉ | 273/1400 [00:37<02:19, 8.07it/s]
end{sphinxVerbatim}
20%|█▉ | 273/1400 [00:37<02:19, 8.07it/s]
- more-to-come:
- class:
stderr
- 20%|█▉ | 274/1400 [00:37<02:21, 7.95it/s]
</pre>
- 20%|█▉ | 274/1400 [00:37<02:21, 7.95it/s]
end{sphinxVerbatim}
20%|█▉ | 274/1400 [00:37<02:21, 7.95it/s]
- more-to-come:
- class:
stderr
- 20%|█▉ | 275/1400 [00:37<02:21, 7.97it/s]
</pre>
- 20%|█▉ | 275/1400 [00:37<02:21, 7.97it/s]
end{sphinxVerbatim}
20%|█▉ | 275/1400 [00:37<02:21, 7.97it/s]
- more-to-come:
- class:
stderr
- 20%|█▉ | 276/1400 [00:37<02:15, 8.32it/s]
</pre>
- 20%|█▉ | 276/1400 [00:37<02:15, 8.32it/s]
end{sphinxVerbatim}
20%|█▉ | 276/1400 [00:37<02:15, 8.32it/s]
- more-to-come:
- class:
stderr
- 20%|█▉ | 278/1400 [00:38<02:08, 8.76it/s]
</pre>
- 20%|█▉ | 278/1400 [00:38<02:08, 8.76it/s]
end{sphinxVerbatim}
20%|█▉ | 278/1400 [00:38<02:08, 8.76it/s]
- more-to-come:
- class:
stderr
- 20%|█▉ | 279/1400 [00:38<02:04, 8.99it/s]
</pre>
- 20%|█▉ | 279/1400 [00:38<02:04, 8.99it/s]
end{sphinxVerbatim}
20%|█▉ | 279/1400 [00:38<02:04, 8.99it/s]
- more-to-come:
- class:
stderr
- 20%|██ | 280/1400 [00:38<02:10, 8.56it/s]
</pre>
- 20%|██ | 280/1400 [00:38<02:10, 8.56it/s]
end{sphinxVerbatim}
20%|██ | 280/1400 [00:38<02:10, 8.56it/s]
- more-to-come:
- class:
stderr
- 20%|██ | 282/1400 [00:38<01:51, 9.99it/s]
</pre>
- 20%|██ | 282/1400 [00:38<01:51, 9.99it/s]
end{sphinxVerbatim}
20%|██ | 282/1400 [00:38<01:51, 9.99it/s]
- more-to-come:
- class:
stderr
- 20%|██ | 283/1400 [00:38<01:53, 9.82it/s]
</pre>
- 20%|██ | 283/1400 [00:38<01:53, 9.82it/s]
end{sphinxVerbatim}
20%|██ | 283/1400 [00:38<01:53, 9.82it/s]
- more-to-come:
- class:
stderr
- 20%|██ | 285/1400 [00:38<02:00, 9.21it/s]
</pre>
- 20%|██ | 285/1400 [00:38<02:00, 9.21it/s]
end{sphinxVerbatim}
20%|██ | 285/1400 [00:38<02:00, 9.21it/s]
- more-to-come:
- class:
stderr
- 20%|██ | 286/1400 [00:39<01:59, 9.30it/s]
</pre>
- 20%|██ | 286/1400 [00:39<01:59, 9.30it/s]
end{sphinxVerbatim}
20%|██ | 286/1400 [00:39<01:59, 9.30it/s]
- more-to-come:
- class:
stderr
- 20%|██ | 287/1400 [00:39<01:59, 9.34it/s]
</pre>
- 20%|██ | 287/1400 [00:39<01:59, 9.34it/s]
end{sphinxVerbatim}
20%|██ | 287/1400 [00:39<01:59, 9.34it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 288/1400 [00:39<02:03, 9.04it/s]
</pre>
- 21%|██ | 288/1400 [00:39<02:03, 9.04it/s]
end{sphinxVerbatim}
21%|██ | 288/1400 [00:39<02:03, 9.04it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 289/1400 [00:39<02:00, 9.24it/s]
</pre>
- 21%|██ | 289/1400 [00:39<02:00, 9.24it/s]
end{sphinxVerbatim}
21%|██ | 289/1400 [00:39<02:00, 9.24it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 290/1400 [00:39<02:08, 8.62it/s]
</pre>
- 21%|██ | 290/1400 [00:39<02:08, 8.62it/s]
end{sphinxVerbatim}
21%|██ | 290/1400 [00:39<02:08, 8.62it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 291/1400 [00:39<02:05, 8.85it/s]
</pre>
- 21%|██ | 291/1400 [00:39<02:05, 8.85it/s]
end{sphinxVerbatim}
21%|██ | 291/1400 [00:39<02:05, 8.85it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 292/1400 [00:39<02:06, 8.73it/s]
</pre>
- 21%|██ | 292/1400 [00:39<02:06, 8.73it/s]
end{sphinxVerbatim}
21%|██ | 292/1400 [00:39<02:06, 8.73it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 293/1400 [00:39<02:04, 8.88it/s]
</pre>
- 21%|██ | 293/1400 [00:39<02:04, 8.88it/s]
end{sphinxVerbatim}
21%|██ | 293/1400 [00:39<02:04, 8.88it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 294/1400 [00:39<02:06, 8.76it/s]
</pre>
- 21%|██ | 294/1400 [00:39<02:06, 8.76it/s]
end{sphinxVerbatim}
21%|██ | 294/1400 [00:39<02:06, 8.76it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 295/1400 [00:40<02:08, 8.57it/s]
</pre>
- 21%|██ | 295/1400 [00:40<02:08, 8.57it/s]
end{sphinxVerbatim}
21%|██ | 295/1400 [00:40<02:08, 8.57it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 296/1400 [00:40<02:09, 8.53it/s]
</pre>
- 21%|██ | 296/1400 [00:40<02:09, 8.53it/s]
end{sphinxVerbatim}
21%|██ | 296/1400 [00:40<02:09, 8.53it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 297/1400 [00:40<02:11, 8.41it/s]
</pre>
- 21%|██ | 297/1400 [00:40<02:11, 8.41it/s]
end{sphinxVerbatim}
21%|██ | 297/1400 [00:40<02:11, 8.41it/s]
- more-to-come:
- class:
stderr
- 21%|██▏ | 298/1400 [00:40<02:10, 8.45it/s]
</pre>
- 21%|██▏ | 298/1400 [00:40<02:10, 8.45it/s]
end{sphinxVerbatim}
21%|██▏ | 298/1400 [00:40<02:10, 8.45it/s]
- more-to-come:
- class:
stderr
- 21%|██▏ | 299/1400 [00:40<02:08, 8.58it/s]
</pre>
- 21%|██▏ | 299/1400 [00:40<02:08, 8.58it/s]
end{sphinxVerbatim}
21%|██▏ | 299/1400 [00:40<02:08, 8.58it/s]
- more-to-come:
- class:
stderr
- 21%|██▏ | 300/1400 [00:40<02:13, 8.25it/s]
</pre>
- 21%|██▏ | 300/1400 [00:40<02:13, 8.25it/s]
end{sphinxVerbatim}
21%|██▏ | 300/1400 [00:40<02:13, 8.25it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 301/1400 [00:40<02:10, 8.42it/s]
</pre>
- 22%|██▏ | 301/1400 [00:40<02:10, 8.42it/s]
end{sphinxVerbatim}
22%|██▏ | 301/1400 [00:40<02:10, 8.42it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 303/1400 [00:41<02:07, 8.63it/s]
</pre>
- 22%|██▏ | 303/1400 [00:41<02:07, 8.63it/s]
end{sphinxVerbatim}
22%|██▏ | 303/1400 [00:41<02:07, 8.63it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 304/1400 [00:41<02:11, 8.36it/s]
</pre>
- 22%|██▏ | 304/1400 [00:41<02:11, 8.36it/s]
end{sphinxVerbatim}
22%|██▏ | 304/1400 [00:41<02:11, 8.36it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 305/1400 [00:41<02:13, 8.19it/s]
</pre>
- 22%|██▏ | 305/1400 [00:41<02:13, 8.19it/s]
end{sphinxVerbatim}
22%|██▏ | 305/1400 [00:41<02:13, 8.19it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 306/1400 [00:41<02:07, 8.58it/s]
</pre>
- 22%|██▏ | 306/1400 [00:41<02:07, 8.58it/s]
end{sphinxVerbatim}
22%|██▏ | 306/1400 [00:41<02:07, 8.58it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 308/1400 [00:41<02:04, 8.80it/s]
</pre>
- 22%|██▏ | 308/1400 [00:41<02:04, 8.80it/s]
end{sphinxVerbatim}
22%|██▏ | 308/1400 [00:41<02:04, 8.80it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 309/1400 [00:41<02:02, 8.92it/s]
</pre>
- 22%|██▏ | 309/1400 [00:41<02:02, 8.92it/s]
end{sphinxVerbatim}
22%|██▏ | 309/1400 [00:41<02:02, 8.92it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 310/1400 [00:41<02:09, 8.45it/s]
</pre>
- 22%|██▏ | 310/1400 [00:41<02:09, 8.45it/s]
end{sphinxVerbatim}
22%|██▏ | 310/1400 [00:41<02:09, 8.45it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 312/1400 [00:41<01:47, 10.16it/s]
</pre>
- 22%|██▏ | 312/1400 [00:41<01:47, 10.16it/s]
end{sphinxVerbatim}
22%|██▏ | 312/1400 [00:41<01:47, 10.16it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 314/1400 [00:42<01:54, 9.50it/s]
</pre>
- 22%|██▏ | 314/1400 [00:42<01:54, 9.50it/s]
end{sphinxVerbatim}
22%|██▏ | 314/1400 [00:42<01:54, 9.50it/s]
- more-to-come:
- class:
stderr
- 22%|██▎ | 315/1400 [00:42<01:58, 9.19it/s]
</pre>
- 22%|██▎ | 315/1400 [00:42<01:58, 9.19it/s]
end{sphinxVerbatim}
22%|██▎ | 315/1400 [00:42<01:58, 9.19it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 316/1400 [00:42<02:02, 8.88it/s]
</pre>
- 23%|██▎ | 316/1400 [00:42<02:02, 8.88it/s]
end{sphinxVerbatim}
23%|██▎ | 316/1400 [00:42<02:02, 8.88it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 317/1400 [00:42<01:59, 9.04it/s]
</pre>
- 23%|██▎ | 317/1400 [00:42<01:59, 9.04it/s]
end{sphinxVerbatim}
23%|██▎ | 317/1400 [00:42<01:59, 9.04it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 318/1400 [00:42<02:00, 9.01it/s]
</pre>
- 23%|██▎ | 318/1400 [00:42<02:00, 9.01it/s]
end{sphinxVerbatim}
23%|██▎ | 318/1400 [00:42<02:00, 9.01it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 319/1400 [00:42<02:01, 8.92it/s]
</pre>
- 23%|██▎ | 319/1400 [00:42<02:01, 8.92it/s]
end{sphinxVerbatim}
23%|██▎ | 319/1400 [00:42<02:01, 8.92it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 320/1400 [00:42<02:03, 8.73it/s]
</pre>
- 23%|██▎ | 320/1400 [00:42<02:03, 8.73it/s]
end{sphinxVerbatim}
23%|██▎ | 320/1400 [00:42<02:03, 8.73it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 321/1400 [00:43<01:59, 9.02it/s]
</pre>
- 23%|██▎ | 321/1400 [00:43<01:59, 9.02it/s]
end{sphinxVerbatim}
23%|██▎ | 321/1400 [00:43<01:59, 9.02it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 322/1400 [00:43<01:57, 9.18it/s]
</pre>
- 23%|██▎ | 322/1400 [00:43<01:57, 9.18it/s]
end{sphinxVerbatim}
23%|██▎ | 322/1400 [00:43<01:57, 9.18it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 323/1400 [00:43<02:01, 8.86it/s]
</pre>
- 23%|██▎ | 323/1400 [00:43<02:01, 8.86it/s]
end{sphinxVerbatim}
23%|██▎ | 323/1400 [00:43<02:01, 8.86it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 324/1400 [00:43<01:57, 9.13it/s]
</pre>
- 23%|██▎ | 324/1400 [00:43<01:57, 9.13it/s]
end{sphinxVerbatim}
23%|██▎ | 324/1400 [00:43<01:57, 9.13it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 325/1400 [00:43<02:05, 8.57it/s]
</pre>
- 23%|██▎ | 325/1400 [00:43<02:05, 8.57it/s]
end{sphinxVerbatim}
23%|██▎ | 325/1400 [00:43<02:05, 8.57it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 326/1400 [00:43<02:07, 8.41it/s]
</pre>
- 23%|██▎ | 326/1400 [00:43<02:07, 8.41it/s]
end{sphinxVerbatim}
23%|██▎ | 326/1400 [00:43<02:07, 8.41it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 327/1400 [00:43<02:10, 8.23it/s]
</pre>
- 23%|██▎ | 327/1400 [00:43<02:10, 8.23it/s]
end{sphinxVerbatim}
23%|██▎ | 327/1400 [00:43<02:10, 8.23it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 328/1400 [00:43<02:11, 8.15it/s]
</pre>
- 23%|██▎ | 328/1400 [00:43<02:11, 8.15it/s]
end{sphinxVerbatim}
23%|██▎ | 328/1400 [00:43<02:11, 8.15it/s]
- more-to-come:
- class:
stderr
- 24%|██▎ | 330/1400 [00:44<02:05, 8.55it/s]
</pre>
- 24%|██▎ | 330/1400 [00:44<02:05, 8.55it/s]
end{sphinxVerbatim}
24%|██▎ | 330/1400 [00:44<02:05, 8.55it/s]
- more-to-come:
- class:
stderr
- 24%|██▎ | 331/1400 [00:44<02:00, 8.84it/s]
</pre>
- 24%|██▎ | 331/1400 [00:44<02:00, 8.84it/s]
end{sphinxVerbatim}
24%|██▎ | 331/1400 [00:44<02:00, 8.84it/s]
- more-to-come:
- class:
stderr
- 24%|██▎ | 332/1400 [00:44<01:58, 9.04it/s]
</pre>
- 24%|██▎ | 332/1400 [00:44<01:58, 9.04it/s]
end{sphinxVerbatim}
24%|██▎ | 332/1400 [00:44<01:58, 9.04it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 333/1400 [00:44<01:58, 8.97it/s]
</pre>
- 24%|██▍ | 333/1400 [00:44<01:58, 8.97it/s]
end{sphinxVerbatim}
24%|██▍ | 333/1400 [00:44<01:58, 8.97it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 334/1400 [00:44<02:07, 8.35it/s]
</pre>
- 24%|██▍ | 334/1400 [00:44<02:07, 8.35it/s]
end{sphinxVerbatim}
24%|██▍ | 334/1400 [00:44<02:07, 8.35it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 335/1400 [00:44<02:12, 8.06it/s]
</pre>
- 24%|██▍ | 335/1400 [00:44<02:12, 8.06it/s]
end{sphinxVerbatim}
24%|██▍ | 335/1400 [00:44<02:12, 8.06it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 336/1400 [00:44<02:11, 8.08it/s]
</pre>
- 24%|██▍ | 336/1400 [00:44<02:11, 8.08it/s]
end{sphinxVerbatim}
24%|██▍ | 336/1400 [00:44<02:11, 8.08it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 338/1400 [00:44<02:00, 8.83it/s]
</pre>
- 24%|██▍ | 338/1400 [00:44<02:00, 8.83it/s]
end{sphinxVerbatim}
24%|██▍ | 338/1400 [00:44<02:00, 8.83it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 340/1400 [00:45<02:00, 8.79it/s]
</pre>
- 24%|██▍ | 340/1400 [00:45<02:00, 8.79it/s]
end{sphinxVerbatim}
24%|██▍ | 340/1400 [00:45<02:00, 8.79it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 341/1400 [00:45<01:57, 9.00it/s]
</pre>
- 24%|██▍ | 341/1400 [00:45<01:57, 9.00it/s]
end{sphinxVerbatim}
24%|██▍ | 341/1400 [00:45<01:57, 9.00it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 342/1400 [00:45<01:56, 9.08it/s]
</pre>
- 24%|██▍ | 342/1400 [00:45<01:56, 9.08it/s]
end{sphinxVerbatim}
24%|██▍ | 342/1400 [00:45<01:56, 9.08it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 343/1400 [00:45<01:54, 9.27it/s]
</pre>
- 24%|██▍ | 343/1400 [00:45<01:54, 9.27it/s]
end{sphinxVerbatim}
24%|██▍ | 343/1400 [00:45<01:54, 9.27it/s]
- more-to-come:
- class:
stderr
- 25%|██▍ | 345/1400 [00:45<01:54, 9.23it/s]
</pre>
- 25%|██▍ | 345/1400 [00:45<01:54, 9.23it/s]
end{sphinxVerbatim}
25%|██▍ | 345/1400 [00:45<01:54, 9.23it/s]
- more-to-come:
- class:
stderr
- 25%|██▍ | 346/1400 [00:45<02:00, 8.76it/s]
</pre>
- 25%|██▍ | 346/1400 [00:45<02:00, 8.76it/s]
end{sphinxVerbatim}
25%|██▍ | 346/1400 [00:45<02:00, 8.76it/s]
- more-to-come:
- class:
stderr
- 25%|██▍ | 347/1400 [00:46<02:02, 8.61it/s]
</pre>
- 25%|██▍ | 347/1400 [00:46<02:02, 8.61it/s]
end{sphinxVerbatim}
25%|██▍ | 347/1400 [00:46<02:02, 8.61it/s]
- more-to-come:
- class:
stderr
- 25%|██▍ | 348/1400 [00:46<02:05, 8.41it/s]
</pre>
- 25%|██▍ | 348/1400 [00:46<02:05, 8.41it/s]
end{sphinxVerbatim}
25%|██▍ | 348/1400 [00:46<02:05, 8.41it/s]
- more-to-come:
- class:
stderr
- 25%|██▍ | 349/1400 [00:46<02:00, 8.75it/s]
</pre>
- 25%|██▍ | 349/1400 [00:46<02:00, 8.75it/s]
end{sphinxVerbatim}
25%|██▍ | 349/1400 [00:46<02:00, 8.75it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 350/1400 [00:46<02:02, 8.59it/s]
</pre>
- 25%|██▌ | 350/1400 [00:46<02:02, 8.59it/s]
end{sphinxVerbatim}
25%|██▌ | 350/1400 [00:46<02:02, 8.59it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 351/1400 [00:46<01:58, 8.88it/s]
</pre>
- 25%|██▌ | 351/1400 [00:46<01:58, 8.88it/s]
end{sphinxVerbatim}
25%|██▌ | 351/1400 [00:46<01:58, 8.88it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 352/1400 [00:46<01:54, 9.13it/s]
</pre>
- 25%|██▌ | 352/1400 [00:46<01:54, 9.13it/s]
end{sphinxVerbatim}
25%|██▌ | 352/1400 [00:46<01:54, 9.13it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 353/1400 [00:46<01:55, 9.09it/s]
</pre>
- 25%|██▌ | 353/1400 [00:46<01:55, 9.09it/s]
end{sphinxVerbatim}
25%|██▌ | 353/1400 [00:46<01:55, 9.09it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 354/1400 [00:46<02:03, 8.45it/s]
</pre>
- 25%|██▌ | 354/1400 [00:46<02:03, 8.45it/s]
end{sphinxVerbatim}
25%|██▌ | 354/1400 [00:46<02:03, 8.45it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 355/1400 [00:46<02:07, 8.21it/s]
</pre>
- 25%|██▌ | 355/1400 [00:46<02:07, 8.21it/s]
end{sphinxVerbatim}
25%|██▌ | 355/1400 [00:46<02:07, 8.21it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 356/1400 [00:47<02:02, 8.53it/s]
</pre>
- 25%|██▌ | 356/1400 [00:47<02:02, 8.53it/s]
end{sphinxVerbatim}
25%|██▌ | 356/1400 [00:47<02:02, 8.53it/s]
- more-to-come:
- class:
stderr
- 26%|██▌ | 358/1400 [00:47<01:38, 10.61it/s]
</pre>
- 26%|██▌ | 358/1400 [00:47<01:38, 10.61it/s]
end{sphinxVerbatim}
26%|██▌ | 358/1400 [00:47<01:38, 10.61it/s]
- more-to-come:
- class:
stderr
- 26%|██▌ | 360/1400 [00:47<01:45, 9.81it/s]
</pre>
- 26%|██▌ | 360/1400 [00:47<01:45, 9.81it/s]
end{sphinxVerbatim}
26%|██▌ | 360/1400 [00:47<01:45, 9.81it/s]
- more-to-come:
- class:
stderr
- 26%|██▌ | 361/1400 [00:47<01:46, 9.78it/s]
</pre>
- 26%|██▌ | 361/1400 [00:47<01:46, 9.78it/s]
end{sphinxVerbatim}
26%|██▌ | 361/1400 [00:47<01:46, 9.78it/s]
- more-to-come:
- class:
stderr
- 26%|██▌ | 363/1400 [00:47<01:32, 11.24it/s]
</pre>
- 26%|██▌ | 363/1400 [00:47<01:32, 11.24it/s]
end{sphinxVerbatim}
26%|██▌ | 363/1400 [00:47<01:32, 11.24it/s]
- more-to-come:
- class:
stderr
- 26%|██▌ | 365/1400 [00:47<01:44, 9.94it/s]
</pre>
- 26%|██▌ | 365/1400 [00:47<01:44, 9.94it/s]
end{sphinxVerbatim}
26%|██▌ | 365/1400 [00:47<01:44, 9.94it/s]
- more-to-come:
- class:
stderr
- 26%|██▌ | 367/1400 [00:48<01:43, 9.98it/s]
</pre>
- 26%|██▌ | 367/1400 [00:48<01:43, 9.98it/s]
end{sphinxVerbatim}
26%|██▌ | 367/1400 [00:48<01:43, 9.98it/s]
- more-to-come:
- class:
stderr
- 26%|██▋ | 369/1400 [00:48<01:42, 10.09it/s]
</pre>
- 26%|██▋ | 369/1400 [00:48<01:42, 10.09it/s]
end{sphinxVerbatim}
26%|██▋ | 369/1400 [00:48<01:42, 10.09it/s]
- more-to-come:
- class:
stderr
- 26%|██▋ | 371/1400 [00:48<01:46, 9.70it/s]
</pre>
- 26%|██▋ | 371/1400 [00:48<01:46, 9.70it/s]
end{sphinxVerbatim}
26%|██▋ | 371/1400 [00:48<01:46, 9.70it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 372/1400 [00:48<01:45, 9.75it/s]
</pre>
- 27%|██▋ | 372/1400 [00:48<01:45, 9.75it/s]
end{sphinxVerbatim}
27%|██▋ | 372/1400 [00:48<01:45, 9.75it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 373/1400 [00:48<01:47, 9.57it/s]
</pre>
- 27%|██▋ | 373/1400 [00:48<01:47, 9.57it/s]
end{sphinxVerbatim}
27%|██▋ | 373/1400 [00:48<01:47, 9.57it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 374/1400 [00:48<01:53, 9.04it/s]
</pre>
- 27%|██▋ | 374/1400 [00:48<01:53, 9.04it/s]
end{sphinxVerbatim}
27%|██▋ | 374/1400 [00:48<01:53, 9.04it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 375/1400 [00:48<02:01, 8.44it/s]
</pre>
- 27%|██▋ | 375/1400 [00:48<02:01, 8.44it/s]
end{sphinxVerbatim}
27%|██▋ | 375/1400 [00:48<02:01, 8.44it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 376/1400 [00:49<02:05, 8.16it/s]
</pre>
- 27%|██▋ | 376/1400 [00:49<02:05, 8.16it/s]
end{sphinxVerbatim}
27%|██▋ | 376/1400 [00:49<02:05, 8.16it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 377/1400 [00:49<02:06, 8.10it/s]
</pre>
- 27%|██▋ | 377/1400 [00:49<02:06, 8.10it/s]
end{sphinxVerbatim}
27%|██▋ | 377/1400 [00:49<02:06, 8.10it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 378/1400 [00:49<02:07, 7.99it/s]
</pre>
- 27%|██▋ | 378/1400 [00:49<02:07, 7.99it/s]
end{sphinxVerbatim}
27%|██▋ | 378/1400 [00:49<02:07, 7.99it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 379/1400 [00:49<02:03, 8.30it/s]
</pre>
- 27%|██▋ | 379/1400 [00:49<02:03, 8.30it/s]
end{sphinxVerbatim}
27%|██▋ | 379/1400 [00:49<02:03, 8.30it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 380/1400 [00:49<02:03, 8.27it/s]
</pre>
- 27%|██▋ | 380/1400 [00:49<02:03, 8.27it/s]
end{sphinxVerbatim}
27%|██▋ | 380/1400 [00:49<02:03, 8.27it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 381/1400 [00:49<02:01, 8.36it/s]
</pre>
- 27%|██▋ | 381/1400 [00:49<02:01, 8.36it/s]
end{sphinxVerbatim}
27%|██▋ | 381/1400 [00:49<02:01, 8.36it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 382/1400 [00:49<01:56, 8.74it/s]
</pre>
- 27%|██▋ | 382/1400 [00:49<01:56, 8.74it/s]
end{sphinxVerbatim}
27%|██▋ | 382/1400 [00:49<01:56, 8.74it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 383/1400 [00:49<01:57, 8.63it/s]
</pre>
- 27%|██▋ | 383/1400 [00:49<01:57, 8.63it/s]
end{sphinxVerbatim}
27%|██▋ | 383/1400 [00:49<01:57, 8.63it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 384/1400 [00:50<01:53, 8.96it/s]
</pre>
- 27%|██▋ | 384/1400 [00:50<01:53, 8.96it/s]
end{sphinxVerbatim}
27%|██▋ | 384/1400 [00:50<01:53, 8.96it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 385/1400 [00:50<01:56, 8.74it/s]
</pre>
- 28%|██▊ | 385/1400 [00:50<01:56, 8.74it/s]
end{sphinxVerbatim}
28%|██▊ | 385/1400 [00:50<01:56, 8.74it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 386/1400 [00:50<01:52, 9.00it/s]
</pre>
- 28%|██▊ | 386/1400 [00:50<01:52, 9.00it/s]
end{sphinxVerbatim}
28%|██▊ | 386/1400 [00:50<01:52, 9.00it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 388/1400 [00:50<01:48, 9.35it/s]
</pre>
- 28%|██▊ | 388/1400 [00:50<01:48, 9.35it/s]
end{sphinxVerbatim}
28%|██▊ | 388/1400 [00:50<01:48, 9.35it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 390/1400 [00:50<01:48, 9.29it/s]
</pre>
- 28%|██▊ | 390/1400 [00:50<01:48, 9.29it/s]
end{sphinxVerbatim}
28%|██▊ | 390/1400 [00:50<01:48, 9.29it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 391/1400 [00:50<01:49, 9.22it/s]
</pre>
- 28%|██▊ | 391/1400 [00:50<01:49, 9.22it/s]
end{sphinxVerbatim}
28%|██▊ | 391/1400 [00:50<01:49, 9.22it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 393/1400 [00:50<01:30, 11.16it/s]
</pre>
- 28%|██▊ | 393/1400 [00:50<01:30, 11.16it/s]
end{sphinxVerbatim}
28%|██▊ | 393/1400 [00:50<01:30, 11.16it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 395/1400 [00:51<01:31, 11.01it/s]
</pre>
- 28%|██▊ | 395/1400 [00:51<01:31, 11.01it/s]
end{sphinxVerbatim}
28%|██▊ | 395/1400 [00:51<01:31, 11.01it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 397/1400 [00:51<01:34, 10.58it/s]
</pre>
- 28%|██▊ | 397/1400 [00:51<01:34, 10.58it/s]
end{sphinxVerbatim}
28%|██▊ | 397/1400 [00:51<01:34, 10.58it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 399/1400 [00:51<01:38, 10.12it/s]
</pre>
- 28%|██▊ | 399/1400 [00:51<01:38, 10.12it/s]
end{sphinxVerbatim}
28%|██▊ | 399/1400 [00:51<01:38, 10.12it/s]
- more-to-come:
- class:
stderr
- 29%|██▊ | 401/1400 [00:51<01:46, 9.36it/s]
</pre>
- 29%|██▊ | 401/1400 [00:51<01:46, 9.36it/s]
end{sphinxVerbatim}
29%|██▊ | 401/1400 [00:51<01:46, 9.36it/s]
- more-to-come:
- class:
stderr
- 29%|██▊ | 402/1400 [00:51<01:46, 9.38it/s]
</pre>
- 29%|██▊ | 402/1400 [00:51<01:46, 9.38it/s]
end{sphinxVerbatim}
29%|██▊ | 402/1400 [00:51<01:46, 9.38it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 403/1400 [00:51<01:45, 9.50it/s]
</pre>
- 29%|██▉ | 403/1400 [00:51<01:45, 9.50it/s]
end{sphinxVerbatim}
29%|██▉ | 403/1400 [00:51<01:45, 9.50it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 405/1400 [00:52<01:43, 9.61it/s]
</pre>
- 29%|██▉ | 405/1400 [00:52<01:43, 9.61it/s]
end{sphinxVerbatim}
29%|██▉ | 405/1400 [00:52<01:43, 9.61it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 407/1400 [00:52<01:39, 9.97it/s]
</pre>
- 29%|██▉ | 407/1400 [00:52<01:39, 9.97it/s]
end{sphinxVerbatim}
29%|██▉ | 407/1400 [00:52<01:39, 9.97it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 409/1400 [00:52<01:39, 9.91it/s]
</pre>
- 29%|██▉ | 409/1400 [00:52<01:39, 9.91it/s]
end{sphinxVerbatim}
29%|██▉ | 409/1400 [00:52<01:39, 9.91it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 410/1400 [00:52<01:44, 9.49it/s]
</pre>
- 29%|██▉ | 410/1400 [00:52<01:44, 9.49it/s]
end{sphinxVerbatim}
29%|██▉ | 410/1400 [00:52<01:44, 9.49it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 411/1400 [00:52<01:45, 9.40it/s]
</pre>
- 29%|██▉ | 411/1400 [00:52<01:45, 9.40it/s]
end{sphinxVerbatim}
29%|██▉ | 411/1400 [00:52<01:45, 9.40it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 412/1400 [00:52<01:44, 9.48it/s]
</pre>
- 29%|██▉ | 412/1400 [00:52<01:44, 9.48it/s]
end{sphinxVerbatim}
29%|██▉ | 412/1400 [00:52<01:44, 9.48it/s]
- more-to-come:
- class:
stderr
- 30%|██▉ | 414/1400 [00:53<01:31, 10.80it/s]
</pre>
- 30%|██▉ | 414/1400 [00:53<01:31, 10.80it/s]
end{sphinxVerbatim}
30%|██▉ | 414/1400 [00:53<01:31, 10.80it/s]
- more-to-come:
- class:
stderr
- 30%|██▉ | 416/1400 [00:53<01:35, 10.31it/s]
</pre>
- 30%|██▉ | 416/1400 [00:53<01:35, 10.31it/s]
end{sphinxVerbatim}
30%|██▉ | 416/1400 [00:53<01:35, 10.31it/s]
- more-to-come:
- class:
stderr
- 30%|██▉ | 418/1400 [00:53<01:36, 10.20it/s]
</pre>
- 30%|██▉ | 418/1400 [00:53<01:36, 10.20it/s]
end{sphinxVerbatim}
30%|██▉ | 418/1400 [00:53<01:36, 10.20it/s]
- more-to-come:
- class:
stderr
- 30%|███ | 420/1400 [00:53<01:29, 10.89it/s]
</pre>
- 30%|███ | 420/1400 [00:53<01:29, 10.89it/s]
end{sphinxVerbatim}
30%|███ | 420/1400 [00:53<01:29, 10.89it/s]
- more-to-come:
- class:
stderr
- 30%|███ | 422/1400 [00:53<01:22, 11.81it/s]
</pre>
- 30%|███ | 422/1400 [00:53<01:22, 11.81it/s]
end{sphinxVerbatim}
30%|███ | 422/1400 [00:53<01:22, 11.81it/s]
- more-to-come:
- class:
stderr
- 30%|███ | 424/1400 [00:54<01:32, 10.51it/s]
</pre>
- 30%|███ | 424/1400 [00:54<01:32, 10.51it/s]
end{sphinxVerbatim}
30%|███ | 424/1400 [00:54<01:32, 10.51it/s]
- more-to-come:
- class:
stderr
- 30%|███ | 426/1400 [00:54<01:39, 9.82it/s]
</pre>
- 30%|███ | 426/1400 [00:54<01:39, 9.82it/s]
end{sphinxVerbatim}
30%|███ | 426/1400 [00:54<01:39, 9.82it/s]
- more-to-come:
- class:
stderr
- 31%|███ | 428/1400 [00:54<01:28, 10.95it/s]
</pre>
- 31%|███ | 428/1400 [00:54<01:28, 10.95it/s]
end{sphinxVerbatim}
31%|███ | 428/1400 [00:54<01:28, 10.95it/s]
- more-to-come:
- class:
stderr
- 31%|███ | 430/1400 [00:54<01:35, 10.19it/s]
</pre>
- 31%|███ | 430/1400 [00:54<01:35, 10.19it/s]
end{sphinxVerbatim}
31%|███ | 430/1400 [00:54<01:35, 10.19it/s]
- more-to-come:
- class:
stderr
- 31%|███ | 432/1400 [00:54<01:36, 10.06it/s]
</pre>
- 31%|███ | 432/1400 [00:54<01:36, 10.06it/s]
end{sphinxVerbatim}
31%|███ | 432/1400 [00:54<01:36, 10.06it/s]
- more-to-come:
- class:
stderr
- 31%|███ | 434/1400 [00:55<01:40, 9.62it/s]
</pre>
- 31%|███ | 434/1400 [00:55<01:40, 9.62it/s]
end{sphinxVerbatim}
31%|███ | 434/1400 [00:55<01:40, 9.62it/s]
- more-to-come:
- class:
stderr
- 31%|███ | 435/1400 [00:55<01:44, 9.19it/s]
</pre>
- 31%|███ | 435/1400 [00:55<01:44, 9.19it/s]
end{sphinxVerbatim}
31%|███ | 435/1400 [00:55<01:44, 9.19it/s]
- more-to-come:
- class:
stderr
- 31%|███▏ | 438/1400 [00:55<01:24, 11.32it/s]
</pre>
- 31%|███▏ | 438/1400 [00:55<01:24, 11.32it/s]
end{sphinxVerbatim}
31%|███▏ | 438/1400 [00:55<01:24, 11.32it/s]
- more-to-come:
- class:
stderr
- 31%|███▏ | 440/1400 [00:55<01:30, 10.61it/s]
</pre>
- 31%|███▏ | 440/1400 [00:55<01:30, 10.61it/s]
end{sphinxVerbatim}
31%|███▏ | 440/1400 [00:55<01:30, 10.61it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 442/1400 [00:55<01:22, 11.68it/s]
</pre>
- 32%|███▏ | 442/1400 [00:55<01:22, 11.68it/s]
end{sphinxVerbatim}
32%|███▏ | 442/1400 [00:55<01:22, 11.68it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 444/1400 [00:55<01:26, 11.02it/s]
</pre>
- 32%|███▏ | 444/1400 [00:55<01:26, 11.02it/s]
end{sphinxVerbatim}
32%|███▏ | 444/1400 [00:55<01:26, 11.02it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 446/1400 [00:56<01:32, 10.34it/s]
</pre>
- 32%|███▏ | 446/1400 [00:56<01:32, 10.34it/s]
end{sphinxVerbatim}
32%|███▏ | 446/1400 [00:56<01:32, 10.34it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 448/1400 [00:56<01:36, 9.89it/s]
</pre>
- 32%|███▏ | 448/1400 [00:56<01:36, 9.89it/s]
end{sphinxVerbatim}
32%|███▏ | 448/1400 [00:56<01:36, 9.89it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 450/1400 [00:56<01:39, 9.58it/s]
</pre>
- 32%|███▏ | 450/1400 [00:56<01:39, 9.58it/s]
end{sphinxVerbatim}
32%|███▏ | 450/1400 [00:56<01:39, 9.58it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 452/1400 [00:56<01:37, 9.76it/s]
</pre>
- 32%|███▏ | 452/1400 [00:56<01:37, 9.76it/s]
end{sphinxVerbatim}
32%|███▏ | 452/1400 [00:56<01:37, 9.76it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 453/1400 [00:56<01:40, 9.40it/s]
</pre>
- 32%|███▏ | 453/1400 [00:56<01:40, 9.40it/s]
end{sphinxVerbatim}
32%|███▏ | 453/1400 [00:56<01:40, 9.40it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 454/1400 [00:57<01:39, 9.48it/s]
</pre>
- 32%|███▏ | 454/1400 [00:57<01:39, 9.48it/s]
end{sphinxVerbatim}
32%|███▏ | 454/1400 [00:57<01:39, 9.48it/s]
- more-to-come:
- class:
stderr
- 32%|███▎ | 455/1400 [00:57<01:45, 8.98it/s]
</pre>
- 32%|███▎ | 455/1400 [00:57<01:45, 8.98it/s]
end{sphinxVerbatim}
32%|███▎ | 455/1400 [00:57<01:45, 8.98it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 456/1400 [00:57<01:43, 9.12it/s]
</pre>
- 33%|███▎ | 456/1400 [00:57<01:43, 9.12it/s]
end{sphinxVerbatim}
33%|███▎ | 456/1400 [00:57<01:43, 9.12it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 457/1400 [00:57<01:42, 9.17it/s]
</pre>
- 33%|███▎ | 457/1400 [00:57<01:42, 9.17it/s]
end{sphinxVerbatim}
33%|███▎ | 457/1400 [00:57<01:42, 9.17it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 458/1400 [00:57<01:41, 9.30it/s]
</pre>
- 33%|███▎ | 458/1400 [00:57<01:41, 9.30it/s]
end{sphinxVerbatim}
33%|███▎ | 458/1400 [00:57<01:41, 9.30it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 459/1400 [00:57<01:43, 9.05it/s]
</pre>
- 33%|███▎ | 459/1400 [00:57<01:43, 9.05it/s]
end{sphinxVerbatim}
33%|███▎ | 459/1400 [00:57<01:43, 9.05it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 460/1400 [00:57<01:45, 8.87it/s]
</pre>
- 33%|███▎ | 460/1400 [00:57<01:45, 8.87it/s]
end{sphinxVerbatim}
33%|███▎ | 460/1400 [00:57<01:45, 8.87it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 461/1400 [00:57<01:46, 8.85it/s]
</pre>
- 33%|███▎ | 461/1400 [00:57<01:46, 8.85it/s]
end{sphinxVerbatim}
33%|███▎ | 461/1400 [00:57<01:46, 8.85it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 462/1400 [00:57<01:44, 8.96it/s]
</pre>
- 33%|███▎ | 462/1400 [00:57<01:44, 8.96it/s]
end{sphinxVerbatim}
33%|███▎ | 462/1400 [00:57<01:44, 8.96it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 463/1400 [00:58<01:46, 8.82it/s]
</pre>
- 33%|███▎ | 463/1400 [00:58<01:46, 8.82it/s]
end{sphinxVerbatim}
33%|███▎ | 463/1400 [00:58<01:46, 8.82it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 464/1400 [00:58<01:44, 8.98it/s]
</pre>
- 33%|███▎ | 464/1400 [00:58<01:44, 8.98it/s]
end{sphinxVerbatim}
33%|███▎ | 464/1400 [00:58<01:44, 8.98it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 465/1400 [00:58<01:49, 8.53it/s]
</pre>
- 33%|███▎ | 465/1400 [00:58<01:49, 8.53it/s]
end{sphinxVerbatim}
33%|███▎ | 465/1400 [00:58<01:49, 8.53it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 466/1400 [00:58<01:45, 8.87it/s]
</pre>
- 33%|███▎ | 466/1400 [00:58<01:45, 8.87it/s]
end{sphinxVerbatim}
33%|███▎ | 466/1400 [00:58<01:45, 8.87it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 467/1400 [00:58<01:43, 9.05it/s]
</pre>
- 33%|███▎ | 467/1400 [00:58<01:43, 9.05it/s]
end{sphinxVerbatim}
33%|███▎ | 467/1400 [00:58<01:43, 9.05it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 468/1400 [00:58<01:45, 8.87it/s]
</pre>
- 33%|███▎ | 468/1400 [00:58<01:45, 8.87it/s]
end{sphinxVerbatim}
33%|███▎ | 468/1400 [00:58<01:45, 8.87it/s]
- more-to-come:
- class:
stderr
- 34%|███▎ | 469/1400 [00:58<01:43, 9.02it/s]
</pre>
- 34%|███▎ | 469/1400 [00:58<01:43, 9.02it/s]
end{sphinxVerbatim}
34%|███▎ | 469/1400 [00:58<01:43, 9.02it/s]
- more-to-come:
- class:
stderr
- 34%|███▎ | 470/1400 [00:58<01:51, 8.36it/s]
</pre>
- 34%|███▎ | 470/1400 [00:58<01:51, 8.36it/s]
end{sphinxVerbatim}
34%|███▎ | 470/1400 [00:58<01:51, 8.36it/s]
- more-to-come:
- class:
stderr
- 34%|███▎ | 471/1400 [00:58<01:47, 8.66it/s]
</pre>
- 34%|███▎ | 471/1400 [00:58<01:47, 8.66it/s]
end{sphinxVerbatim}
34%|███▎ | 471/1400 [00:58<01:47, 8.66it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 473/1400 [00:59<01:40, 9.27it/s]
</pre>
- 34%|███▍ | 473/1400 [00:59<01:40, 9.27it/s]
end{sphinxVerbatim}
34%|███▍ | 473/1400 [00:59<01:40, 9.27it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 474/1400 [00:59<01:40, 9.17it/s]
</pre>
- 34%|███▍ | 474/1400 [00:59<01:40, 9.17it/s]
end{sphinxVerbatim}
34%|███▍ | 474/1400 [00:59<01:40, 9.17it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 475/1400 [00:59<01:49, 8.45it/s]
</pre>
- 34%|███▍ | 475/1400 [00:59<01:49, 8.45it/s]
end{sphinxVerbatim}
34%|███▍ | 475/1400 [00:59<01:49, 8.45it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 476/1400 [00:59<01:47, 8.61it/s]
</pre>
- 34%|███▍ | 476/1400 [00:59<01:47, 8.61it/s]
end{sphinxVerbatim}
34%|███▍ | 476/1400 [00:59<01:47, 8.61it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 478/1400 [00:59<01:28, 10.36it/s]
</pre>
- 34%|███▍ | 478/1400 [00:59<01:28, 10.36it/s]
end{sphinxVerbatim}
34%|███▍ | 478/1400 [00:59<01:28, 10.36it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 480/1400 [00:59<01:34, 9.72it/s]
</pre>
- 34%|███▍ | 480/1400 [00:59<01:34, 9.72it/s]
end{sphinxVerbatim}
34%|███▍ | 480/1400 [00:59<01:34, 9.72it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 482/1400 [01:00<01:22, 11.16it/s]
</pre>
- 34%|███▍ | 482/1400 [01:00<01:22, 11.16it/s]
end{sphinxVerbatim}
34%|███▍ | 482/1400 [01:00<01:22, 11.16it/s]
- more-to-come:
- class:
stderr
- 35%|███▍ | 484/1400 [01:00<01:21, 11.17it/s]
</pre>
- 35%|███▍ | 484/1400 [01:00<01:21, 11.17it/s]
end{sphinxVerbatim}
35%|███▍ | 484/1400 [01:00<01:21, 11.17it/s]
- more-to-come:
- class:
stderr
- 35%|███▍ | 486/1400 [01:00<01:28, 10.33it/s]
</pre>
- 35%|███▍ | 486/1400 [01:00<01:28, 10.33it/s]
end{sphinxVerbatim}
35%|███▍ | 486/1400 [01:00<01:28, 10.33it/s]
- more-to-come:
- class:
stderr
- 35%|███▍ | 488/1400 [01:00<01:16, 11.87it/s]
</pre>
- 35%|███▍ | 488/1400 [01:00<01:16, 11.87it/s]
end{sphinxVerbatim}
35%|███▍ | 488/1400 [01:00<01:16, 11.87it/s]
- more-to-come:
- class:
stderr
- 35%|███▌ | 490/1400 [01:00<01:20, 11.34it/s]
</pre>
- 35%|███▌ | 490/1400 [01:00<01:20, 11.34it/s]
end{sphinxVerbatim}
35%|███▌ | 490/1400 [01:00<01:20, 11.34it/s]
- more-to-come:
- class:
stderr
- 35%|███▌ | 492/1400 [01:00<01:21, 11.16it/s]
</pre>
- 35%|███▌ | 492/1400 [01:00<01:21, 11.16it/s]
end{sphinxVerbatim}
35%|███▌ | 492/1400 [01:00<01:21, 11.16it/s]
- more-to-come:
- class:
stderr
- 35%|███▌ | 494/1400 [01:01<01:26, 10.53it/s]
</pre>
- 35%|███▌ | 494/1400 [01:01<01:26, 10.53it/s]
end{sphinxVerbatim}
35%|███▌ | 494/1400 [01:01<01:26, 10.53it/s]
- more-to-come:
- class:
stderr
- 35%|███▌ | 496/1400 [01:01<01:33, 9.71it/s]
</pre>
- 35%|███▌ | 496/1400 [01:01<01:33, 9.71it/s]
end{sphinxVerbatim}
35%|███▌ | 496/1400 [01:01<01:33, 9.71it/s]
- more-to-come:
- class:
stderr
- 36%|███▌ | 498/1400 [01:01<01:24, 10.70it/s]
</pre>
- 36%|███▌ | 498/1400 [01:01<01:24, 10.70it/s]
end{sphinxVerbatim}
36%|███▌ | 498/1400 [01:01<01:24, 10.70it/s]
- more-to-come:
- class:
stderr
- 36%|███▌ | 500/1400 [01:01<01:29, 10.04it/s]
</pre>
- 36%|███▌ | 500/1400 [01:01<01:29, 10.04it/s]
end{sphinxVerbatim}
36%|███▌ | 500/1400 [01:01<01:29, 10.04it/s]
- more-to-come:
- class:
stderr
- 36%|███▌ | 502/1400 [01:01<01:36, 9.31it/s]
</pre>
- 36%|███▌ | 502/1400 [01:01<01:36, 9.31it/s]
end{sphinxVerbatim}
36%|███▌ | 502/1400 [01:01<01:36, 9.31it/s]
- more-to-come:
- class:
stderr
- 36%|███▌ | 503/1400 [01:02<01:35, 9.39it/s]
</pre>
- 36%|███▌ | 503/1400 [01:02<01:35, 9.39it/s]
end{sphinxVerbatim}
36%|███▌ | 503/1400 [01:02<01:35, 9.39it/s]
- more-to-come:
- class:
stderr
- 36%|███▌ | 505/1400 [01:02<01:37, 9.13it/s]
</pre>
- 36%|███▌ | 505/1400 [01:02<01:37, 9.13it/s]
end{sphinxVerbatim}
36%|███▌ | 505/1400 [01:02<01:37, 9.13it/s]
- more-to-come:
- class:
stderr
- 36%|███▌ | 506/1400 [01:02<01:36, 9.27it/s]
</pre>
- 36%|███▌ | 506/1400 [01:02<01:36, 9.27it/s]
end{sphinxVerbatim}
36%|███▌ | 506/1400 [01:02<01:36, 9.27it/s]
- more-to-come:
- class:
stderr
- 36%|███▋ | 508/1400 [01:02<01:20, 11.09it/s]
</pre>
- 36%|███▋ | 508/1400 [01:02<01:20, 11.09it/s]
end{sphinxVerbatim}
36%|███▋ | 508/1400 [01:02<01:20, 11.09it/s]
- more-to-come:
- class:
stderr
- 36%|███▋ | 510/1400 [01:02<01:27, 10.18it/s]
</pre>
- 36%|███▋ | 510/1400 [01:02<01:27, 10.18it/s]
end{sphinxVerbatim}
36%|███▋ | 510/1400 [01:02<01:27, 10.18it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 512/1400 [01:02<01:27, 10.11it/s]
</pre>
- 37%|███▋ | 512/1400 [01:02<01:27, 10.11it/s]
end{sphinxVerbatim}
37%|███▋ | 512/1400 [01:02<01:27, 10.11it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 514/1400 [01:03<01:19, 11.21it/s]
</pre>
- 37%|███▋ | 514/1400 [01:03<01:19, 11.21it/s]
end{sphinxVerbatim}
37%|███▋ | 514/1400 [01:03<01:19, 11.21it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 516/1400 [01:03<01:28, 9.97it/s]
</pre>
- 37%|███▋ | 516/1400 [01:03<01:28, 9.97it/s]
end{sphinxVerbatim}
37%|███▋ | 516/1400 [01:03<01:28, 9.97it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 518/1400 [01:03<01:33, 9.39it/s]
</pre>
- 37%|███▋ | 518/1400 [01:03<01:33, 9.39it/s]
end{sphinxVerbatim}
37%|███▋ | 518/1400 [01:03<01:33, 9.39it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 519/1400 [01:03<01:41, 8.65it/s]
</pre>
- 37%|███▋ | 519/1400 [01:03<01:41, 8.65it/s]
end{sphinxVerbatim}
37%|███▋ | 519/1400 [01:03<01:41, 8.65it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 520/1400 [01:03<01:47, 8.20it/s]
</pre>
- 37%|███▋ | 520/1400 [01:03<01:47, 8.20it/s]
end{sphinxVerbatim}
37%|███▋ | 520/1400 [01:03<01:47, 8.20it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 521/1400 [01:04<01:47, 8.21it/s]
</pre>
- 37%|███▋ | 521/1400 [01:04<01:47, 8.21it/s]
end{sphinxVerbatim}
37%|███▋ | 521/1400 [01:04<01:47, 8.21it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 523/1400 [01:04<01:24, 10.32it/s]
</pre>
- 37%|███▋ | 523/1400 [01:04<01:24, 10.32it/s]
end{sphinxVerbatim}
37%|███▋ | 523/1400 [01:04<01:24, 10.32it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 525/1400 [01:04<01:26, 10.09it/s]
</pre>
- 38%|███▊ | 525/1400 [01:04<01:26, 10.09it/s]
end{sphinxVerbatim}
38%|███▊ | 525/1400 [01:04<01:26, 10.09it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 527/1400 [01:04<01:21, 10.77it/s]
</pre>
- 38%|███▊ | 527/1400 [01:04<01:21, 10.77it/s]
end{sphinxVerbatim}
38%|███▊ | 527/1400 [01:04<01:21, 10.77it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 529/1400 [01:04<01:11, 12.21it/s]
</pre>
- 38%|███▊ | 529/1400 [01:04<01:11, 12.21it/s]
end{sphinxVerbatim}
38%|███▊ | 529/1400 [01:04<01:11, 12.21it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 531/1400 [01:04<01:16, 11.33it/s]
</pre>
- 38%|███▊ | 531/1400 [01:04<01:16, 11.33it/s]
end{sphinxVerbatim}
38%|███▊ | 531/1400 [01:04<01:16, 11.33it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 533/1400 [01:04<01:08, 12.65it/s]
</pre>
- 38%|███▊ | 533/1400 [01:04<01:08, 12.65it/s]
end{sphinxVerbatim}
38%|███▊ | 533/1400 [01:04<01:08, 12.65it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 535/1400 [01:05<01:16, 11.37it/s]
</pre>
- 38%|███▊ | 535/1400 [01:05<01:16, 11.37it/s]
end{sphinxVerbatim}
38%|███▊ | 535/1400 [01:05<01:16, 11.37it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 537/1400 [01:05<01:07, 12.74it/s]
</pre>
- 38%|███▊ | 537/1400 [01:05<01:07, 12.74it/s]
end{sphinxVerbatim}
38%|███▊ | 537/1400 [01:05<01:07, 12.74it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 539/1400 [01:05<01:01, 14.01it/s]
</pre>
- 38%|███▊ | 539/1400 [01:05<01:01, 14.01it/s]
end{sphinxVerbatim}
38%|███▊ | 539/1400 [01:05<01:01, 14.01it/s]
- more-to-come:
- class:
stderr
- 39%|███▊ | 541/1400 [01:05<01:07, 12.67it/s]
</pre>
- 39%|███▊ | 541/1400 [01:05<01:07, 12.67it/s]
end{sphinxVerbatim}
39%|███▊ | 541/1400 [01:05<01:07, 12.67it/s]
- more-to-come:
- class:
stderr
- 39%|███▉ | 543/1400 [01:05<01:03, 13.43it/s]
</pre>
- 39%|███▉ | 543/1400 [01:05<01:03, 13.43it/s]
end{sphinxVerbatim}
39%|███▉ | 543/1400 [01:05<01:03, 13.43it/s]
- more-to-come:
- class:
stderr
- 39%|███▉ | 545/1400 [01:05<01:10, 12.18it/s]
</pre>
- 39%|███▉ | 545/1400 [01:05<01:10, 12.18it/s]
end{sphinxVerbatim}
39%|███▉ | 545/1400 [01:05<01:10, 12.18it/s]
- more-to-come:
- class:
stderr
- 39%|███▉ | 547/1400 [01:06<01:06, 12.86it/s]
</pre>
- 39%|███▉ | 547/1400 [01:06<01:06, 12.86it/s]
end{sphinxVerbatim}
39%|███▉ | 547/1400 [01:06<01:06, 12.86it/s]
- more-to-come:
- class:
stderr
- 39%|███▉ | 549/1400 [01:06<01:11, 11.86it/s]
</pre>
- 39%|███▉ | 549/1400 [01:06<01:11, 11.86it/s]
end{sphinxVerbatim}
39%|███▉ | 549/1400 [01:06<01:11, 11.86it/s]
- more-to-come:
- class:
stderr
- 39%|███▉ | 551/1400 [01:06<01:11, 11.90it/s]
</pre>
- 39%|███▉ | 551/1400 [01:06<01:11, 11.90it/s]
end{sphinxVerbatim}
39%|███▉ | 551/1400 [01:06<01:11, 11.90it/s]
- more-to-come:
- class:
stderr
- 40%|███▉ | 553/1400 [01:06<01:06, 12.77it/s]
</pre>
- 40%|███▉ | 553/1400 [01:06<01:06, 12.77it/s]
end{sphinxVerbatim}
40%|███▉ | 553/1400 [01:06<01:06, 12.77it/s]
- more-to-come:
- class:
stderr
- 40%|███▉ | 555/1400 [01:06<01:11, 11.78it/s]
</pre>
- 40%|███▉ | 555/1400 [01:06<01:11, 11.78it/s]
end{sphinxVerbatim}
40%|███▉ | 555/1400 [01:06<01:11, 11.78it/s]
- more-to-come:
- class:
stderr
- 40%|███▉ | 557/1400 [01:06<01:14, 11.25it/s]
</pre>
- 40%|███▉ | 557/1400 [01:06<01:14, 11.25it/s]
end{sphinxVerbatim}
40%|███▉ | 557/1400 [01:06<01:14, 11.25it/s]
- more-to-come:
- class:
stderr
- 40%|███▉ | 559/1400 [01:07<01:18, 10.71it/s]
</pre>
- 40%|███▉ | 559/1400 [01:07<01:18, 10.71it/s]
end{sphinxVerbatim}
40%|███▉ | 559/1400 [01:07<01:18, 10.71it/s]
- more-to-come:
- class:
stderr
- 40%|████ | 561/1400 [01:07<01:11, 11.68it/s]
</pre>
- 40%|████ | 561/1400 [01:07<01:11, 11.68it/s]
end{sphinxVerbatim}
40%|████ | 561/1400 [01:07<01:11, 11.68it/s]
- more-to-come:
- class:
stderr
- 40%|████ | 563/1400 [01:07<01:13, 11.39it/s]
</pre>
- 40%|████ | 563/1400 [01:07<01:13, 11.39it/s]
end{sphinxVerbatim}
40%|████ | 563/1400 [01:07<01:13, 11.39it/s]
- more-to-come:
- class:
stderr
- 40%|████ | 566/1400 [01:07<00:56, 14.86it/s]
</pre>
- 40%|████ | 566/1400 [01:07<00:56, 14.86it/s]
end{sphinxVerbatim}
40%|████ | 566/1400 [01:07<00:56, 14.86it/s]
- more-to-come:
- class:
stderr
- 41%|████ | 568/1400 [01:07<00:56, 14.65it/s]
</pre>
- 41%|████ | 568/1400 [01:07<00:56, 14.65it/s]
end{sphinxVerbatim}
41%|████ | 568/1400 [01:07<00:56, 14.65it/s]
- more-to-come:
- class:
stderr
- 41%|████ | 570/1400 [01:07<00:55, 14.87it/s]
</pre>
- 41%|████ | 570/1400 [01:07<00:55, 14.87it/s]
end{sphinxVerbatim}
41%|████ | 570/1400 [01:07<00:55, 14.87it/s]
- more-to-come:
- class:
stderr
- 41%|████ | 572/1400 [01:07<00:55, 14.88it/s]
</pre>
- 41%|████ | 572/1400 [01:07<00:55, 14.88it/s]
end{sphinxVerbatim}
41%|████ | 572/1400 [01:07<00:55, 14.88it/s]
- more-to-come:
- class:
stderr
- 41%|████ | 574/1400 [01:08<01:03, 13.00it/s]
</pre>
- 41%|████ | 574/1400 [01:08<01:03, 13.00it/s]
end{sphinxVerbatim}
41%|████ | 574/1400 [01:08<01:03, 13.00it/s]
- more-to-come:
- class:
stderr
- 41%|████ | 576/1400 [01:08<01:06, 12.38it/s]
</pre>
- 41%|████ | 576/1400 [01:08<01:06, 12.38it/s]
end{sphinxVerbatim}
41%|████ | 576/1400 [01:08<01:06, 12.38it/s]
- more-to-come:
- class:
stderr
- 41%|████▏ | 578/1400 [01:08<01:00, 13.49it/s]
</pre>
- 41%|████▏ | 578/1400 [01:08<01:00, 13.49it/s]
end{sphinxVerbatim}
41%|████▏ | 578/1400 [01:08<01:00, 13.49it/s]
- more-to-come:
- class:
stderr
- 41%|████▏ | 580/1400 [01:08<01:00, 13.51it/s]
</pre>
- 41%|████▏ | 580/1400 [01:08<01:00, 13.51it/s]
end{sphinxVerbatim}
41%|████▏ | 580/1400 [01:08<01:00, 13.51it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 582/1400 [01:08<00:57, 14.35it/s]
</pre>
- 42%|████▏ | 582/1400 [01:08<00:57, 14.35it/s]
end{sphinxVerbatim}
42%|████▏ | 582/1400 [01:08<00:57, 14.35it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 584/1400 [01:08<01:03, 12.77it/s]
</pre>
- 42%|████▏ | 584/1400 [01:08<01:03, 12.77it/s]
end{sphinxVerbatim}
42%|████▏ | 584/1400 [01:08<01:03, 12.77it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 586/1400 [01:09<01:08, 11.95it/s]
</pre>
- 42%|████▏ | 586/1400 [01:09<01:08, 11.95it/s]
end{sphinxVerbatim}
42%|████▏ | 586/1400 [01:09<01:08, 11.95it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 588/1400 [01:09<01:11, 11.30it/s]
</pre>
- 42%|████▏ | 588/1400 [01:09<01:11, 11.30it/s]
end{sphinxVerbatim}
42%|████▏ | 588/1400 [01:09<01:11, 11.30it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 590/1400 [01:09<01:15, 10.73it/s]
</pre>
- 42%|████▏ | 590/1400 [01:09<01:15, 10.73it/s]
end{sphinxVerbatim}
42%|████▏ | 590/1400 [01:09<01:15, 10.73it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 592/1400 [01:09<01:08, 11.71it/s]
</pre>
- 42%|████▏ | 592/1400 [01:09<01:08, 11.71it/s]
end{sphinxVerbatim}
42%|████▏ | 592/1400 [01:09<01:08, 11.71it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 594/1400 [01:09<01:03, 12.60it/s]
</pre>
- 42%|████▏ | 594/1400 [01:09<01:03, 12.60it/s]
end{sphinxVerbatim}
42%|████▏ | 594/1400 [01:09<01:03, 12.60it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 596/1400 [01:09<01:06, 12.18it/s]
</pre>
- 43%|████▎ | 596/1400 [01:09<01:06, 12.18it/s]
end{sphinxVerbatim}
43%|████▎ | 596/1400 [01:09<01:06, 12.18it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 598/1400 [01:10<01:11, 11.22it/s]
</pre>
- 43%|████▎ | 598/1400 [01:10<01:11, 11.22it/s]
end{sphinxVerbatim}
43%|████▎ | 598/1400 [01:10<01:11, 11.22it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 600/1400 [01:10<01:17, 10.28it/s]
</pre>
- 43%|████▎ | 600/1400 [01:10<01:17, 10.28it/s]
end{sphinxVerbatim}
43%|████▎ | 600/1400 [01:10<01:17, 10.28it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 602/1400 [01:10<01:08, 11.64it/s]
</pre>
- 43%|████▎ | 602/1400 [01:10<01:08, 11.64it/s]
end{sphinxVerbatim}
43%|████▎ | 602/1400 [01:10<01:08, 11.64it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 604/1400 [01:10<01:02, 12.64it/s]
</pre>
- 43%|████▎ | 604/1400 [01:10<01:02, 12.64it/s]
end{sphinxVerbatim}
43%|████▎ | 604/1400 [01:10<01:02, 12.64it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 606/1400 [01:10<01:10, 11.27it/s]
</pre>
- 43%|████▎ | 606/1400 [01:10<01:10, 11.27it/s]
end{sphinxVerbatim}
43%|████▎ | 606/1400 [01:10<01:10, 11.27it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 608/1400 [01:11<01:13, 10.80it/s]
</pre>
- 43%|████▎ | 608/1400 [01:11<01:13, 10.80it/s]
end{sphinxVerbatim}
43%|████▎ | 608/1400 [01:11<01:13, 10.80it/s]
- more-to-come:
- class:
stderr
- 44%|████▎ | 610/1400 [01:11<01:08, 11.58it/s]
</pre>
- 44%|████▎ | 610/1400 [01:11<01:08, 11.58it/s]
end{sphinxVerbatim}
44%|████▎ | 610/1400 [01:11<01:08, 11.58it/s]
- more-to-come:
- class:
stderr
- 44%|████▎ | 612/1400 [01:11<01:10, 11.14it/s]
</pre>
- 44%|████▎ | 612/1400 [01:11<01:10, 11.14it/s]
end{sphinxVerbatim}
44%|████▎ | 612/1400 [01:11<01:10, 11.14it/s]
- more-to-come:
- class:
stderr
- 44%|████▍ | 614/1400 [01:11<01:10, 11.08it/s]
</pre>
- 44%|████▍ | 614/1400 [01:11<01:10, 11.08it/s]
end{sphinxVerbatim}
44%|████▍ | 614/1400 [01:11<01:10, 11.08it/s]
- more-to-come:
- class:
stderr
- 44%|████▍ | 616/1400 [01:11<01:07, 11.67it/s]
</pre>
- 44%|████▍ | 616/1400 [01:11<01:07, 11.67it/s]
end{sphinxVerbatim}
44%|████▍ | 616/1400 [01:11<01:07, 11.67it/s]
- more-to-come:
- class:
stderr
- 44%|████▍ | 618/1400 [01:11<01:09, 11.21it/s]
</pre>
- 44%|████▍ | 618/1400 [01:11<01:09, 11.21it/s]
end{sphinxVerbatim}
44%|████▍ | 618/1400 [01:11<01:09, 11.21it/s]
- more-to-come:
- class:
stderr
- 44%|████▍ | 620/1400 [01:12<01:12, 10.72it/s]
</pre>
- 44%|████▍ | 620/1400 [01:12<01:12, 10.72it/s]
end{sphinxVerbatim}
44%|████▍ | 620/1400 [01:12<01:12, 10.72it/s]
- more-to-come:
- class:
stderr
- 44%|████▍ | 622/1400 [01:12<01:12, 10.76it/s]
</pre>
- 44%|████▍ | 622/1400 [01:12<01:12, 10.76it/s]
end{sphinxVerbatim}
44%|████▍ | 622/1400 [01:12<01:12, 10.76it/s]
- more-to-come:
- class:
stderr
- 45%|████▍ | 624/1400 [01:12<01:06, 11.66it/s]
</pre>
- 45%|████▍ | 624/1400 [01:12<01:06, 11.66it/s]
end{sphinxVerbatim}
45%|████▍ | 624/1400 [01:12<01:06, 11.66it/s]
- more-to-come:
- class:
stderr
- 45%|████▍ | 626/1400 [01:12<01:11, 10.89it/s]
</pre>
- 45%|████▍ | 626/1400 [01:12<01:11, 10.89it/s]
end{sphinxVerbatim}
45%|████▍ | 626/1400 [01:12<01:11, 10.89it/s]
- more-to-come:
- class:
stderr
- 45%|████▍ | 628/1400 [01:12<01:04, 11.89it/s]
</pre>
- 45%|████▍ | 628/1400 [01:12<01:04, 11.89it/s]
end{sphinxVerbatim}
45%|████▍ | 628/1400 [01:12<01:04, 11.89it/s]
- more-to-come:
- class:
stderr
- 45%|████▌ | 630/1400 [01:13<01:06, 11.52it/s]
</pre>
- 45%|████▌ | 630/1400 [01:13<01:06, 11.52it/s]
end{sphinxVerbatim}
45%|████▌ | 630/1400 [01:13<01:06, 11.52it/s]
- more-to-come:
- class:
stderr
- 45%|████▌ | 632/1400 [01:13<01:10, 10.82it/s]
</pre>
- 45%|████▌ | 632/1400 [01:13<01:10, 10.82it/s]
end{sphinxVerbatim}
45%|████▌ | 632/1400 [01:13<01:10, 10.82it/s]
- more-to-come:
- class:
stderr
- 45%|████▌ | 634/1400 [01:13<01:12, 10.55it/s]
</pre>
- 45%|████▌ | 634/1400 [01:13<01:12, 10.55it/s]
end{sphinxVerbatim}
45%|████▌ | 634/1400 [01:13<01:12, 10.55it/s]
- more-to-come:
- class:
stderr
- 45%|████▌ | 636/1400 [01:13<01:15, 10.11it/s]
</pre>
- 45%|████▌ | 636/1400 [01:13<01:15, 10.11it/s]
end{sphinxVerbatim}
45%|████▌ | 636/1400 [01:13<01:15, 10.11it/s]
- more-to-come:
- class:
stderr
- 46%|████▌ | 638/1400 [01:13<01:16, 9.95it/s]
</pre>
- 46%|████▌ | 638/1400 [01:13<01:16, 9.95it/s]
end{sphinxVerbatim}
46%|████▌ | 638/1400 [01:13<01:16, 9.95it/s]
- more-to-come:
- class:
stderr
- 46%|████▌ | 640/1400 [01:14<01:17, 9.80it/s]
</pre>
- 46%|████▌ | 640/1400 [01:14<01:17, 9.80it/s]
end{sphinxVerbatim}
46%|████▌ | 640/1400 [01:14<01:17, 9.80it/s]
- more-to-come:
- class:
stderr
- 46%|████▌ | 642/1400 [01:14<01:16, 9.90it/s]
</pre>
- 46%|████▌ | 642/1400 [01:14<01:16, 9.90it/s]
end{sphinxVerbatim}
46%|████▌ | 642/1400 [01:14<01:16, 9.90it/s]
- more-to-come:
- class:
stderr
- 46%|████▌ | 644/1400 [01:14<01:16, 9.93it/s]
</pre>
- 46%|████▌ | 644/1400 [01:14<01:16, 9.93it/s]
end{sphinxVerbatim}
46%|████▌ | 644/1400 [01:14<01:16, 9.93it/s]
- more-to-come:
- class:
stderr
- 46%|████▌ | 646/1400 [01:14<01:09, 10.92it/s]
</pre>
- 46%|████▌ | 646/1400 [01:14<01:09, 10.92it/s]
end{sphinxVerbatim}
46%|████▌ | 646/1400 [01:14<01:09, 10.92it/s]
- more-to-come:
- class:
stderr
- 46%|████▋ | 648/1400 [01:14<01:06, 11.38it/s]
</pre>
- 46%|████▋ | 648/1400 [01:14<01:06, 11.38it/s]
end{sphinxVerbatim}
46%|████▋ | 648/1400 [01:14<01:06, 11.38it/s]
- more-to-come:
- class:
stderr
- 46%|████▋ | 650/1400 [01:15<01:14, 10.12it/s]
</pre>
- 46%|████▋ | 650/1400 [01:15<01:14, 10.12it/s]
end{sphinxVerbatim}
46%|████▋ | 650/1400 [01:15<01:14, 10.12it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 652/1400 [01:15<01:14, 10.08it/s]
</pre>
- 47%|████▋ | 652/1400 [01:15<01:14, 10.08it/s]
end{sphinxVerbatim}
47%|████▋ | 652/1400 [01:15<01:14, 10.08it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 654/1400 [01:15<01:15, 9.87it/s]
</pre>
- 47%|████▋ | 654/1400 [01:15<01:15, 9.87it/s]
end{sphinxVerbatim}
47%|████▋ | 654/1400 [01:15<01:15, 9.87it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 656/1400 [01:15<01:18, 9.45it/s]
</pre>
- 47%|████▋ | 656/1400 [01:15<01:18, 9.45it/s]
end{sphinxVerbatim}
47%|████▋ | 656/1400 [01:15<01:18, 9.45it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 657/1400 [01:15<01:20, 9.26it/s]
</pre>
- 47%|████▋ | 657/1400 [01:15<01:20, 9.26it/s]
end{sphinxVerbatim}
47%|████▋ | 657/1400 [01:15<01:20, 9.26it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 658/1400 [01:15<01:20, 9.19it/s]
</pre>
- 47%|████▋ | 658/1400 [01:15<01:20, 9.19it/s]
end{sphinxVerbatim}
47%|████▋ | 658/1400 [01:15<01:20, 9.19it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 660/1400 [01:16<01:20, 9.19it/s]
</pre>
- 47%|████▋ | 660/1400 [01:16<01:20, 9.19it/s]
end{sphinxVerbatim}
47%|████▋ | 660/1400 [01:16<01:20, 9.19it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 661/1400 [01:16<01:19, 9.28it/s]
</pre>
- 47%|████▋ | 661/1400 [01:16<01:19, 9.28it/s]
end{sphinxVerbatim}
47%|████▋ | 661/1400 [01:16<01:19, 9.28it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 662/1400 [01:16<01:21, 9.01it/s]
</pre>
- 47%|████▋ | 662/1400 [01:16<01:21, 9.01it/s]
end{sphinxVerbatim}
47%|████▋ | 662/1400 [01:16<01:21, 9.01it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 664/1400 [01:16<01:19, 9.20it/s]
</pre>
- 47%|████▋ | 664/1400 [01:16<01:19, 9.20it/s]
end{sphinxVerbatim}
47%|████▋ | 664/1400 [01:16<01:19, 9.20it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 665/1400 [01:16<01:19, 9.21it/s]
</pre>
- 48%|████▊ | 665/1400 [01:16<01:19, 9.21it/s]
end{sphinxVerbatim}
48%|████▊ | 665/1400 [01:16<01:19, 9.21it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 667/1400 [01:16<01:07, 10.83it/s]
</pre>
- 48%|████▊ | 667/1400 [01:16<01:07, 10.83it/s]
end{sphinxVerbatim}
48%|████▊ | 667/1400 [01:16<01:07, 10.83it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 669/1400 [01:17<01:13, 9.91it/s]
</pre>
- 48%|████▊ | 669/1400 [01:17<01:13, 9.91it/s]
end{sphinxVerbatim}
48%|████▊ | 669/1400 [01:17<01:13, 9.91it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 671/1400 [01:17<01:16, 9.58it/s]
</pre>
- 48%|████▊ | 671/1400 [01:17<01:16, 9.58it/s]
end{sphinxVerbatim}
48%|████▊ | 671/1400 [01:17<01:16, 9.58it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 672/1400 [01:17<01:15, 9.64it/s]
</pre>
- 48%|████▊ | 672/1400 [01:17<01:15, 9.64it/s]
end{sphinxVerbatim}
48%|████▊ | 672/1400 [01:17<01:15, 9.64it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 673/1400 [01:17<01:15, 9.57it/s]
</pre>
- 48%|████▊ | 673/1400 [01:17<01:15, 9.57it/s]
end{sphinxVerbatim}
48%|████▊ | 673/1400 [01:17<01:15, 9.57it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 674/1400 [01:17<01:18, 9.30it/s]
</pre>
- 48%|████▊ | 674/1400 [01:17<01:18, 9.30it/s]
end{sphinxVerbatim}
48%|████▊ | 674/1400 [01:17<01:18, 9.30it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 675/1400 [01:17<01:19, 9.12it/s]
</pre>
- 48%|████▊ | 675/1400 [01:17<01:19, 9.12it/s]
end{sphinxVerbatim}
48%|████▊ | 675/1400 [01:17<01:19, 9.12it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 676/1400 [01:17<01:19, 9.16it/s]
</pre>
- 48%|████▊ | 676/1400 [01:17<01:19, 9.16it/s]
end{sphinxVerbatim}
48%|████▊ | 676/1400 [01:17<01:19, 9.16it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 677/1400 [01:17<01:18, 9.19it/s]
</pre>
- 48%|████▊ | 677/1400 [01:17<01:18, 9.19it/s]
end{sphinxVerbatim}
48%|████▊ | 677/1400 [01:17<01:18, 9.19it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 678/1400 [01:18<01:21, 8.85it/s]
</pre>
- 48%|████▊ | 678/1400 [01:18<01:21, 8.85it/s]
end{sphinxVerbatim}
48%|████▊ | 678/1400 [01:18<01:21, 8.85it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 679/1400 [01:18<01:20, 8.93it/s]
</pre>
- 48%|████▊ | 679/1400 [01:18<01:20, 8.93it/s]
end{sphinxVerbatim}
48%|████▊ | 679/1400 [01:18<01:20, 8.93it/s]
- more-to-come:
- class:
stderr
- 49%|████▊ | 680/1400 [01:18<01:24, 8.57it/s]
</pre>
- 49%|████▊ | 680/1400 [01:18<01:24, 8.57it/s]
end{sphinxVerbatim}
49%|████▊ | 680/1400 [01:18<01:24, 8.57it/s]
- more-to-come:
- class:
stderr
- 49%|████▊ | 682/1400 [01:18<01:16, 9.36it/s]
</pre>
- 49%|████▊ | 682/1400 [01:18<01:16, 9.36it/s]
end{sphinxVerbatim}
49%|████▊ | 682/1400 [01:18<01:16, 9.36it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 683/1400 [01:18<01:16, 9.35it/s]
</pre>
- 49%|████▉ | 683/1400 [01:18<01:16, 9.35it/s]
end{sphinxVerbatim}
49%|████▉ | 683/1400 [01:18<01:16, 9.35it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 684/1400 [01:18<01:15, 9.51it/s]
</pre>
- 49%|████▉ | 684/1400 [01:18<01:15, 9.51it/s]
end{sphinxVerbatim}
49%|████▉ | 684/1400 [01:18<01:15, 9.51it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 685/1400 [01:18<01:15, 9.51it/s]
</pre>
- 49%|████▉ | 685/1400 [01:18<01:15, 9.51it/s]
end{sphinxVerbatim}
49%|████▉ | 685/1400 [01:18<01:15, 9.51it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 686/1400 [01:18<01:15, 9.48it/s]
</pre>
- 49%|████▉ | 686/1400 [01:18<01:15, 9.48it/s]
end{sphinxVerbatim}
49%|████▉ | 686/1400 [01:18<01:15, 9.48it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 687/1400 [01:18<01:18, 9.10it/s]
</pre>
- 49%|████▉ | 687/1400 [01:18<01:18, 9.10it/s]
end{sphinxVerbatim}
49%|████▉ | 687/1400 [01:18<01:18, 9.10it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 688/1400 [01:19<01:16, 9.32it/s]
</pre>
- 49%|████▉ | 688/1400 [01:19<01:16, 9.32it/s]
end{sphinxVerbatim}
49%|████▉ | 688/1400 [01:19<01:16, 9.32it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 689/1400 [01:19<01:15, 9.38it/s]
</pre>
- 49%|████▉ | 689/1400 [01:19<01:15, 9.38it/s]
end{sphinxVerbatim}
49%|████▉ | 689/1400 [01:19<01:15, 9.38it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 690/1400 [01:19<01:16, 9.26it/s]
</pre>
- 49%|████▉ | 690/1400 [01:19<01:16, 9.26it/s]
end{sphinxVerbatim}
49%|████▉ | 690/1400 [01:19<01:16, 9.26it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 691/1400 [01:19<01:15, 9.39it/s]
</pre>
- 49%|████▉ | 691/1400 [01:19<01:15, 9.39it/s]
end{sphinxVerbatim}
49%|████▉ | 691/1400 [01:19<01:15, 9.39it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 692/1400 [01:19<01:15, 9.42it/s]
</pre>
- 49%|████▉ | 692/1400 [01:19<01:15, 9.42it/s]
end{sphinxVerbatim}
49%|████▉ | 692/1400 [01:19<01:15, 9.42it/s]
- more-to-come:
- class:
stderr
- 50%|████▉ | 693/1400 [01:19<01:14, 9.48it/s]
</pre>
- 50%|████▉ | 693/1400 [01:19<01:14, 9.48it/s]
end{sphinxVerbatim}
50%|████▉ | 693/1400 [01:19<01:14, 9.48it/s]
- more-to-come:
- class:
stderr
- 50%|████▉ | 694/1400 [01:19<01:13, 9.58it/s]
</pre>
- 50%|████▉ | 694/1400 [01:19<01:13, 9.58it/s]
end{sphinxVerbatim}
50%|████▉ | 694/1400 [01:19<01:13, 9.58it/s]
- more-to-come:
- class:
stderr
- 50%|████▉ | 695/1400 [01:19<01:15, 9.32it/s]
</pre>
- 50%|████▉ | 695/1400 [01:19<01:15, 9.32it/s]
end{sphinxVerbatim}
50%|████▉ | 695/1400 [01:19<01:15, 9.32it/s]
- more-to-come:
- class:
stderr
- 50%|████▉ | 696/1400 [01:19<01:15, 9.37it/s]
</pre>
- 50%|████▉ | 696/1400 [01:19<01:15, 9.37it/s]
end{sphinxVerbatim}
50%|████▉ | 696/1400 [01:19<01:15, 9.37it/s]
- more-to-come:
- class:
stderr
- 50%|████▉ | 697/1400 [01:20<01:15, 9.34it/s]
</pre>
- 50%|████▉ | 697/1400 [01:20<01:15, 9.34it/s]
end{sphinxVerbatim}
50%|████▉ | 697/1400 [01:20<01:15, 9.34it/s]
- more-to-come:
- class:
stderr
- 50%|████▉ | 699/1400 [01:20<01:03, 11.11it/s]
</pre>
- 50%|████▉ | 699/1400 [01:20<01:03, 11.11it/s]
end{sphinxVerbatim}
50%|████▉ | 699/1400 [01:20<01:03, 11.11it/s]
- more-to-come:
- class:
stderr
- 50%|█████ | 701/1400 [01:20<00:57, 12.22it/s]
</pre>
- 50%|█████ | 701/1400 [01:20<00:57, 12.22it/s]
end{sphinxVerbatim}
50%|█████ | 701/1400 [01:20<00:57, 12.22it/s]
- more-to-come:
- class:
stderr
- 50%|█████ | 703/1400 [01:20<00:55, 12.57it/s]
</pre>
- 50%|█████ | 703/1400 [01:20<00:55, 12.57it/s]
end{sphinxVerbatim}
50%|█████ | 703/1400 [01:20<00:55, 12.57it/s]
- more-to-come:
- class:
stderr
- 50%|█████ | 705/1400 [01:20<01:04, 10.78it/s]
</pre>
- 50%|█████ | 705/1400 [01:20<01:04, 10.78it/s]
end{sphinxVerbatim}
50%|█████ | 705/1400 [01:20<01:04, 10.78it/s]
- more-to-come:
- class:
stderr
- 50%|█████ | 707/1400 [01:20<01:05, 10.53it/s]
</pre>
- 50%|█████ | 707/1400 [01:20<01:05, 10.53it/s]
end{sphinxVerbatim}
50%|█████ | 707/1400 [01:20<01:05, 10.53it/s]
- more-to-come:
- class:
stderr
- 51%|█████ | 710/1400 [01:21<00:56, 12.26it/s]
</pre>
- 51%|█████ | 710/1400 [01:21<00:56, 12.26it/s]
end{sphinxVerbatim}
51%|█████ | 710/1400 [01:21<00:56, 12.26it/s]
- more-to-come:
- class:
stderr
- 51%|█████ | 712/1400 [01:21<01:00, 11.42it/s]
</pre>
- 51%|█████ | 712/1400 [01:21<01:00, 11.42it/s]
end{sphinxVerbatim}
51%|█████ | 712/1400 [01:21<01:00, 11.42it/s]
- more-to-come:
- class:
stderr
- 51%|█████ | 714/1400 [01:21<01:03, 10.84it/s]
</pre>
- 51%|█████ | 714/1400 [01:21<01:03, 10.84it/s]
end{sphinxVerbatim}
51%|█████ | 714/1400 [01:21<01:03, 10.84it/s]
- more-to-come:
- class:
stderr
- 51%|█████ | 716/1400 [01:21<00:58, 11.74it/s]
</pre>
- 51%|█████ | 716/1400 [01:21<00:58, 11.74it/s]
end{sphinxVerbatim}
51%|█████ | 716/1400 [01:21<00:58, 11.74it/s]
- more-to-come:
- class:
stderr
- 51%|█████▏ | 718/1400 [01:21<01:01, 11.00it/s]
</pre>
- 51%|█████▏ | 718/1400 [01:21<01:01, 11.00it/s]
end{sphinxVerbatim}
51%|█████▏ | 718/1400 [01:21<01:01, 11.00it/s]
- more-to-come:
- class:
stderr
- 51%|█████▏ | 720/1400 [01:21<00:57, 11.89it/s]
</pre>
- 51%|█████▏ | 720/1400 [01:21<00:57, 11.89it/s]
end{sphinxVerbatim}
51%|█████▏ | 720/1400 [01:21<00:57, 11.89it/s]
- more-to-come:
- class:
stderr
- 52%|█████▏ | 723/1400 [01:22<00:50, 13.33it/s]
</pre>
- 52%|█████▏ | 723/1400 [01:22<00:50, 13.33it/s]
end{sphinxVerbatim}
52%|█████▏ | 723/1400 [01:22<00:50, 13.33it/s]
- more-to-come:
- class:
stderr
- 52%|█████▏ | 725/1400 [01:22<00:56, 11.97it/s]
</pre>
- 52%|█████▏ | 725/1400 [01:22<00:56, 11.97it/s]
end{sphinxVerbatim}
52%|█████▏ | 725/1400 [01:22<00:56, 11.97it/s]
- more-to-come:
- class:
stderr
- 52%|█████▏ | 727/1400 [01:22<00:54, 12.45it/s]
</pre>
- 52%|█████▏ | 727/1400 [01:22<00:54, 12.45it/s]
end{sphinxVerbatim}
52%|█████▏ | 727/1400 [01:22<00:54, 12.45it/s]
- more-to-come:
- class:
stderr
- 52%|█████▏ | 729/1400 [01:22<00:51, 13.09it/s]
</pre>
- 52%|█████▏ | 729/1400 [01:22<00:51, 13.09it/s]
end{sphinxVerbatim}
52%|█████▏ | 729/1400 [01:22<00:51, 13.09it/s]
- more-to-come:
- class:
stderr
- 52%|█████▏ | 731/1400 [01:22<00:56, 11.94it/s]
</pre>
- 52%|█████▏ | 731/1400 [01:22<00:56, 11.94it/s]
end{sphinxVerbatim}
52%|█████▏ | 731/1400 [01:22<00:56, 11.94it/s]
- more-to-come:
- class:
stderr
- 52%|█████▏ | 733/1400 [01:23<01:00, 11.05it/s]
</pre>
- 52%|█████▏ | 733/1400 [01:23<01:00, 11.05it/s]
end{sphinxVerbatim}
52%|█████▏ | 733/1400 [01:23<01:00, 11.05it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 736/1400 [01:23<00:46, 14.29it/s]
</pre>
- 53%|█████▎ | 736/1400 [01:23<00:46, 14.29it/s]
end{sphinxVerbatim}
53%|█████▎ | 736/1400 [01:23<00:46, 14.29it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 738/1400 [01:23<00:45, 14.46it/s]
</pre>
- 53%|█████▎ | 738/1400 [01:23<00:45, 14.46it/s]
end{sphinxVerbatim}
53%|█████▎ | 738/1400 [01:23<00:45, 14.46it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 740/1400 [01:23<00:51, 12.84it/s]
</pre>
- 53%|█████▎ | 740/1400 [01:23<00:51, 12.84it/s]
end{sphinxVerbatim}
53%|█████▎ | 740/1400 [01:23<00:51, 12.84it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 742/1400 [01:23<00:49, 13.17it/s]
</pre>
- 53%|█████▎ | 742/1400 [01:23<00:49, 13.17it/s]
end{sphinxVerbatim}
53%|█████▎ | 742/1400 [01:23<00:49, 13.17it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 744/1400 [01:23<00:54, 12.11it/s]
</pre>
- 53%|█████▎ | 744/1400 [01:23<00:54, 12.11it/s]
end{sphinxVerbatim}
53%|█████▎ | 744/1400 [01:23<00:54, 12.11it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 746/1400 [01:24<00:57, 11.35it/s]
</pre>
- 53%|█████▎ | 746/1400 [01:24<00:57, 11.35it/s]
end{sphinxVerbatim}
53%|█████▎ | 746/1400 [01:24<00:57, 11.35it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 748/1400 [01:24<01:02, 10.49it/s]
</pre>
- 53%|█████▎ | 748/1400 [01:24<01:02, 10.49it/s]
end{sphinxVerbatim}
53%|█████▎ | 748/1400 [01:24<01:02, 10.49it/s]
- more-to-come:
- class:
stderr
- 54%|█████▎ | 750/1400 [01:24<01:04, 10.05it/s]
</pre>
- 54%|█████▎ | 750/1400 [01:24<01:04, 10.05it/s]
end{sphinxVerbatim}
54%|█████▎ | 750/1400 [01:24<01:04, 10.05it/s]
- more-to-come:
- class:
stderr
- 54%|█████▎ | 752/1400 [01:24<00:58, 11.16it/s]
</pre>
- 54%|█████▎ | 752/1400 [01:24<00:58, 11.16it/s]
end{sphinxVerbatim}
54%|█████▎ | 752/1400 [01:24<00:58, 11.16it/s]
- more-to-come:
- class:
stderr
- 54%|█████▍ | 754/1400 [01:24<00:58, 10.96it/s]
</pre>
- 54%|█████▍ | 754/1400 [01:24<00:58, 10.96it/s]
end{sphinxVerbatim}
54%|█████▍ | 754/1400 [01:24<00:58, 10.96it/s]
- more-to-come:
- class:
stderr
- 54%|█████▍ | 756/1400 [01:25<01:03, 10.17it/s]
</pre>
- 54%|█████▍ | 756/1400 [01:25<01:03, 10.17it/s]
end{sphinxVerbatim}
54%|█████▍ | 756/1400 [01:25<01:03, 10.17it/s]
- more-to-come:
- class:
stderr
- 54%|█████▍ | 758/1400 [01:25<01:04, 9.90it/s]
</pre>
- 54%|█████▍ | 758/1400 [01:25<01:04, 9.90it/s]
end{sphinxVerbatim}
54%|█████▍ | 758/1400 [01:25<01:04, 9.90it/s]
- more-to-come:
- class:
stderr
- 54%|█████▍ | 760/1400 [01:25<01:09, 9.27it/s]
</pre>
- 54%|█████▍ | 760/1400 [01:25<01:09, 9.27it/s]
end{sphinxVerbatim}
54%|█████▍ | 760/1400 [01:25<01:09, 9.27it/s]
- more-to-come:
- class:
stderr
- 54%|█████▍ | 761/1400 [01:25<01:09, 9.20it/s]
</pre>
- 54%|█████▍ | 761/1400 [01:25<01:09, 9.20it/s]
end{sphinxVerbatim}
54%|█████▍ | 761/1400 [01:25<01:09, 9.20it/s]
- more-to-come:
- class:
stderr
- 54%|█████▍ | 762/1400 [01:25<01:09, 9.18it/s]
</pre>
- 54%|█████▍ | 762/1400 [01:25<01:09, 9.18it/s]
end{sphinxVerbatim}
54%|█████▍ | 762/1400 [01:25<01:09, 9.18it/s]
- more-to-come:
- class:
stderr
- 55%|█████▍ | 763/1400 [01:25<01:08, 9.27it/s]
</pre>
- 55%|█████▍ | 763/1400 [01:25<01:08, 9.27it/s]
end{sphinxVerbatim}
55%|█████▍ | 763/1400 [01:25<01:08, 9.27it/s]
- more-to-come:
- class:
stderr
- 55%|█████▍ | 764/1400 [01:25<01:08, 9.34it/s]
</pre>
- 55%|█████▍ | 764/1400 [01:25<01:08, 9.34it/s]
end{sphinxVerbatim}
55%|█████▍ | 764/1400 [01:25<01:08, 9.34it/s]
- more-to-come:
- class:
stderr
- 55%|█████▍ | 765/1400 [01:26<01:10, 8.98it/s]
</pre>
- 55%|█████▍ | 765/1400 [01:26<01:10, 8.98it/s]
end{sphinxVerbatim}
55%|█████▍ | 765/1400 [01:26<01:10, 8.98it/s]
- more-to-come:
- class:
stderr
- 55%|█████▍ | 766/1400 [01:26<01:10, 9.03it/s]
</pre>
- 55%|█████▍ | 766/1400 [01:26<01:10, 9.03it/s]
end{sphinxVerbatim}
55%|█████▍ | 766/1400 [01:26<01:10, 9.03it/s]
- more-to-come:
- class:
stderr
- 55%|█████▍ | 767/1400 [01:26<01:09, 9.15it/s]
</pre>
- 55%|█████▍ | 767/1400 [01:26<01:09, 9.15it/s]
end{sphinxVerbatim}
55%|█████▍ | 767/1400 [01:26<01:09, 9.15it/s]
- more-to-come:
- class:
stderr
- 55%|█████▍ | 768/1400 [01:26<01:09, 9.09it/s]
</pre>
- 55%|█████▍ | 768/1400 [01:26<01:09, 9.09it/s]
end{sphinxVerbatim}
55%|█████▍ | 768/1400 [01:26<01:09, 9.09it/s]
- more-to-come:
- class:
stderr
- 55%|█████▍ | 769/1400 [01:26<01:08, 9.16it/s]
</pre>
- 55%|█████▍ | 769/1400 [01:26<01:08, 9.16it/s]
end{sphinxVerbatim}
55%|█████▍ | 769/1400 [01:26<01:08, 9.16it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 770/1400 [01:26<01:12, 8.70it/s]
</pre>
- 55%|█████▌ | 770/1400 [01:26<01:12, 8.70it/s]
end{sphinxVerbatim}
55%|█████▌ | 770/1400 [01:26<01:12, 8.70it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 771/1400 [01:26<01:12, 8.72it/s]
</pre>
- 55%|█████▌ | 771/1400 [01:26<01:12, 8.72it/s]
end{sphinxVerbatim}
55%|█████▌ | 771/1400 [01:26<01:12, 8.72it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 772/1400 [01:26<01:10, 8.97it/s]
</pre>
- 55%|█████▌ | 772/1400 [01:26<01:10, 8.97it/s]
end{sphinxVerbatim}
55%|█████▌ | 772/1400 [01:26<01:10, 8.97it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 773/1400 [01:26<01:10, 8.85it/s]
</pre>
- 55%|█████▌ | 773/1400 [01:26<01:10, 8.85it/s]
end{sphinxVerbatim}
55%|█████▌ | 773/1400 [01:26<01:10, 8.85it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 774/1400 [01:27<01:12, 8.68it/s]
</pre>
- 55%|█████▌ | 774/1400 [01:27<01:12, 8.68it/s]
end{sphinxVerbatim}
55%|█████▌ | 774/1400 [01:27<01:12, 8.68it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 775/1400 [01:27<01:12, 8.62it/s]
</pre>
- 55%|█████▌ | 775/1400 [01:27<01:12, 8.62it/s]
end{sphinxVerbatim}
55%|█████▌ | 775/1400 [01:27<01:12, 8.62it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 776/1400 [01:27<01:09, 8.92it/s]
</pre>
- 55%|█████▌ | 776/1400 [01:27<01:09, 8.92it/s]
end{sphinxVerbatim}
55%|█████▌ | 776/1400 [01:27<01:09, 8.92it/s]
- more-to-come:
- class:
stderr
- 56%|█████▌ | 778/1400 [01:27<01:06, 9.38it/s]
</pre>
- 56%|█████▌ | 778/1400 [01:27<01:06, 9.38it/s]
end{sphinxVerbatim}
56%|█████▌ | 778/1400 [01:27<01:06, 9.38it/s]
- more-to-come:
- class:
stderr
- 56%|█████▌ | 779/1400 [01:27<01:06, 9.33it/s]
</pre>
- 56%|█████▌ | 779/1400 [01:27<01:06, 9.33it/s]
end{sphinxVerbatim}
56%|█████▌ | 779/1400 [01:27<01:06, 9.33it/s]
- more-to-come:
- class:
stderr
- 56%|█████▌ | 780/1400 [01:27<01:05, 9.41it/s]
</pre>
- 56%|█████▌ | 780/1400 [01:27<01:05, 9.41it/s]
end{sphinxVerbatim}
56%|█████▌ | 780/1400 [01:27<01:05, 9.41it/s]
- more-to-come:
- class:
stderr
- 56%|█████▌ | 783/1400 [01:27<00:50, 12.25it/s]
</pre>
- 56%|█████▌ | 783/1400 [01:27<00:50, 12.25it/s]
end{sphinxVerbatim}
56%|█████▌ | 783/1400 [01:27<00:50, 12.25it/s]
- more-to-come:
- class:
stderr
- 56%|█████▌ | 785/1400 [01:28<00:46, 13.12it/s]
</pre>
- 56%|█████▌ | 785/1400 [01:28<00:46, 13.12it/s]
end{sphinxVerbatim}
56%|█████▌ | 785/1400 [01:28<00:46, 13.12it/s]
- more-to-come:
- class:
stderr
- 56%|█████▌ | 787/1400 [01:28<00:50, 12.13it/s]
</pre>
- 56%|█████▌ | 787/1400 [01:28<00:50, 12.13it/s]
end{sphinxVerbatim}
56%|█████▌ | 787/1400 [01:28<00:50, 12.13it/s]
- more-to-come:
- class:
stderr
- 56%|█████▋ | 789/1400 [01:28<00:56, 10.86it/s]
</pre>
- 56%|█████▋ | 789/1400 [01:28<00:56, 10.86it/s]
end{sphinxVerbatim}
56%|█████▋ | 789/1400 [01:28<00:56, 10.86it/s]
- more-to-come:
- class:
stderr
- 56%|█████▋ | 791/1400 [01:28<00:50, 12.10it/s]
</pre>
- 56%|█████▋ | 791/1400 [01:28<00:50, 12.10it/s]
end{sphinxVerbatim}
56%|█████▋ | 791/1400 [01:28<00:50, 12.10it/s]
- more-to-come:
- class:
stderr
- 57%|█████▋ | 793/1400 [01:28<00:53, 11.38it/s]
</pre>
- 57%|█████▋ | 793/1400 [01:28<00:53, 11.38it/s]
end{sphinxVerbatim}
57%|█████▋ | 793/1400 [01:28<00:53, 11.38it/s]
- more-to-come:
- class:
stderr
- 57%|█████▋ | 795/1400 [01:28<00:54, 11.18it/s]
</pre>
- 57%|█████▋ | 795/1400 [01:28<00:54, 11.18it/s]
end{sphinxVerbatim}
57%|█████▋ | 795/1400 [01:28<00:54, 11.18it/s]
- more-to-come:
- class:
stderr
- 57%|█████▋ | 797/1400 [01:29<00:49, 12.11it/s]
</pre>
- 57%|█████▋ | 797/1400 [01:29<00:49, 12.11it/s]
end{sphinxVerbatim}
57%|█████▋ | 797/1400 [01:29<00:49, 12.11it/s]
- more-to-come:
- class:
stderr
- 57%|█████▋ | 799/1400 [01:29<00:54, 10.98it/s]
</pre>
- 57%|█████▋ | 799/1400 [01:29<00:54, 10.98it/s]
end{sphinxVerbatim}
57%|█████▋ | 799/1400 [01:29<00:54, 10.98it/s]
- more-to-come:
- class:
stderr
- 57%|█████▋ | 801/1400 [01:29<00:54, 11.01it/s]
</pre>
- 57%|█████▋ | 801/1400 [01:29<00:54, 11.01it/s]
end{sphinxVerbatim}
57%|█████▋ | 801/1400 [01:29<00:54, 11.01it/s]
- more-to-come:
- class:
stderr
- 57%|█████▋ | 803/1400 [01:29<00:48, 12.39it/s]
</pre>
- 57%|█████▋ | 803/1400 [01:29<00:48, 12.39it/s]
end{sphinxVerbatim}
57%|█████▋ | 803/1400 [01:29<00:48, 12.39it/s]
- more-to-come:
- class:
stderr
- 57%|█████▊ | 805/1400 [01:29<00:45, 13.02it/s]
</pre>
- 57%|█████▊ | 805/1400 [01:29<00:45, 13.02it/s]
end{sphinxVerbatim}
57%|█████▊ | 805/1400 [01:29<00:45, 13.02it/s]
- more-to-come:
- class:
stderr
- 58%|█████▊ | 807/1400 [01:29<00:43, 13.53it/s]
</pre>
- 58%|█████▊ | 807/1400 [01:29<00:43, 13.53it/s]
end{sphinxVerbatim}
58%|█████▊ | 807/1400 [01:29<00:43, 13.53it/s]
- more-to-come:
- class:
stderr
- 58%|█████▊ | 810/1400 [01:30<00:39, 14.89it/s]
</pre>
- 58%|█████▊ | 810/1400 [01:30<00:39, 14.89it/s]
end{sphinxVerbatim}
58%|█████▊ | 810/1400 [01:30<00:39, 14.89it/s]
- more-to-come:
- class:
stderr
- 58%|█████▊ | 813/1400 [01:30<00:35, 16.43it/s]
</pre>
- 58%|█████▊ | 813/1400 [01:30<00:35, 16.43it/s]
end{sphinxVerbatim}
58%|█████▊ | 813/1400 [01:30<00:35, 16.43it/s]
- more-to-come:
- class:
stderr
- 58%|█████▊ | 815/1400 [01:30<00:36, 15.86it/s]
</pre>
- 58%|█████▊ | 815/1400 [01:30<00:36, 15.86it/s]
end{sphinxVerbatim}
58%|█████▊ | 815/1400 [01:30<00:36, 15.86it/s]
- more-to-come:
- class:
stderr
- 58%|█████▊ | 817/1400 [01:30<00:38, 15.25it/s]
</pre>
- 58%|█████▊ | 817/1400 [01:30<00:38, 15.25it/s]
end{sphinxVerbatim}
58%|█████▊ | 817/1400 [01:30<00:38, 15.25it/s]
- more-to-come:
- class:
stderr
- 58%|█████▊ | 819/1400 [01:30<00:36, 15.76it/s]
</pre>
- 58%|█████▊ | 819/1400 [01:30<00:36, 15.76it/s]
end{sphinxVerbatim}
58%|█████▊ | 819/1400 [01:30<00:36, 15.76it/s]
- more-to-come:
- class:
stderr
- 59%|█████▊ | 821/1400 [01:30<00:37, 15.56it/s]
</pre>
- 59%|█████▊ | 821/1400 [01:30<00:37, 15.56it/s]
end{sphinxVerbatim}
59%|█████▊ | 821/1400 [01:30<00:37, 15.56it/s]
- more-to-come:
- class:
stderr
- 59%|█████▉ | 823/1400 [01:30<00:37, 15.29it/s]
</pre>
- 59%|█████▉ | 823/1400 [01:30<00:37, 15.29it/s]
end{sphinxVerbatim}
59%|█████▉ | 823/1400 [01:30<00:37, 15.29it/s]
- more-to-come:
- class:
stderr
- 59%|█████▉ | 825/1400 [01:30<00:36, 15.85it/s]
</pre>
- 59%|█████▉ | 825/1400 [01:30<00:36, 15.85it/s]
end{sphinxVerbatim}
59%|█████▉ | 825/1400 [01:30<00:36, 15.85it/s]
- more-to-come:
- class:
stderr
- 59%|█████▉ | 827/1400 [01:31<00:35, 16.14it/s]
</pre>
- 59%|█████▉ | 827/1400 [01:31<00:35, 16.14it/s]
end{sphinxVerbatim}
59%|█████▉ | 827/1400 [01:31<00:35, 16.14it/s]
- more-to-come:
- class:
stderr
- 59%|█████▉ | 829/1400 [01:31<00:34, 16.50it/s]
</pre>
- 59%|█████▉ | 829/1400 [01:31<00:34, 16.50it/s]
end{sphinxVerbatim}
59%|█████▉ | 829/1400 [01:31<00:34, 16.50it/s]
- more-to-come:
- class:
stderr
- 59%|█████▉ | 831/1400 [01:31<00:35, 15.85it/s]
</pre>
- 59%|█████▉ | 831/1400 [01:31<00:35, 15.85it/s]
end{sphinxVerbatim}
59%|█████▉ | 831/1400 [01:31<00:35, 15.85it/s]
- more-to-come:
- class:
stderr
- 60%|█████▉ | 834/1400 [01:31<00:29, 19.03it/s]
</pre>
- 60%|█████▉ | 834/1400 [01:31<00:29, 19.03it/s]
end{sphinxVerbatim}
60%|█████▉ | 834/1400 [01:31<00:29, 19.03it/s]
- more-to-come:
- class:
stderr
- 60%|█████▉ | 836/1400 [01:31<00:31, 17.74it/s]
</pre>
- 60%|█████▉ | 836/1400 [01:31<00:31, 17.74it/s]
end{sphinxVerbatim}
60%|█████▉ | 836/1400 [01:31<00:31, 17.74it/s]
- more-to-come:
- class:
stderr
- 60%|█████▉ | 838/1400 [01:31<00:37, 15.07it/s]
</pre>
- 60%|█████▉ | 838/1400 [01:31<00:37, 15.07it/s]
end{sphinxVerbatim}
60%|█████▉ | 838/1400 [01:31<00:37, 15.07it/s]
- more-to-come:
- class:
stderr
- 60%|██████ | 840/1400 [01:31<00:37, 15.06it/s]
</pre>
- 60%|██████ | 840/1400 [01:31<00:37, 15.06it/s]
end{sphinxVerbatim}
60%|██████ | 840/1400 [01:31<00:37, 15.06it/s]
- more-to-come:
- class:
stderr
- 60%|██████ | 844/1400 [01:32<00:28, 19.54it/s]
</pre>
- 60%|██████ | 844/1400 [01:32<00:28, 19.54it/s]
end{sphinxVerbatim}
60%|██████ | 844/1400 [01:32<00:28, 19.54it/s]
- more-to-come:
- class:
stderr
- 60%|██████ | 847/1400 [01:32<00:27, 19.93it/s]
</pre>
- 60%|██████ | 847/1400 [01:32<00:27, 19.93it/s]
end{sphinxVerbatim}
60%|██████ | 847/1400 [01:32<00:27, 19.93it/s]
- more-to-come:
- class:
stderr
- 61%|██████ | 850/1400 [01:32<00:27, 20.18it/s]
</pre>
- 61%|██████ | 850/1400 [01:32<00:27, 20.18it/s]
end{sphinxVerbatim}
61%|██████ | 850/1400 [01:32<00:27, 20.18it/s]
- more-to-come:
- class:
stderr
- 61%|██████ | 854/1400 [01:32<00:23, 23.33it/s]
</pre>
- 61%|██████ | 854/1400 [01:32<00:23, 23.33it/s]
end{sphinxVerbatim}
61%|██████ | 854/1400 [01:32<00:23, 23.33it/s]
- more-to-come:
- class:
stderr
- 61%|██████ | 857/1400 [01:32<00:26, 20.73it/s]
</pre>
- 61%|██████ | 857/1400 [01:32<00:26, 20.73it/s]
end{sphinxVerbatim}
61%|██████ | 857/1400 [01:32<00:26, 20.73it/s]
- more-to-come:
- class:
stderr
- 61%|██████▏ | 860/1400 [01:32<00:30, 17.57it/s]
</pre>
- 61%|██████▏ | 860/1400 [01:32<00:30, 17.57it/s]
end{sphinxVerbatim}
61%|██████▏ | 860/1400 [01:32<00:30, 17.57it/s]
- more-to-come:
- class:
stderr
- 62%|██████▏ | 864/1400 [01:33<00:29, 18.45it/s]
</pre>
- 62%|██████▏ | 864/1400 [01:33<00:29, 18.45it/s]
end{sphinxVerbatim}
62%|██████▏ | 864/1400 [01:33<00:29, 18.45it/s]
- more-to-come:
- class:
stderr
- 62%|██████▏ | 866/1400 [01:33<00:31, 16.93it/s]
</pre>
- 62%|██████▏ | 866/1400 [01:33<00:31, 16.93it/s]
end{sphinxVerbatim}
62%|██████▏ | 866/1400 [01:33<00:31, 16.93it/s]
- more-to-come:
- class:
stderr
- 62%|██████▏ | 869/1400 [01:33<00:30, 17.65it/s]
</pre>
- 62%|██████▏ | 869/1400 [01:33<00:30, 17.65it/s]
end{sphinxVerbatim}
62%|██████▏ | 869/1400 [01:33<00:30, 17.65it/s]
- more-to-come:
- class:
stderr
- 62%|██████▏ | 872/1400 [01:33<00:28, 18.40it/s]
</pre>
- 62%|██████▏ | 872/1400 [01:33<00:28, 18.40it/s]
end{sphinxVerbatim}
62%|██████▏ | 872/1400 [01:33<00:28, 18.40it/s]
- more-to-come:
- class:
stderr
- 62%|██████▎ | 875/1400 [01:33<00:28, 18.39it/s]
</pre>
- 62%|██████▎ | 875/1400 [01:33<00:28, 18.39it/s]
end{sphinxVerbatim}
62%|██████▎ | 875/1400 [01:33<00:28, 18.39it/s]
- more-to-come:
- class:
stderr
- 63%|██████▎ | 879/1400 [01:33<00:24, 21.70it/s]
</pre>
- 63%|██████▎ | 879/1400 [01:33<00:24, 21.70it/s]
end{sphinxVerbatim}
63%|██████▎ | 879/1400 [01:33<00:24, 21.70it/s]
- more-to-come:
- class:
stderr
- 63%|██████▎ | 882/1400 [01:33<00:24, 21.52it/s]
</pre>
- 63%|██████▎ | 882/1400 [01:33<00:24, 21.52it/s]
end{sphinxVerbatim}
63%|██████▎ | 882/1400 [01:33<00:24, 21.52it/s]
- more-to-come:
- class:
stderr
- 63%|██████▎ | 885/1400 [01:34<00:25, 20.55it/s]
</pre>
- 63%|██████▎ | 885/1400 [01:34<00:25, 20.55it/s]
end{sphinxVerbatim}
63%|██████▎ | 885/1400 [01:34<00:25, 20.55it/s]
- more-to-come:
- class:
stderr
- 63%|██████▎ | 888/1400 [01:34<00:28, 18.25it/s]
</pre>
- 63%|██████▎ | 888/1400 [01:34<00:28, 18.25it/s]
end{sphinxVerbatim}
63%|██████▎ | 888/1400 [01:34<00:28, 18.25it/s]
- more-to-come:
- class:
stderr
- 64%|██████▎ | 890/1400 [01:34<00:29, 17.26it/s]
</pre>
- 64%|██████▎ | 890/1400 [01:34<00:29, 17.26it/s]
end{sphinxVerbatim}
64%|██████▎ | 890/1400 [01:34<00:29, 17.26it/s]
- more-to-come:
- class:
stderr
- 64%|██████▎ | 892/1400 [01:34<00:30, 16.59it/s]
</pre>
- 64%|██████▎ | 892/1400 [01:34<00:30, 16.59it/s]
end{sphinxVerbatim}
64%|██████▎ | 892/1400 [01:34<00:30, 16.59it/s]
- more-to-come:
- class:
stderr
- 64%|██████▍ | 895/1400 [01:34<00:26, 19.41it/s]
</pre>
- 64%|██████▍ | 895/1400 [01:34<00:26, 19.41it/s]
end{sphinxVerbatim}
64%|██████▍ | 895/1400 [01:34<00:26, 19.41it/s]
- more-to-come:
- class:
stderr
- 64%|██████▍ | 898/1400 [01:34<00:26, 18.69it/s]
</pre>
- 64%|██████▍ | 898/1400 [01:34<00:26, 18.69it/s]
end{sphinxVerbatim}
64%|██████▍ | 898/1400 [01:34<00:26, 18.69it/s]
- more-to-come:
- class:
stderr
- 64%|██████▍ | 900/1400 [01:35<00:28, 17.63it/s]
</pre>
- 64%|██████▍ | 900/1400 [01:35<00:28, 17.63it/s]
end{sphinxVerbatim}
64%|██████▍ | 900/1400 [01:35<00:28, 17.63it/s]
- more-to-come:
- class:
stderr
- 64%|██████▍ | 902/1400 [01:35<00:29, 16.64it/s]
</pre>
- 64%|██████▍ | 902/1400 [01:35<00:29, 16.64it/s]
end{sphinxVerbatim}
64%|██████▍ | 902/1400 [01:35<00:29, 16.64it/s]
- more-to-come:
- class:
stderr
- 65%|██████▍ | 905/1400 [01:35<00:29, 16.98it/s]
</pre>
- 65%|██████▍ | 905/1400 [01:35<00:29, 16.98it/s]
end{sphinxVerbatim}
65%|██████▍ | 905/1400 [01:35<00:29, 16.98it/s]
- more-to-come:
- class:
stderr
- 65%|██████▍ | 909/1400 [01:35<00:23, 20.88it/s]
</pre>
- 65%|██████▍ | 909/1400 [01:35<00:23, 20.88it/s]
end{sphinxVerbatim}
65%|██████▍ | 909/1400 [01:35<00:23, 20.88it/s]
- more-to-come:
- class:
stderr
- 65%|██████▌ | 912/1400 [01:35<00:27, 17.50it/s]
</pre>
- 65%|██████▌ | 912/1400 [01:35<00:27, 17.50it/s]
end{sphinxVerbatim}
65%|██████▌ | 912/1400 [01:35<00:27, 17.50it/s]
- more-to-come:
- class:
stderr
- 65%|██████▌ | 916/1400 [01:35<00:26, 18.54it/s]
</pre>
- 65%|██████▌ | 916/1400 [01:35<00:26, 18.54it/s]
end{sphinxVerbatim}
65%|██████▌ | 916/1400 [01:35<00:26, 18.54it/s]
- more-to-come:
- class:
stderr
- 66%|██████▌ | 919/1400 [01:36<00:23, 20.23it/s]
</pre>
- 66%|██████▌ | 919/1400 [01:36<00:23, 20.23it/s]
end{sphinxVerbatim}
66%|██████▌ | 919/1400 [01:36<00:23, 20.23it/s]
- more-to-come:
- class:
stderr
- 66%|██████▌ | 922/1400 [01:36<00:24, 19.55it/s]
</pre>
- 66%|██████▌ | 922/1400 [01:36<00:24, 19.55it/s]
end{sphinxVerbatim}
66%|██████▌ | 922/1400 [01:36<00:24, 19.55it/s]
- more-to-come:
- class:
stderr
- 66%|██████▌ | 925/1400 [01:36<00:24, 19.13it/s]
</pre>
- 66%|██████▌ | 925/1400 [01:36<00:24, 19.13it/s]
end{sphinxVerbatim}
66%|██████▌ | 925/1400 [01:36<00:24, 19.13it/s]
- more-to-come:
- class:
stderr
- 66%|██████▌ | 927/1400 [01:36<00:26, 17.84it/s]
</pre>
- 66%|██████▌ | 927/1400 [01:36<00:26, 17.84it/s]
end{sphinxVerbatim}
66%|██████▌ | 927/1400 [01:36<00:26, 17.84it/s]
- more-to-come:
- class:
stderr
- 66%|██████▋ | 930/1400 [01:36<00:26, 17.69it/s]
</pre>
- 66%|██████▋ | 930/1400 [01:36<00:26, 17.69it/s]
end{sphinxVerbatim}
66%|██████▋ | 930/1400 [01:36<00:26, 17.69it/s]
- more-to-come:
- class:
stderr
- 67%|██████▋ | 932/1400 [01:36<00:27, 16.92it/s]
</pre>
- 67%|██████▋ | 932/1400 [01:36<00:27, 16.92it/s]
end{sphinxVerbatim}
67%|██████▋ | 932/1400 [01:36<00:27, 16.92it/s]
- more-to-come:
- class:
stderr
- 67%|██████▋ | 934/1400 [01:36<00:27, 16.98it/s]
</pre>
- 67%|██████▋ | 934/1400 [01:36<00:27, 16.98it/s]
end{sphinxVerbatim}
67%|██████▋ | 934/1400 [01:36<00:27, 16.98it/s]
- more-to-come:
- class:
stderr
- 67%|██████▋ | 936/1400 [01:37<00:26, 17.29it/s]
</pre>
- 67%|██████▋ | 936/1400 [01:37<00:26, 17.29it/s]
end{sphinxVerbatim}
67%|██████▋ | 936/1400 [01:37<00:26, 17.29it/s]
- more-to-come:
- class:
stderr
- 67%|██████▋ | 939/1400 [01:37<00:26, 17.44it/s]
</pre>
- 67%|██████▋ | 939/1400 [01:37<00:26, 17.44it/s]
end{sphinxVerbatim}
67%|██████▋ | 939/1400 [01:37<00:26, 17.44it/s]
- more-to-come:
- class:
stderr
- 67%|██████▋ | 942/1400 [01:37<00:22, 20.32it/s]
</pre>
- 67%|██████▋ | 942/1400 [01:37<00:22, 20.32it/s]
end{sphinxVerbatim}
67%|██████▋ | 942/1400 [01:37<00:22, 20.32it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 945/1400 [01:37<00:24, 18.71it/s]
</pre>
- 68%|██████▊ | 945/1400 [01:37<00:24, 18.71it/s]
end{sphinxVerbatim}
68%|██████▊ | 945/1400 [01:37<00:24, 18.71it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 947/1400 [01:37<00:26, 16.99it/s]
</pre>
- 68%|██████▊ | 947/1400 [01:37<00:26, 16.99it/s]
end{sphinxVerbatim}
68%|██████▊ | 947/1400 [01:37<00:26, 16.99it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 949/1400 [01:37<00:27, 16.44it/s]
</pre>
- 68%|██████▊ | 949/1400 [01:37<00:27, 16.44it/s]
end{sphinxVerbatim}
68%|██████▊ | 949/1400 [01:37<00:27, 16.44it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 952/1400 [01:37<00:22, 19.54it/s]
</pre>
- 68%|██████▊ | 952/1400 [01:37<00:22, 19.54it/s]
end{sphinxVerbatim}
68%|██████▊ | 952/1400 [01:37<00:22, 19.54it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 955/1400 [01:38<00:23, 18.64it/s]
</pre>
- 68%|██████▊ | 955/1400 [01:38<00:23, 18.64it/s]
end{sphinxVerbatim}
68%|██████▊ | 955/1400 [01:38<00:23, 18.64it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 958/1400 [01:38<00:20, 21.23it/s]
</pre>
- 68%|██████▊ | 958/1400 [01:38<00:20, 21.23it/s]
end{sphinxVerbatim}
68%|██████▊ | 958/1400 [01:38<00:20, 21.23it/s]
- more-to-come:
- class:
stderr
- 69%|██████▊ | 961/1400 [01:38<00:24, 17.69it/s]
</pre>
- 69%|██████▊ | 961/1400 [01:38<00:24, 17.69it/s]
end{sphinxVerbatim}
69%|██████▊ | 961/1400 [01:38<00:24, 17.69it/s]
- more-to-come:
- class:
stderr
- 69%|██████▉ | 965/1400 [01:38<00:20, 21.14it/s]
</pre>
- 69%|██████▉ | 965/1400 [01:38<00:20, 21.14it/s]
end{sphinxVerbatim}
69%|██████▉ | 965/1400 [01:38<00:20, 21.14it/s]
- more-to-come:
- class:
stderr
- 69%|██████▉ | 968/1400 [01:38<00:21, 19.79it/s]
</pre>
- 69%|██████▉ | 968/1400 [01:38<00:21, 19.79it/s]
end{sphinxVerbatim}
69%|██████▉ | 968/1400 [01:38<00:21, 19.79it/s]
- more-to-come:
- class:
stderr
- 69%|██████▉ | 971/1400 [01:38<00:22, 19.20it/s]
</pre>
- 69%|██████▉ | 971/1400 [01:38<00:22, 19.20it/s]
end{sphinxVerbatim}
69%|██████▉ | 971/1400 [01:38<00:22, 19.20it/s]
- more-to-come:
- class:
stderr
- 70%|██████▉ | 974/1400 [01:38<00:21, 19.66it/s]
</pre>
- 70%|██████▉ | 974/1400 [01:38<00:21, 19.66it/s]
end{sphinxVerbatim}
70%|██████▉ | 974/1400 [01:38<00:21, 19.66it/s]
- more-to-come:
- class:
stderr
- 70%|██████▉ | 977/1400 [01:39<00:22, 19.06it/s]
</pre>
- 70%|██████▉ | 977/1400 [01:39<00:22, 19.06it/s]
end{sphinxVerbatim}
70%|██████▉ | 977/1400 [01:39<00:22, 19.06it/s]
- more-to-come:
- class:
stderr
- 70%|███████ | 981/1400 [01:39<00:18, 22.17it/s]
</pre>
- 70%|███████ | 981/1400 [01:39<00:18, 22.17it/s]
end{sphinxVerbatim}
70%|███████ | 981/1400 [01:39<00:18, 22.17it/s]
- more-to-come:
- class:
stderr
- 70%|███████ | 984/1400 [01:39<00:20, 20.61it/s]
</pre>
- 70%|███████ | 984/1400 [01:39<00:20, 20.61it/s]
end{sphinxVerbatim}
70%|███████ | 984/1400 [01:39<00:20, 20.61it/s]
- more-to-come:
- class:
stderr
- 70%|███████ | 987/1400 [01:39<00:18, 21.94it/s]
</pre>
- 70%|███████ | 987/1400 [01:39<00:18, 21.94it/s]
end{sphinxVerbatim}
70%|███████ | 987/1400 [01:39<00:18, 21.94it/s]
- more-to-come:
- class:
stderr
- 71%|███████ | 990/1400 [01:39<00:20, 19.98it/s]
</pre>
- 71%|███████ | 990/1400 [01:39<00:20, 19.98it/s]
end{sphinxVerbatim}
71%|███████ | 990/1400 [01:39<00:20, 19.98it/s]
- more-to-come:
- class:
stderr
- 71%|███████ | 993/1400 [01:39<00:18, 21.98it/s]
</pre>
- 71%|███████ | 993/1400 [01:39<00:18, 21.98it/s]
end{sphinxVerbatim}
71%|███████ | 993/1400 [01:39<00:18, 21.98it/s]
- more-to-come:
- class:
stderr
- 71%|███████ | 996/1400 [01:39<00:17, 23.58it/s]
</pre>
- 71%|███████ | 996/1400 [01:39<00:17, 23.58it/s]
end{sphinxVerbatim}
71%|███████ | 996/1400 [01:39<00:17, 23.58it/s]
- more-to-come:
- class:
stderr
- 71%|███████▏ | 999/1400 [01:40<00:15, 25.14it/s]
</pre>
- 71%|███████▏ | 999/1400 [01:40<00:15, 25.14it/s]
end{sphinxVerbatim}
71%|███████▏ | 999/1400 [01:40<00:15, 25.14it/s]
- more-to-come:
- class:
stderr
- 72%|███████▏ | 1002/1400 [01:40<00:17, 23.23it/s]
</pre>
- 72%|███████▏ | 1002/1400 [01:40<00:17, 23.23it/s]
end{sphinxVerbatim}
72%|███████▏ | 1002/1400 [01:40<00:17, 23.23it/s]
- more-to-come:
- class:
stderr
- 72%|███████▏ | 1005/1400 [01:40<00:16, 24.66it/s]
</pre>
- 72%|███████▏ | 1005/1400 [01:40<00:16, 24.66it/s]
end{sphinxVerbatim}
72%|███████▏ | 1005/1400 [01:40<00:16, 24.66it/s]
- more-to-come:
- class:
stderr
- 72%|███████▏ | 1008/1400 [01:40<00:18, 21.71it/s]
</pre>
- 72%|███████▏ | 1008/1400 [01:40<00:18, 21.71it/s]
end{sphinxVerbatim}
72%|███████▏ | 1008/1400 [01:40<00:18, 21.71it/s]
- more-to-come:
- class:
stderr
- 72%|███████▏ | 1011/1400 [01:40<00:17, 22.52it/s]
</pre>
- 72%|███████▏ | 1011/1400 [01:40<00:17, 22.52it/s]
end{sphinxVerbatim}
72%|███████▏ | 1011/1400 [01:40<00:17, 22.52it/s]
- more-to-come:
- class:
stderr
- 72%|███████▏ | 1014/1400 [01:40<00:16, 23.80it/s]
</pre>
- 72%|███████▏ | 1014/1400 [01:40<00:16, 23.80it/s]
end{sphinxVerbatim}
72%|███████▏ | 1014/1400 [01:40<00:16, 23.80it/s]
- more-to-come:
- class:
stderr
- 73%|███████▎ | 1018/1400 [01:40<00:16, 23.06it/s]
</pre>
- 73%|███████▎ | 1018/1400 [01:40<00:16, 23.06it/s]
end{sphinxVerbatim}
73%|███████▎ | 1018/1400 [01:40<00:16, 23.06it/s]
- more-to-come:
- class:
stderr
- 73%|███████▎ | 1021/1400 [01:41<00:20, 18.30it/s]
</pre>
- 73%|███████▎ | 1021/1400 [01:41<00:20, 18.30it/s]
end{sphinxVerbatim}
73%|███████▎ | 1021/1400 [01:41<00:20, 18.30it/s]
- more-to-come:
- class:
stderr
- 73%|███████▎ | 1024/1400 [01:41<00:21, 17.60it/s]
</pre>
- 73%|███████▎ | 1024/1400 [01:41<00:21, 17.60it/s]
end{sphinxVerbatim}
73%|███████▎ | 1024/1400 [01:41<00:21, 17.60it/s]
- more-to-come:
- class:
stderr
- 73%|███████▎ | 1027/1400 [01:41<00:18, 19.76it/s]
</pre>
- 73%|███████▎ | 1027/1400 [01:41<00:18, 19.76it/s]
end{sphinxVerbatim}
73%|███████▎ | 1027/1400 [01:41<00:18, 19.76it/s]
- more-to-come:
- class:
stderr
- 74%|███████▎ | 1030/1400 [01:41<00:16, 21.87it/s]
</pre>
- 74%|███████▎ | 1030/1400 [01:41<00:16, 21.87it/s]
end{sphinxVerbatim}
74%|███████▎ | 1030/1400 [01:41<00:16, 21.87it/s]
- more-to-come:
- class:
stderr
- 74%|███████▍ | 1033/1400 [01:41<00:15, 23.55it/s]
</pre>
- 74%|███████▍ | 1033/1400 [01:41<00:15, 23.55it/s]
end{sphinxVerbatim}
74%|███████▍ | 1033/1400 [01:41<00:15, 23.55it/s]
- more-to-come:
- class:
stderr
- 74%|███████▍ | 1036/1400 [01:41<00:17, 20.88it/s]
</pre>
- 74%|███████▍ | 1036/1400 [01:41<00:17, 20.88it/s]
end{sphinxVerbatim}
74%|███████▍ | 1036/1400 [01:41<00:17, 20.88it/s]
- more-to-come:
- class:
stderr
- 74%|███████▍ | 1040/1400 [01:41<00:15, 23.15it/s]
</pre>
- 74%|███████▍ | 1040/1400 [01:41<00:15, 23.15it/s]
end{sphinxVerbatim}
74%|███████▍ | 1040/1400 [01:41<00:15, 23.15it/s]
- more-to-come:
- class:
stderr
- 74%|███████▍ | 1043/1400 [01:42<00:17, 20.22it/s]
</pre>
- 74%|███████▍ | 1043/1400 [01:42<00:17, 20.22it/s]
end{sphinxVerbatim}
74%|███████▍ | 1043/1400 [01:42<00:17, 20.22it/s]
- more-to-come:
- class:
stderr
- 75%|███████▍ | 1046/1400 [01:42<00:18, 19.10it/s]
</pre>
- 75%|███████▍ | 1046/1400 [01:42<00:18, 19.10it/s]
end{sphinxVerbatim}
75%|███████▍ | 1046/1400 [01:42<00:18, 19.10it/s]
- more-to-come:
- class:
stderr
- 75%|███████▍ | 1049/1400 [01:42<00:18, 19.27it/s]
</pre>
- 75%|███████▍ | 1049/1400 [01:42<00:18, 19.27it/s]
end{sphinxVerbatim}
75%|███████▍ | 1049/1400 [01:42<00:18, 19.27it/s]
- more-to-come:
- class:
stderr
- 75%|███████▌ | 1052/1400 [01:42<00:19, 18.06it/s]
</pre>
- 75%|███████▌ | 1052/1400 [01:42<00:19, 18.06it/s]
end{sphinxVerbatim}
75%|███████▌ | 1052/1400 [01:42<00:19, 18.06it/s]
- more-to-come:
- class:
stderr
- 75%|███████▌ | 1054/1400 [01:42<00:22, 15.46it/s]
</pre>
- 75%|███████▌ | 1054/1400 [01:42<00:22, 15.46it/s]
end{sphinxVerbatim}
75%|███████▌ | 1054/1400 [01:42<00:22, 15.46it/s]
- more-to-come:
- class:
stderr
- 75%|███████▌ | 1056/1400 [01:43<00:22, 15.04it/s]
</pre>
- 75%|███████▌ | 1056/1400 [01:43<00:22, 15.04it/s]
end{sphinxVerbatim}
75%|███████▌ | 1056/1400 [01:43<00:22, 15.04it/s]
- more-to-come:
- class:
stderr
- 76%|███████▌ | 1060/1400 [01:43<00:18, 18.82it/s]
</pre>
- 76%|███████▌ | 1060/1400 [01:43<00:18, 18.82it/s]
end{sphinxVerbatim}
76%|███████▌ | 1060/1400 [01:43<00:18, 18.82it/s]
- more-to-come:
- class:
stderr
- 76%|███████▌ | 1063/1400 [01:43<00:15, 21.17it/s]
</pre>
- 76%|███████▌ | 1063/1400 [01:43<00:15, 21.17it/s]
end{sphinxVerbatim}
76%|███████▌ | 1063/1400 [01:43<00:15, 21.17it/s]
- more-to-come:
- class:
stderr
- 76%|███████▌ | 1067/1400 [01:43<00:14, 23.48it/s]
</pre>
- 76%|███████▌ | 1067/1400 [01:43<00:14, 23.48it/s]
end{sphinxVerbatim}
76%|███████▌ | 1067/1400 [01:43<00:14, 23.48it/s]
- more-to-come:
- class:
stderr
- 76%|███████▋ | 1070/1400 [01:43<00:15, 21.79it/s]
</pre>
- 76%|███████▋ | 1070/1400 [01:43<00:15, 21.79it/s]
end{sphinxVerbatim}
76%|███████▋ | 1070/1400 [01:43<00:15, 21.79it/s]
- more-to-come:
- class:
stderr
- 77%|███████▋ | 1073/1400 [01:43<00:15, 20.60it/s]
</pre>
- 77%|███████▋ | 1073/1400 [01:43<00:15, 20.60it/s]
end{sphinxVerbatim}
77%|███████▋ | 1073/1400 [01:43<00:15, 20.60it/s]
- more-to-come:
- class:
stderr
- 77%|███████▋ | 1076/1400 [01:43<00:16, 19.77it/s]
</pre>
- 77%|███████▋ | 1076/1400 [01:43<00:16, 19.77it/s]
end{sphinxVerbatim}
77%|███████▋ | 1076/1400 [01:43<00:16, 19.77it/s]
- more-to-come:
- class:
stderr
- 77%|███████▋ | 1079/1400 [01:44<00:14, 21.61it/s]
</pre>
- 77%|███████▋ | 1079/1400 [01:44<00:14, 21.61it/s]
end{sphinxVerbatim}
77%|███████▋ | 1079/1400 [01:44<00:14, 21.61it/s]
- more-to-come:
- class:
stderr
- 77%|███████▋ | 1082/1400 [01:44<00:16, 19.77it/s]
</pre>
- 77%|███████▋ | 1082/1400 [01:44<00:16, 19.77it/s]
end{sphinxVerbatim}
77%|███████▋ | 1082/1400 [01:44<00:16, 19.77it/s]
- more-to-come:
- class:
stderr
- 78%|███████▊ | 1085/1400 [01:44<00:17, 18.43it/s]
</pre>
- 78%|███████▊ | 1085/1400 [01:44<00:17, 18.43it/s]
end{sphinxVerbatim}
78%|███████▊ | 1085/1400 [01:44<00:17, 18.43it/s]
- more-to-come:
- class:
stderr
- 78%|███████▊ | 1087/1400 [01:44<00:17, 17.48it/s]
</pre>
- 78%|███████▊ | 1087/1400 [01:44<00:17, 17.48it/s]
end{sphinxVerbatim}
78%|███████▊ | 1087/1400 [01:44<00:17, 17.48it/s]
- more-to-come:
- class:
stderr
- 78%|███████▊ | 1091/1400 [01:44<00:17, 18.09it/s]
</pre>
- 78%|███████▊ | 1091/1400 [01:44<00:17, 18.09it/s]
end{sphinxVerbatim}
78%|███████▊ | 1091/1400 [01:44<00:17, 18.09it/s]
- more-to-come:
- class:
stderr
- 78%|███████▊ | 1094/1400 [01:44<00:16, 18.66it/s]
</pre>
- 78%|███████▊ | 1094/1400 [01:44<00:16, 18.66it/s]
end{sphinxVerbatim}
78%|███████▊ | 1094/1400 [01:44<00:16, 18.66it/s]
- more-to-come:
- class:
stderr
- 78%|███████▊ | 1096/1400 [01:45<00:17, 17.63it/s]
</pre>
- 78%|███████▊ | 1096/1400 [01:45<00:17, 17.63it/s]
end{sphinxVerbatim}
78%|███████▊ | 1096/1400 [01:45<00:17, 17.63it/s]
- more-to-come:
- class:
stderr
- 79%|███████▊ | 1100/1400 [01:45<00:16, 18.67it/s]
</pre>
- 79%|███████▊ | 1100/1400 [01:45<00:16, 18.67it/s]
end{sphinxVerbatim}
79%|███████▊ | 1100/1400 [01:45<00:16, 18.67it/s]
- more-to-come:
- class:
stderr
- 79%|███████▉ | 1104/1400 [01:45<00:13, 21.77it/s]
</pre>
- 79%|███████▉ | 1104/1400 [01:45<00:13, 21.77it/s]
end{sphinxVerbatim}
79%|███████▉ | 1104/1400 [01:45<00:13, 21.77it/s]
- more-to-come:
- class:
stderr
- 79%|███████▉ | 1107/1400 [01:45<00:13, 21.21it/s]
</pre>
- 79%|███████▉ | 1107/1400 [01:45<00:13, 21.21it/s]
end{sphinxVerbatim}
79%|███████▉ | 1107/1400 [01:45<00:13, 21.21it/s]
- more-to-come:
- class:
stderr
- 79%|███████▉ | 1110/1400 [01:45<00:12, 23.06it/s]
</pre>
- 79%|███████▉ | 1110/1400 [01:45<00:12, 23.06it/s]
end{sphinxVerbatim}
79%|███████▉ | 1110/1400 [01:45<00:12, 23.06it/s]
- more-to-come:
- class:
stderr
- 80%|███████▉ | 1113/1400 [01:45<00:11, 24.65it/s]
</pre>
- 80%|███████▉ | 1113/1400 [01:45<00:11, 24.65it/s]
end{sphinxVerbatim}
80%|███████▉ | 1113/1400 [01:45<00:11, 24.65it/s]
- more-to-come:
- class:
stderr
- 80%|███████▉ | 1117/1400 [01:45<00:10, 26.43it/s]
</pre>
- 80%|███████▉ | 1117/1400 [01:45<00:10, 26.43it/s]
end{sphinxVerbatim}
80%|███████▉ | 1117/1400 [01:45<00:10, 26.43it/s]
- more-to-come:
- class:
stderr
- 80%|████████ | 1120/1400 [01:45<00:11, 23.48it/s]
</pre>
- 80%|████████ | 1120/1400 [01:45<00:11, 23.48it/s]
end{sphinxVerbatim}
80%|████████ | 1120/1400 [01:45<00:11, 23.48it/s]
- more-to-come:
- class:
stderr
- 80%|████████ | 1123/1400 [01:46<00:13, 20.99it/s]
</pre>
- 80%|████████ | 1123/1400 [01:46<00:13, 20.99it/s]
end{sphinxVerbatim}
80%|████████ | 1123/1400 [01:46<00:13, 20.99it/s]
- more-to-come:
- class:
stderr
- 80%|████████ | 1126/1400 [01:46<00:13, 20.66it/s]
</pre>
- 80%|████████ | 1126/1400 [01:46<00:13, 20.66it/s]
end{sphinxVerbatim}
80%|████████ | 1126/1400 [01:46<00:13, 20.66it/s]
- more-to-come:
- class:
stderr
- 81%|████████ | 1129/1400 [01:46<00:11, 22.68it/s]
</pre>
- 81%|████████ | 1129/1400 [01:46<00:11, 22.68it/s]
end{sphinxVerbatim}
81%|████████ | 1129/1400 [01:46<00:11, 22.68it/s]
- more-to-come:
- class:
stderr
- 81%|████████ | 1132/1400 [01:46<00:11, 24.21it/s]
</pre>
- 81%|████████ | 1132/1400 [01:46<00:11, 24.21it/s]
end{sphinxVerbatim}
81%|████████ | 1132/1400 [01:46<00:11, 24.21it/s]
- more-to-come:
- class:
stderr
- 81%|████████ | 1136/1400 [01:46<00:10, 25.81it/s]
</pre>
- 81%|████████ | 1136/1400 [01:46<00:10, 25.81it/s]
end{sphinxVerbatim}
81%|████████ | 1136/1400 [01:46<00:10, 25.81it/s]
- more-to-come:
- class:
stderr
- 81%|████████▏ | 1140/1400 [01:46<00:10, 23.70it/s]
</pre>
- 81%|████████▏ | 1140/1400 [01:46<00:10, 23.70it/s]
end{sphinxVerbatim}
81%|████████▏ | 1140/1400 [01:46<00:10, 23.70it/s]
- more-to-come:
- class:
stderr
- 82%|████████▏ | 1143/1400 [01:46<00:10, 24.74it/s]
</pre>
- 82%|████████▏ | 1143/1400 [01:46<00:10, 24.74it/s]
end{sphinxVerbatim}
82%|████████▏ | 1143/1400 [01:46<00:10, 24.74it/s]
- more-to-come:
- class:
stderr
- 82%|████████▏ | 1147/1400 [01:47<00:09, 26.45it/s]
</pre>
- 82%|████████▏ | 1147/1400 [01:47<00:09, 26.45it/s]
end{sphinxVerbatim}
82%|████████▏ | 1147/1400 [01:47<00:09, 26.45it/s]
- more-to-come:
- class:
stderr
- 82%|████████▏ | 1150/1400 [01:47<00:10, 23.21it/s]
</pre>
- 82%|████████▏ | 1150/1400 [01:47<00:10, 23.21it/s]
end{sphinxVerbatim}
82%|████████▏ | 1150/1400 [01:47<00:10, 23.21it/s]
- more-to-come:
- class:
stderr
- 82%|████████▏ | 1154/1400 [01:47<00:09, 25.39it/s]
</pre>
- 82%|████████▏ | 1154/1400 [01:47<00:09, 25.39it/s]
end{sphinxVerbatim}
82%|████████▏ | 1154/1400 [01:47<00:09, 25.39it/s]
- more-to-come:
- class:
stderr
- 83%|████████▎ | 1157/1400 [01:47<00:11, 20.62it/s]
</pre>
- 83%|████████▎ | 1157/1400 [01:47<00:11, 20.62it/s]
end{sphinxVerbatim}
83%|████████▎ | 1157/1400 [01:47<00:11, 20.62it/s]
- more-to-come:
- class:
stderr
- 83%|████████▎ | 1160/1400 [01:47<00:10, 22.10it/s]
</pre>
- 83%|████████▎ | 1160/1400 [01:47<00:10, 22.10it/s]
end{sphinxVerbatim}
83%|████████▎ | 1160/1400 [01:47<00:10, 22.10it/s]
- more-to-come:
- class:
stderr
- 83%|████████▎ | 1163/1400 [01:47<00:10, 23.45it/s]
</pre>
- 83%|████████▎ | 1163/1400 [01:47<00:10, 23.45it/s]
end{sphinxVerbatim}
83%|████████▎ | 1163/1400 [01:47<00:10, 23.45it/s]
- more-to-come:
- class:
stderr
- 83%|████████▎ | 1166/1400 [01:47<00:10, 21.77it/s]
</pre>
- 83%|████████▎ | 1166/1400 [01:47<00:10, 21.77it/s]
end{sphinxVerbatim}
83%|████████▎ | 1166/1400 [01:47<00:10, 21.77it/s]
- more-to-come:
- class:
stderr
- 84%|████████▎ | 1170/1400 [01:48<00:09, 24.41it/s]
</pre>
- 84%|████████▎ | 1170/1400 [01:48<00:09, 24.41it/s]
end{sphinxVerbatim}
84%|████████▎ | 1170/1400 [01:48<00:09, 24.41it/s]
- more-to-come:
- class:
stderr
- 84%|████████▍ | 1173/1400 [01:48<00:10, 22.34it/s]
</pre>
- 84%|████████▍ | 1173/1400 [01:48<00:10, 22.34it/s]
end{sphinxVerbatim}
84%|████████▍ | 1173/1400 [01:48<00:10, 22.34it/s]
- more-to-come:
- class:
stderr
- 84%|████████▍ | 1176/1400 [01:48<00:10, 20.89it/s]
</pre>
- 84%|████████▍ | 1176/1400 [01:48<00:10, 20.89it/s]
end{sphinxVerbatim}
84%|████████▍ | 1176/1400 [01:48<00:10, 20.89it/s]
- more-to-come:
- class:
stderr
- 84%|████████▍ | 1180/1400 [01:48<00:09, 23.48it/s]
</pre>
- 84%|████████▍ | 1180/1400 [01:48<00:09, 23.48it/s]
end{sphinxVerbatim}
84%|████████▍ | 1180/1400 [01:48<00:09, 23.48it/s]
- more-to-come:
- class:
stderr
- 85%|████████▍ | 1184/1400 [01:48<00:08, 25.54it/s]
</pre>
- 85%|████████▍ | 1184/1400 [01:48<00:08, 25.54it/s]
end{sphinxVerbatim}
85%|████████▍ | 1184/1400 [01:48<00:08, 25.54it/s]
- more-to-come:
- class:
stderr
- 85%|████████▍ | 1187/1400 [01:48<00:09, 23.65it/s]
</pre>
- 85%|████████▍ | 1187/1400 [01:48<00:09, 23.65it/s]
end{sphinxVerbatim}
85%|████████▍ | 1187/1400 [01:48<00:09, 23.65it/s]
- more-to-come:
- class:
stderr
- 85%|████████▌ | 1190/1400 [01:49<00:09, 21.15it/s]
</pre>
- 85%|████████▌ | 1190/1400 [01:49<00:09, 21.15it/s]
end{sphinxVerbatim}
85%|████████▌ | 1190/1400 [01:49<00:09, 21.15it/s]
- more-to-come:
- class:
stderr
- 85%|████████▌ | 1194/1400 [01:49<00:08, 23.62it/s]
</pre>
- 85%|████████▌ | 1194/1400 [01:49<00:08, 23.62it/s]
end{sphinxVerbatim}
85%|████████▌ | 1194/1400 [01:49<00:08, 23.62it/s]
- more-to-come:
- class:
stderr
- 86%|████████▌ | 1197/1400 [01:49<00:09, 20.90it/s]
</pre>
- 86%|████████▌ | 1197/1400 [01:49<00:09, 20.90it/s]
end{sphinxVerbatim}
86%|████████▌ | 1197/1400 [01:49<00:09, 20.90it/s]
- more-to-come:
- class:
stderr
- 86%|████████▌ | 1200/1400 [01:49<00:11, 17.23it/s]
</pre>
- 86%|████████▌ | 1200/1400 [01:49<00:11, 17.23it/s]
end{sphinxVerbatim}
86%|████████▌ | 1200/1400 [01:49<00:11, 17.23it/s]
- more-to-come:
- class:
stderr
- 86%|████████▌ | 1204/1400 [01:49<00:09, 20.28it/s]
</pre>
- 86%|████████▌ | 1204/1400 [01:49<00:09, 20.28it/s]
end{sphinxVerbatim}
86%|████████▌ | 1204/1400 [01:49<00:09, 20.28it/s]
- more-to-come:
- class:
stderr
- 86%|████████▌ | 1207/1400 [01:49<00:08, 22.15it/s]
</pre>
- 86%|████████▌ | 1207/1400 [01:49<00:08, 22.15it/s]
end{sphinxVerbatim}
86%|████████▌ | 1207/1400 [01:49<00:08, 22.15it/s]
- more-to-come:
- class:
stderr
- 86%|████████▋ | 1210/1400 [01:49<00:07, 23.86it/s]
</pre>
- 86%|████████▋ | 1210/1400 [01:49<00:07, 23.86it/s]
end{sphinxVerbatim}
86%|████████▋ | 1210/1400 [01:49<00:07, 23.86it/s]
- more-to-come:
- class:
stderr
- 87%|████████▋ | 1213/1400 [01:50<00:09, 18.86it/s]
</pre>
- 87%|████████▋ | 1213/1400 [01:50<00:09, 18.86it/s]
end{sphinxVerbatim}
87%|████████▋ | 1213/1400 [01:50<00:09, 18.86it/s]
- more-to-come:
- class:
stderr
- 87%|████████▋ | 1216/1400 [01:50<00:09, 19.00it/s]
</pre>
- 87%|████████▋ | 1216/1400 [01:50<00:09, 19.00it/s]
end{sphinxVerbatim}
87%|████████▋ | 1216/1400 [01:50<00:09, 19.00it/s]
- more-to-come:
- class:
stderr
- 87%|████████▋ | 1220/1400 [01:50<00:09, 19.33it/s]
</pre>
- 87%|████████▋ | 1220/1400 [01:50<00:09, 19.33it/s]
end{sphinxVerbatim}
87%|████████▋ | 1220/1400 [01:50<00:09, 19.33it/s]
- more-to-come:
- class:
stderr
- 87%|████████▋ | 1223/1400 [01:50<00:09, 19.40it/s]
</pre>
- 87%|████████▋ | 1223/1400 [01:50<00:09, 19.40it/s]
end{sphinxVerbatim}
87%|████████▋ | 1223/1400 [01:50<00:09, 19.40it/s]
- more-to-come:
- class:
stderr
- 88%|████████▊ | 1226/1400 [01:50<00:09, 18.51it/s]
</pre>
- 88%|████████▊ | 1226/1400 [01:50<00:09, 18.51it/s]
end{sphinxVerbatim}
88%|████████▊ | 1226/1400 [01:50<00:09, 18.51it/s]
- more-to-come:
- class:
stderr
- 88%|████████▊ | 1230/1400 [01:51<00:07, 21.59it/s]
</pre>
- 88%|████████▊ | 1230/1400 [01:51<00:07, 21.59it/s]
end{sphinxVerbatim}
88%|████████▊ | 1230/1400 [01:51<00:07, 21.59it/s]
- more-to-come:
- class:
stderr
- 88%|████████▊ | 1234/1400 [01:51<00:06, 23.74it/s]
</pre>
- 88%|████████▊ | 1234/1400 [01:51<00:06, 23.74it/s]
end{sphinxVerbatim}
88%|████████▊ | 1234/1400 [01:51<00:06, 23.74it/s]
- more-to-come:
- class:
stderr
- 88%|████████▊ | 1237/1400 [01:51<00:08, 20.01it/s]
</pre>
- 88%|████████▊ | 1237/1400 [01:51<00:08, 20.01it/s]
end{sphinxVerbatim}
88%|████████▊ | 1237/1400 [01:51<00:08, 20.01it/s]
- more-to-come:
- class:
stderr
- 89%|████████▊ | 1240/1400 [01:51<00:08, 19.37it/s]
</pre>
- 89%|████████▊ | 1240/1400 [01:51<00:08, 19.37it/s]
end{sphinxVerbatim}
89%|████████▊ | 1240/1400 [01:51<00:08, 19.37it/s]
- more-to-come:
- class:
stderr
- 89%|████████▉ | 1243/1400 [01:51<00:08, 19.00it/s]
</pre>
- 89%|████████▉ | 1243/1400 [01:51<00:08, 19.00it/s]
end{sphinxVerbatim}
89%|████████▉ | 1243/1400 [01:51<00:08, 19.00it/s]
- more-to-come:
- class:
stderr
- 89%|████████▉ | 1245/1400 [01:51<00:08, 18.00it/s]
</pre>
- 89%|████████▉ | 1245/1400 [01:51<00:08, 18.00it/s]
end{sphinxVerbatim}
89%|████████▉ | 1245/1400 [01:51<00:08, 18.00it/s]
- more-to-come:
- class:
stderr
- 89%|████████▉ | 1247/1400 [01:51<00:08, 17.13it/s]
</pre>
- 89%|████████▉ | 1247/1400 [01:51<00:08, 17.13it/s]
end{sphinxVerbatim}
89%|████████▉ | 1247/1400 [01:51<00:08, 17.13it/s]
- more-to-come:
- class:
stderr
- 89%|████████▉ | 1250/1400 [01:52<00:08, 17.22it/s]
</pre>
- 89%|████████▉ | 1250/1400 [01:52<00:08, 17.22it/s]
end{sphinxVerbatim}
89%|████████▉ | 1250/1400 [01:52<00:08, 17.22it/s]
- more-to-come:
- class:
stderr
- 90%|████████▉ | 1253/1400 [01:52<00:07, 19.74it/s]
</pre>
- 90%|████████▉ | 1253/1400 [01:52<00:07, 19.74it/s]
end{sphinxVerbatim}
90%|████████▉ | 1253/1400 [01:52<00:07, 19.74it/s]
- more-to-come:
- class:
stderr
- 90%|████████▉ | 1256/1400 [01:52<00:06, 22.08it/s]
</pre>
- 90%|████████▉ | 1256/1400 [01:52<00:06, 22.08it/s]
end{sphinxVerbatim}
90%|████████▉ | 1256/1400 [01:52<00:06, 22.08it/s]
- more-to-come:
- class:
stderr
- 90%|████████▉ | 1259/1400 [01:52<00:06, 20.22it/s]
</pre>
- 90%|████████▉ | 1259/1400 [01:52<00:06, 20.22it/s]
end{sphinxVerbatim}
90%|████████▉ | 1259/1400 [01:52<00:06, 20.22it/s]
- more-to-come:
- class:
stderr
- 90%|█████████ | 1263/1400 [01:52<00:05, 23.22it/s]
</pre>
- 90%|█████████ | 1263/1400 [01:52<00:05, 23.22it/s]
end{sphinxVerbatim}
90%|█████████ | 1263/1400 [01:52<00:05, 23.22it/s]
- more-to-come:
- class:
stderr
- 90%|█████████ | 1266/1400 [01:52<00:06, 19.50it/s]
</pre>
- 90%|█████████ | 1266/1400 [01:52<00:06, 19.50it/s]
end{sphinxVerbatim}
90%|█████████ | 1266/1400 [01:52<00:06, 19.50it/s]
- more-to-come:
- class:
stderr
- 91%|█████████ | 1269/1400 [01:52<00:06, 21.25it/s]
</pre>
- 91%|█████████ | 1269/1400 [01:52<00:06, 21.25it/s]
end{sphinxVerbatim}
91%|█████████ | 1269/1400 [01:52<00:06, 21.25it/s]
- more-to-come:
- class:
stderr
- 91%|█████████ | 1272/1400 [01:53<00:06, 19.79it/s]
</pre>
- 91%|█████████ | 1272/1400 [01:53<00:06, 19.79it/s]
end{sphinxVerbatim}
91%|█████████ | 1272/1400 [01:53<00:06, 19.79it/s]
- more-to-come:
- class:
stderr
- 91%|█████████ | 1275/1400 [01:53<00:06, 19.14it/s]
</pre>
- 91%|█████████ | 1275/1400 [01:53<00:06, 19.14it/s]
end{sphinxVerbatim}
91%|█████████ | 1275/1400 [01:53<00:06, 19.14it/s]
- more-to-come:
- class:
stderr
- 91%|█████████▏| 1278/1400 [01:53<00:06, 18.74it/s]
</pre>
- 91%|█████████▏| 1278/1400 [01:53<00:06, 18.74it/s]
end{sphinxVerbatim}
91%|█████████▏| 1278/1400 [01:53<00:06, 18.74it/s]
- more-to-come:
- class:
stderr
- 91%|█████████▏| 1280/1400 [01:53<00:06, 17.52it/s]
</pre>
- 91%|█████████▏| 1280/1400 [01:53<00:06, 17.52it/s]
end{sphinxVerbatim}
91%|█████████▏| 1280/1400 [01:53<00:06, 17.52it/s]
- more-to-come:
- class:
stderr
- 92%|█████████▏| 1283/1400 [01:53<00:05, 20.10it/s]
</pre>
- 92%|█████████▏| 1283/1400 [01:53<00:05, 20.10it/s]
end{sphinxVerbatim}
92%|█████████▏| 1283/1400 [01:53<00:05, 20.10it/s]
- more-to-come:
- class:
stderr
- 92%|█████████▏| 1286/1400 [01:53<00:05, 19.32it/s]
</pre>
- 92%|█████████▏| 1286/1400 [01:53<00:05, 19.32it/s]
end{sphinxVerbatim}
92%|█████████▏| 1286/1400 [01:53<00:05, 19.32it/s]
- more-to-come:
- class:
stderr
- 92%|█████████▏| 1289/1400 [01:54<00:06, 16.29it/s]
</pre>
- 92%|█████████▏| 1289/1400 [01:54<00:06, 16.29it/s]
end{sphinxVerbatim}
92%|█████████▏| 1289/1400 [01:54<00:06, 16.29it/s]
- more-to-come:
- class:
stderr
- 92%|█████████▏| 1292/1400 [01:54<00:05, 18.71it/s]
</pre>
- 92%|█████████▏| 1292/1400 [01:54<00:05, 18.71it/s]
end{sphinxVerbatim}
92%|█████████▏| 1292/1400 [01:54<00:05, 18.71it/s]
- more-to-come:
- class:
stderr
- 92%|█████████▎| 1295/1400 [01:54<00:05, 20.88it/s]
</pre>
- 92%|█████████▎| 1295/1400 [01:54<00:05, 20.88it/s]
end{sphinxVerbatim}
92%|█████████▎| 1295/1400 [01:54<00:05, 20.88it/s]
- more-to-come:
- class:
stderr
- 93%|█████████▎| 1298/1400 [01:54<00:05, 20.21it/s]
</pre>
- 93%|█████████▎| 1298/1400 [01:54<00:05, 20.21it/s]
end{sphinxVerbatim}
93%|█████████▎| 1298/1400 [01:54<00:05, 20.21it/s]
- more-to-come:
- class:
stderr
- 93%|█████████▎| 1302/1400 [01:54<00:04, 23.01it/s]
</pre>
- 93%|█████████▎| 1302/1400 [01:54<00:04, 23.01it/s]
end{sphinxVerbatim}
93%|█████████▎| 1302/1400 [01:54<00:04, 23.01it/s]
- more-to-come:
- class:
stderr
- 93%|█████████▎| 1305/1400 [01:54<00:04, 20.48it/s]
</pre>
- 93%|█████████▎| 1305/1400 [01:54<00:04, 20.48it/s]
end{sphinxVerbatim}
93%|█████████▎| 1305/1400 [01:54<00:04, 20.48it/s]
- more-to-come:
- class:
stderr
- 93%|█████████▎| 1308/1400 [01:55<00:04, 19.46it/s]
</pre>
- 93%|█████████▎| 1308/1400 [01:55<00:04, 19.46it/s]
end{sphinxVerbatim}
93%|█████████▎| 1308/1400 [01:55<00:04, 19.46it/s]
- more-to-come:
- class:
stderr
- 94%|█████████▎| 1312/1400 [01:55<00:03, 22.44it/s]
</pre>
- 94%|█████████▎| 1312/1400 [01:55<00:03, 22.44it/s]
end{sphinxVerbatim}
94%|█████████▎| 1312/1400 [01:55<00:03, 22.44it/s]
- more-to-come:
- class:
stderr
- 94%|█████████▍| 1315/1400 [01:55<00:03, 23.98it/s]
</pre>
- 94%|█████████▍| 1315/1400 [01:55<00:03, 23.98it/s]
end{sphinxVerbatim}
94%|█████████▍| 1315/1400 [01:55<00:03, 23.98it/s]
- more-to-come:
- class:
stderr
- 94%|█████████▍| 1319/1400 [01:55<00:03, 25.87it/s]
</pre>
- 94%|█████████▍| 1319/1400 [01:55<00:03, 25.87it/s]
end{sphinxVerbatim}
94%|█████████▍| 1319/1400 [01:55<00:03, 25.87it/s]
- more-to-come:
- class:
stderr
- 94%|█████████▍| 1322/1400 [01:55<00:02, 26.24it/s]
</pre>
- 94%|█████████▍| 1322/1400 [01:55<00:02, 26.24it/s]
end{sphinxVerbatim}
94%|█████████▍| 1322/1400 [01:55<00:02, 26.24it/s]
- more-to-come:
- class:
stderr
- 95%|█████████▍| 1325/1400 [01:55<00:02, 26.72it/s]
</pre>
- 95%|█████████▍| 1325/1400 [01:55<00:02, 26.72it/s]
end{sphinxVerbatim}
95%|█████████▍| 1325/1400 [01:55<00:02, 26.72it/s]
- more-to-come:
- class:
stderr
- 95%|█████████▍| 1329/1400 [01:55<00:02, 27.96it/s]
</pre>
- 95%|█████████▍| 1329/1400 [01:55<00:02, 27.96it/s]
end{sphinxVerbatim}
95%|█████████▍| 1329/1400 [01:55<00:02, 27.96it/s]
- more-to-come:
- class:
stderr
- 95%|█████████▌| 1333/1400 [01:55<00:02, 28.82it/s]
</pre>
- 95%|█████████▌| 1333/1400 [01:55<00:02, 28.82it/s]
end{sphinxVerbatim}
95%|█████████▌| 1333/1400 [01:55<00:02, 28.82it/s]
- more-to-come:
- class:
stderr
- 95%|█████████▌| 1336/1400 [01:55<00:02, 29.07it/s]
</pre>
- 95%|█████████▌| 1336/1400 [01:55<00:02, 29.07it/s]
end{sphinxVerbatim}
95%|█████████▌| 1336/1400 [01:55<00:02, 29.07it/s]
- more-to-come:
- class:
stderr
- 96%|█████████▌| 1339/1400 [01:56<00:02, 25.47it/s]
</pre>
- 96%|█████████▌| 1339/1400 [01:56<00:02, 25.47it/s]
end{sphinxVerbatim}
96%|█████████▌| 1339/1400 [01:56<00:02, 25.47it/s]
- more-to-come:
- class:
stderr
- 96%|█████████▌| 1342/1400 [01:56<00:02, 22.73it/s]
</pre>
- 96%|█████████▌| 1342/1400 [01:56<00:02, 22.73it/s]
end{sphinxVerbatim}
96%|█████████▌| 1342/1400 [01:56<00:02, 22.73it/s]
- more-to-come:
- class:
stderr
- 96%|█████████▌| 1345/1400 [01:56<00:02, 24.10it/s]
</pre>
- 96%|█████████▌| 1345/1400 [01:56<00:02, 24.10it/s]
end{sphinxVerbatim}
96%|█████████▌| 1345/1400 [01:56<00:02, 24.10it/s]
- more-to-come:
- class:
stderr
- 96%|█████████▋| 1348/1400 [01:56<00:02, 25.20it/s]
</pre>
- 96%|█████████▋| 1348/1400 [01:56<00:02, 25.20it/s]
end{sphinxVerbatim}
96%|█████████▋| 1348/1400 [01:56<00:02, 25.20it/s]
- more-to-come:
- class:
stderr
- 96%|█████████▋| 1351/1400 [01:56<00:02, 22.64it/s]
</pre>
- 96%|█████████▋| 1351/1400 [01:56<00:02, 22.64it/s]
end{sphinxVerbatim}
96%|█████████▋| 1351/1400 [01:56<00:02, 22.64it/s]
- more-to-come:
- class:
stderr
- 97%|█████████▋| 1355/1400 [01:56<00:01, 25.24it/s]
</pre>
- 97%|█████████▋| 1355/1400 [01:56<00:01, 25.24it/s]
end{sphinxVerbatim}
97%|█████████▋| 1355/1400 [01:56<00:01, 25.24it/s]
- more-to-come:
- class:
stderr
- 97%|█████████▋| 1358/1400 [01:56<00:01, 26.13it/s]
</pre>
- 97%|█████████▋| 1358/1400 [01:56<00:01, 26.13it/s]
end{sphinxVerbatim}
97%|█████████▋| 1358/1400 [01:56<00:01, 26.13it/s]
- more-to-come:
- class:
stderr
- 97%|█████████▋| 1361/1400 [01:56<00:01, 26.98it/s]
</pre>
- 97%|█████████▋| 1361/1400 [01:56<00:01, 26.98it/s]
end{sphinxVerbatim}
97%|█████████▋| 1361/1400 [01:56<00:01, 26.98it/s]
- more-to-come:
- class:
stderr
- 98%|█████████▊| 1365/1400 [01:57<00:01, 27.83it/s]
</pre>
- 98%|█████████▊| 1365/1400 [01:57<00:01, 27.83it/s]
end{sphinxVerbatim}
98%|█████████▊| 1365/1400 [01:57<00:01, 27.83it/s]
- more-to-come:
- class:
stderr
- 98%|█████████▊| 1368/1400 [01:57<00:01, 27.61it/s]
</pre>
- 98%|█████████▊| 1368/1400 [01:57<00:01, 27.61it/s]
end{sphinxVerbatim}
98%|█████████▊| 1368/1400 [01:57<00:01, 27.61it/s]
- more-to-come:
- class:
stderr
- 98%|█████████▊| 1371/1400 [01:57<00:01, 28.22it/s]
</pre>
- 98%|█████████▊| 1371/1400 [01:57<00:01, 28.22it/s]
end{sphinxVerbatim}
98%|█████████▊| 1371/1400 [01:57<00:01, 28.22it/s]
- more-to-come:
- class:
stderr
- 98%|█████████▊| 1374/1400 [01:57<00:01, 24.47it/s]
</pre>
- 98%|█████████▊| 1374/1400 [01:57<00:01, 24.47it/s]
end{sphinxVerbatim}
98%|█████████▊| 1374/1400 [01:57<00:01, 24.47it/s]
- more-to-come:
- class:
stderr
- 98%|█████████▊| 1377/1400 [01:57<00:01, 22.95it/s]
</pre>
- 98%|█████████▊| 1377/1400 [01:57<00:01, 22.95it/s]
end{sphinxVerbatim}
98%|█████████▊| 1377/1400 [01:57<00:01, 22.95it/s]
- more-to-come:
- class:
stderr
- 99%|█████████▊| 1380/1400 [01:57<00:00, 24.56it/s]
</pre>
- 99%|█████████▊| 1380/1400 [01:57<00:00, 24.56it/s]
end{sphinxVerbatim}
99%|█████████▊| 1380/1400 [01:57<00:00, 24.56it/s]
- more-to-come:
- class:
stderr
- 99%|█████████▉| 1383/1400 [01:57<00:00, 21.83it/s]
</pre>
- 99%|█████████▉| 1383/1400 [01:57<00:00, 21.83it/s]
end{sphinxVerbatim}
99%|█████████▉| 1383/1400 [01:57<00:00, 21.83it/s]
- more-to-come:
- class:
stderr
- 99%|█████████▉| 1386/1400 [01:58<00:00, 23.72it/s]
</pre>
- 99%|█████████▉| 1386/1400 [01:58<00:00, 23.72it/s]
end{sphinxVerbatim}
99%|█████████▉| 1386/1400 [01:58<00:00, 23.72it/s]
- more-to-come:
- class:
stderr
- 99%|█████████▉| 1389/1400 [01:58<00:00, 25.24it/s]
</pre>
- 99%|█████████▉| 1389/1400 [01:58<00:00, 25.24it/s]
end{sphinxVerbatim}
99%|█████████▉| 1389/1400 [01:58<00:00, 25.24it/s]
- more-to-come:
- class:
stderr
- 99%|█████████▉| 1392/1400 [01:58<00:00, 21.86it/s]
</pre>
- 99%|█████████▉| 1392/1400 [01:58<00:00, 21.86it/s]
end{sphinxVerbatim}
99%|█████████▉| 1392/1400 [01:58<00:00, 21.86it/s]
- 100%|█████████▉| 1395/1400 [01:58<00:00, 19.93it/s]
</pre>
- 100%|█████████▉| 1395/1400 [01:58<00:00, 19.93it/s]
end{sphinxVerbatim}
100%|█████████▉| 1395/1400 [01:58<00:00, 19.93it/s]
- 100%|█████████▉| 1398/1400 [01:58<00:00, 21.44it/s]
</pre>
- 100%|█████████▉| 1398/1400 [01:58<00:00, 21.44it/s]
end{sphinxVerbatim}
100%|█████████▉| 1398/1400 [01:58<00:00, 21.44it/s]
- 100%|██████████| 1400/1400 [01:58<00:00, 11.79it/s]
</pre>
- 100%|██████████| 1400/1400 [01:58<00:00, 11.79it/s]
end{sphinxVerbatim}
100%|██████████| 1400/1400 [01:58<00:00, 11.79it/s]
- more-to-come:
- class:
stderr
- 0%| | 0/280 [00:00<?, ?it/s]
</pre>
- 0%| | 0/280 [00:00<?, ?it/s]
end{sphinxVerbatim}
0%| | 0/280 [00:00<?, ?it/s]
- more-to-come:
- class:
stderr
- 0%| | 1/280 [00:00<03:36, 1.29it/s]
</pre>
- 0%| | 1/280 [00:00<03:36, 1.29it/s]
end{sphinxVerbatim}
0%| | 1/280 [00:00<03:36, 1.29it/s]
- more-to-come:
- class:
stderr
- 1%| | 2/280 [00:01<02:08, 2.16it/s]
</pre>
- 1%| | 2/280 [00:01<02:08, 2.16it/s]
end{sphinxVerbatim}
1%| | 2/280 [00:01<02:08, 2.16it/s]
- more-to-come:
- class:
stderr
- 1%| | 3/280 [00:01<01:38, 2.82it/s]
</pre>
- 1%| | 3/280 [00:01<01:38, 2.82it/s]
end{sphinxVerbatim}
1%| | 3/280 [00:01<01:38, 2.82it/s]
- more-to-come:
- class:
stderr
- 1%|▏ | 4/280 [00:01<01:55, 2.39it/s]
</pre>
- 1%|▏ | 4/280 [00:01<01:55, 2.39it/s]
end{sphinxVerbatim}
1%|▏ | 4/280 [00:01<01:55, 2.39it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 5/280 [00:01<01:32, 2.98it/s]
</pre>
- 2%|▏ | 5/280 [00:01<01:32, 2.98it/s]
end{sphinxVerbatim}
2%|▏ | 5/280 [00:01<01:32, 2.98it/s]
- more-to-come:
- class:
stderr
- 2%|▏ | 6/280 [00:02<01:36, 2.85it/s]
</pre>
- 2%|▏ | 6/280 [00:02<01:36, 2.85it/s]
end{sphinxVerbatim}
2%|▏ | 6/280 [00:02<01:36, 2.85it/s]
- more-to-come:
- class:
stderr
- 2%|▎ | 7/280 [00:02<01:34, 2.88it/s]
</pre>
- 2%|▎ | 7/280 [00:02<01:34, 2.88it/s]
end{sphinxVerbatim}
2%|▎ | 7/280 [00:02<01:34, 2.88it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 8/280 [00:02<01:23, 3.25it/s]
</pre>
- 3%|▎ | 8/280 [00:02<01:23, 3.25it/s]
end{sphinxVerbatim}
3%|▎ | 8/280 [00:02<01:23, 3.25it/s]
- more-to-come:
- class:
stderr
- 3%|▎ | 9/280 [00:03<01:15, 3.58it/s]
</pre>
- 3%|▎ | 9/280 [00:03<01:15, 3.58it/s]
end{sphinxVerbatim}
3%|▎ | 9/280 [00:03<01:15, 3.58it/s]
- more-to-come:
- class:
stderr
- 4%|▎ | 10/280 [00:03<01:46, 2.53it/s]
</pre>
- 4%|▎ | 10/280 [00:03<01:46, 2.53it/s]
end{sphinxVerbatim}
4%|▎ | 10/280 [00:03<01:46, 2.53it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 11/280 [00:04<01:44, 2.57it/s]
</pre>
- 4%|▍ | 11/280 [00:04<01:44, 2.57it/s]
end{sphinxVerbatim}
4%|▍ | 11/280 [00:04<01:44, 2.57it/s]
- more-to-come:
- class:
stderr
- 4%|▍ | 12/280 [00:04<01:30, 2.95it/s]
</pre>
- 4%|▍ | 12/280 [00:04<01:30, 2.95it/s]
end{sphinxVerbatim}
4%|▍ | 12/280 [00:04<01:30, 2.95it/s]
- more-to-come:
- class:
stderr
- 5%|▍ | 13/280 [00:04<01:17, 3.43it/s]
</pre>
- 5%|▍ | 13/280 [00:04<01:17, 3.43it/s]
end{sphinxVerbatim}
5%|▍ | 13/280 [00:04<01:17, 3.43it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 14/280 [00:04<01:17, 3.44it/s]
</pre>
- 5%|▌ | 14/280 [00:04<01:17, 3.44it/s]
end{sphinxVerbatim}
5%|▌ | 14/280 [00:04<01:17, 3.44it/s]
- more-to-come:
- class:
stderr
- 5%|▌ | 15/280 [00:05<01:14, 3.55it/s]
</pre>
- 5%|▌ | 15/280 [00:05<01:14, 3.55it/s]
end{sphinxVerbatim}
5%|▌ | 15/280 [00:05<01:14, 3.55it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 16/280 [00:05<01:08, 3.86it/s]
</pre>
- 6%|▌ | 16/280 [00:05<01:08, 3.86it/s]
end{sphinxVerbatim}
6%|▌ | 16/280 [00:05<01:08, 3.86it/s]
- more-to-come:
- class:
stderr
- 6%|▌ | 17/280 [00:05<01:08, 3.83it/s]
</pre>
- 6%|▌ | 17/280 [00:05<01:08, 3.83it/s]
end{sphinxVerbatim}
6%|▌ | 17/280 [00:05<01:08, 3.83it/s]
- more-to-come:
- class:
stderr
- 6%|▋ | 18/280 [00:06<02:11, 2.00it/s]
</pre>
- 6%|▋ | 18/280 [00:06<02:11, 2.00it/s]
end{sphinxVerbatim}
6%|▋ | 18/280 [00:06<02:11, 2.00it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 19/280 [00:06<01:58, 2.21it/s]
</pre>
- 7%|▋ | 19/280 [00:06<01:58, 2.21it/s]
end{sphinxVerbatim}
7%|▋ | 19/280 [00:06<01:58, 2.21it/s]
- more-to-come:
- class:
stderr
- 7%|▋ | 20/280 [00:07<02:29, 1.74it/s]
</pre>
- 7%|▋ | 20/280 [00:07<02:29, 1.74it/s]
end{sphinxVerbatim}
7%|▋ | 20/280 [00:07<02:29, 1.74it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 21/280 [00:08<02:16, 1.90it/s]
</pre>
- 8%|▊ | 21/280 [00:08<02:16, 1.90it/s]
end{sphinxVerbatim}
8%|▊ | 21/280 [00:08<02:16, 1.90it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 22/280 [00:08<01:50, 2.34it/s]
</pre>
- 8%|▊ | 22/280 [00:08<01:50, 2.34it/s]
end{sphinxVerbatim}
8%|▊ | 22/280 [00:08<01:50, 2.34it/s]
- more-to-come:
- class:
stderr
- 8%|▊ | 23/280 [00:08<01:59, 2.16it/s]
</pre>
- 8%|▊ | 23/280 [00:08<01:59, 2.16it/s]
end{sphinxVerbatim}
8%|▊ | 23/280 [00:08<01:59, 2.16it/s]
- more-to-come:
- class:
stderr
- 9%|▊ | 24/280 [00:09<01:39, 2.58it/s]
</pre>
- 9%|▊ | 24/280 [00:09<01:39, 2.58it/s]
end{sphinxVerbatim}
9%|▊ | 24/280 [00:09<01:39, 2.58it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 25/280 [00:09<01:33, 2.72it/s]
</pre>
- 9%|▉ | 25/280 [00:09<01:33, 2.72it/s]
end{sphinxVerbatim}
9%|▉ | 25/280 [00:09<01:33, 2.72it/s]
- more-to-come:
- class:
stderr
- 9%|▉ | 26/280 [00:10<02:03, 2.06it/s]
</pre>
- 9%|▉ | 26/280 [00:10<02:03, 2.06it/s]
end{sphinxVerbatim}
9%|▉ | 26/280 [00:10<02:03, 2.06it/s]
- more-to-come:
- class:
stderr
- 10%|▉ | 27/280 [00:10<01:44, 2.42it/s]
</pre>
- 10%|▉ | 27/280 [00:10<01:44, 2.42it/s]
end{sphinxVerbatim}
10%|▉ | 27/280 [00:10<01:44, 2.42it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 28/280 [00:10<01:33, 2.70it/s]
</pre>
- 10%|█ | 28/280 [00:10<01:33, 2.70it/s]
end{sphinxVerbatim}
10%|█ | 28/280 [00:10<01:33, 2.70it/s]
- more-to-come:
- class:
stderr
- 10%|█ | 29/280 [00:11<02:06, 1.98it/s]
</pre>
- 10%|█ | 29/280 [00:11<02:06, 1.98it/s]
end{sphinxVerbatim}
10%|█ | 29/280 [00:11<02:06, 1.98it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 30/280 [00:11<01:43, 2.41it/s]
</pre>
- 11%|█ | 30/280 [00:11<01:43, 2.41it/s]
end{sphinxVerbatim}
11%|█ | 30/280 [00:11<01:43, 2.41it/s]
- more-to-come:
- class:
stderr
- 11%|█ | 31/280 [00:12<01:28, 2.83it/s]
</pre>
- 11%|█ | 31/280 [00:12<01:28, 2.83it/s]
end{sphinxVerbatim}
11%|█ | 31/280 [00:12<01:28, 2.83it/s]
- more-to-come:
- class:
stderr
- 11%|█▏ | 32/280 [00:12<01:21, 3.06it/s]
</pre>
- 11%|█▏ | 32/280 [00:12<01:21, 3.06it/s]
end{sphinxVerbatim}
11%|█▏ | 32/280 [00:12<01:21, 3.06it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 33/280 [00:12<01:15, 3.27it/s]
</pre>
- 12%|█▏ | 33/280 [00:12<01:15, 3.27it/s]
end{sphinxVerbatim}
12%|█▏ | 33/280 [00:12<01:15, 3.27it/s]
- more-to-come:
- class:
stderr
- 12%|█▏ | 34/280 [00:12<01:07, 3.64it/s]
</pre>
- 12%|█▏ | 34/280 [00:12<01:07, 3.64it/s]
end{sphinxVerbatim}
12%|█▏ | 34/280 [00:12<01:07, 3.64it/s]
- more-to-come:
- class:
stderr
- 12%|█▎ | 35/280 [00:13<01:11, 3.44it/s]
</pre>
- 12%|█▎ | 35/280 [00:13<01:11, 3.44it/s]
end{sphinxVerbatim}
12%|█▎ | 35/280 [00:13<01:11, 3.44it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 36/280 [00:13<01:07, 3.62it/s]
</pre>
- 13%|█▎ | 36/280 [00:13<01:07, 3.62it/s]
end{sphinxVerbatim}
13%|█▎ | 36/280 [00:13<01:07, 3.62it/s]
- more-to-come:
- class:
stderr
- 13%|█▎ | 37/280 [00:13<01:10, 3.47it/s]
</pre>
- 13%|█▎ | 37/280 [00:13<01:10, 3.47it/s]
end{sphinxVerbatim}
13%|█▎ | 37/280 [00:13<01:10, 3.47it/s]
- more-to-come:
- class:
stderr
- 14%|█▎ | 38/280 [00:13<01:03, 3.79it/s]
</pre>
- 14%|█▎ | 38/280 [00:13<01:03, 3.79it/s]
end{sphinxVerbatim}
14%|█▎ | 38/280 [00:13<01:03, 3.79it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 39/280 [00:14<00:58, 4.11it/s]
</pre>
- 14%|█▍ | 39/280 [00:14<00:58, 4.11it/s]
end{sphinxVerbatim}
14%|█▍ | 39/280 [00:14<00:58, 4.11it/s]
- more-to-come:
- class:
stderr
- 14%|█▍ | 40/280 [00:14<00:53, 4.45it/s]
</pre>
- 14%|█▍ | 40/280 [00:14<00:53, 4.45it/s]
end{sphinxVerbatim}
14%|█▍ | 40/280 [00:14<00:53, 4.45it/s]
- more-to-come:
- class:
stderr
- 15%|█▍ | 41/280 [00:14<00:53, 4.46it/s]
</pre>
- 15%|█▍ | 41/280 [00:14<00:53, 4.46it/s]
end{sphinxVerbatim}
15%|█▍ | 41/280 [00:14<00:53, 4.46it/s]
- more-to-come:
- class:
stderr
- 15%|█▌ | 42/280 [00:14<00:50, 4.73it/s]
</pre>
- 15%|█▌ | 42/280 [00:14<00:50, 4.73it/s]
end{sphinxVerbatim}
15%|█▌ | 42/280 [00:14<00:50, 4.73it/s]
- more-to-come:
- class:
stderr
- 15%|█▌ | 43/280 [00:14<00:51, 4.61it/s]
</pre>
- 15%|█▌ | 43/280 [00:14<00:51, 4.61it/s]
end{sphinxVerbatim}
15%|█▌ | 43/280 [00:14<00:51, 4.61it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 44/280 [00:15<00:53, 4.38it/s]
</pre>
- 16%|█▌ | 44/280 [00:15<00:53, 4.38it/s]
end{sphinxVerbatim}
16%|█▌ | 44/280 [00:15<00:53, 4.38it/s]
- more-to-come:
- class:
stderr
- 16%|█▌ | 45/280 [00:15<00:55, 4.24it/s]
</pre>
- 16%|█▌ | 45/280 [00:15<00:55, 4.24it/s]
end{sphinxVerbatim}
16%|█▌ | 45/280 [00:15<00:55, 4.24it/s]
- more-to-come:
- class:
stderr
- 16%|█▋ | 46/280 [00:15<00:50, 4.67it/s]
</pre>
- 16%|█▋ | 46/280 [00:15<00:50, 4.67it/s]
end{sphinxVerbatim}
16%|█▋ | 46/280 [00:15<00:50, 4.67it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 47/280 [00:15<01:05, 3.55it/s]
</pre>
- 17%|█▋ | 47/280 [00:15<01:05, 3.55it/s]
end{sphinxVerbatim}
17%|█▋ | 47/280 [00:15<01:05, 3.55it/s]
- more-to-come:
- class:
stderr
- 17%|█▋ | 48/280 [00:16<00:58, 4.00it/s]
</pre>
- 17%|█▋ | 48/280 [00:16<00:58, 4.00it/s]
end{sphinxVerbatim}
17%|█▋ | 48/280 [00:16<00:58, 4.00it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 49/280 [00:16<00:57, 4.04it/s]
</pre>
- 18%|█▊ | 49/280 [00:16<00:57, 4.04it/s]
end{sphinxVerbatim}
18%|█▊ | 49/280 [00:16<00:57, 4.04it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 50/280 [00:16<00:54, 4.22it/s]
</pre>
- 18%|█▊ | 50/280 [00:16<00:54, 4.22it/s]
end{sphinxVerbatim}
18%|█▊ | 50/280 [00:16<00:54, 4.22it/s]
- more-to-come:
- class:
stderr
- 18%|█▊ | 51/280 [00:16<00:48, 4.69it/s]
</pre>
- 18%|█▊ | 51/280 [00:16<00:48, 4.69it/s]
end{sphinxVerbatim}
18%|█▊ | 51/280 [00:16<00:48, 4.69it/s]
- more-to-come:
- class:
stderr
- 19%|█▊ | 52/280 [00:16<00:49, 4.58it/s]
</pre>
- 19%|█▊ | 52/280 [00:16<00:49, 4.58it/s]
end{sphinxVerbatim}
19%|█▊ | 52/280 [00:16<00:49, 4.58it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 53/280 [00:17<00:51, 4.45it/s]
</pre>
- 19%|█▉ | 53/280 [00:17<00:51, 4.45it/s]
end{sphinxVerbatim}
19%|█▉ | 53/280 [00:17<00:51, 4.45it/s]
- more-to-come:
- class:
stderr
- 19%|█▉ | 54/280 [00:17<01:24, 2.68it/s]
</pre>
- 19%|█▉ | 54/280 [00:17<01:24, 2.68it/s]
end{sphinxVerbatim}
19%|█▉ | 54/280 [00:17<01:24, 2.68it/s]
- more-to-come:
- class:
stderr
- 20%|█▉ | 55/280 [00:18<01:53, 1.98it/s]
</pre>
- 20%|█▉ | 55/280 [00:18<01:53, 1.98it/s]
end{sphinxVerbatim}
20%|█▉ | 55/280 [00:18<01:53, 1.98it/s]
- more-to-come:
- class:
stderr
- 20%|██ | 56/280 [00:18<01:34, 2.36it/s]
</pre>
- 20%|██ | 56/280 [00:18<01:34, 2.36it/s]
end{sphinxVerbatim}
20%|██ | 56/280 [00:18<01:34, 2.36it/s]
- more-to-come:
- class:
stderr
- 20%|██ | 57/280 [00:19<01:22, 2.71it/s]
</pre>
- 20%|██ | 57/280 [00:19<01:22, 2.71it/s]
end{sphinxVerbatim}
20%|██ | 57/280 [00:19<01:22, 2.71it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 58/280 [00:19<01:09, 3.18it/s]
</pre>
- 21%|██ | 58/280 [00:19<01:09, 3.18it/s]
end{sphinxVerbatim}
21%|██ | 58/280 [00:19<01:09, 3.18it/s]
- more-to-come:
- class:
stderr
- 21%|██ | 59/280 [00:19<01:05, 3.39it/s]
</pre>
- 21%|██ | 59/280 [00:19<01:05, 3.39it/s]
end{sphinxVerbatim}
21%|██ | 59/280 [00:19<01:05, 3.39it/s]
- more-to-come:
- class:
stderr
- 21%|██▏ | 60/280 [00:19<00:59, 3.68it/s]
</pre>
- 21%|██▏ | 60/280 [00:19<00:59, 3.68it/s]
end{sphinxVerbatim}
21%|██▏ | 60/280 [00:19<00:59, 3.68it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 61/280 [00:20<00:56, 3.87it/s]
</pre>
- 22%|██▏ | 61/280 [00:20<00:56, 3.87it/s]
end{sphinxVerbatim}
22%|██▏ | 61/280 [00:20<00:56, 3.87it/s]
- more-to-come:
- class:
stderr
- 22%|██▏ | 62/280 [00:20<01:19, 2.73it/s]
</pre>
- 22%|██▏ | 62/280 [00:20<01:19, 2.73it/s]
end{sphinxVerbatim}
22%|██▏ | 62/280 [00:20<01:19, 2.73it/s]
- more-to-come:
- class:
stderr
- 22%|██▎ | 63/280 [00:21<01:18, 2.77it/s]
</pre>
- 22%|██▎ | 63/280 [00:21<01:18, 2.77it/s]
end{sphinxVerbatim}
22%|██▎ | 63/280 [00:21<01:18, 2.77it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 64/280 [00:21<01:08, 3.13it/s]
</pre>
- 23%|██▎ | 64/280 [00:21<01:08, 3.13it/s]
end{sphinxVerbatim}
23%|██▎ | 64/280 [00:21<01:08, 3.13it/s]
- more-to-come:
- class:
stderr
- 23%|██▎ | 65/280 [00:21<01:10, 3.04it/s]
</pre>
- 23%|██▎ | 65/280 [00:21<01:10, 3.04it/s]
end{sphinxVerbatim}
23%|██▎ | 65/280 [00:21<01:10, 3.04it/s]
- more-to-come:
- class:
stderr
- 24%|██▎ | 66/280 [00:22<01:31, 2.35it/s]
</pre>
- 24%|██▎ | 66/280 [00:22<01:31, 2.35it/s]
end{sphinxVerbatim}
24%|██▎ | 66/280 [00:22<01:31, 2.35it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 67/280 [00:22<01:18, 2.71it/s]
</pre>
- 24%|██▍ | 67/280 [00:22<01:18, 2.71it/s]
end{sphinxVerbatim}
24%|██▍ | 67/280 [00:22<01:18, 2.71it/s]
- more-to-come:
- class:
stderr
- 24%|██▍ | 68/280 [00:22<01:11, 2.96it/s]
</pre>
- 24%|██▍ | 68/280 [00:22<01:11, 2.96it/s]
end{sphinxVerbatim}
24%|██▍ | 68/280 [00:22<01:11, 2.96it/s]
- more-to-come:
- class:
stderr
- 25%|██▍ | 69/280 [00:23<01:12, 2.91it/s]
</pre>
- 25%|██▍ | 69/280 [00:23<01:12, 2.91it/s]
end{sphinxVerbatim}
25%|██▍ | 69/280 [00:23<01:12, 2.91it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 70/280 [00:23<01:39, 2.11it/s]
</pre>
- 25%|██▌ | 70/280 [00:23<01:39, 2.11it/s]
end{sphinxVerbatim}
25%|██▌ | 70/280 [00:23<01:39, 2.11it/s]
- more-to-come:
- class:
stderr
- 25%|██▌ | 71/280 [00:24<01:23, 2.50it/s]
</pre>
- 25%|██▌ | 71/280 [00:24<01:23, 2.50it/s]
end{sphinxVerbatim}
25%|██▌ | 71/280 [00:24<01:23, 2.50it/s]
- more-to-come:
- class:
stderr
- 26%|██▌ | 72/280 [00:24<01:13, 2.82it/s]
</pre>
- 26%|██▌ | 72/280 [00:24<01:13, 2.82it/s]
end{sphinxVerbatim}
26%|██▌ | 72/280 [00:24<01:13, 2.82it/s]
- more-to-come:
- class:
stderr
- 26%|██▌ | 73/280 [00:24<01:04, 3.20it/s]
</pre>
- 26%|██▌ | 73/280 [00:24<01:04, 3.20it/s]
end{sphinxVerbatim}
26%|██▌ | 73/280 [00:24<01:04, 3.20it/s]
- more-to-come:
- class:
stderr
- 26%|██▋ | 74/280 [00:25<01:11, 2.87it/s]
</pre>
- 26%|██▋ | 74/280 [00:25<01:11, 2.87it/s]
end{sphinxVerbatim}
26%|██▋ | 74/280 [00:25<01:11, 2.87it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 75/280 [00:25<01:19, 2.58it/s]
</pre>
- 27%|██▋ | 75/280 [00:25<01:19, 2.58it/s]
end{sphinxVerbatim}
27%|██▋ | 75/280 [00:25<01:19, 2.58it/s]
- more-to-come:
- class:
stderr
- 27%|██▋ | 76/280 [00:25<01:05, 3.13it/s]
</pre>
- 27%|██▋ | 76/280 [00:25<01:05, 3.13it/s]
end{sphinxVerbatim}
27%|██▋ | 76/280 [00:25<01:05, 3.13it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 77/280 [00:25<00:58, 3.45it/s]
</pre>
- 28%|██▊ | 77/280 [00:25<00:58, 3.45it/s]
end{sphinxVerbatim}
28%|██▊ | 77/280 [00:25<00:58, 3.45it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 78/280 [00:26<00:55, 3.63it/s]
</pre>
- 28%|██▊ | 78/280 [00:26<00:55, 3.63it/s]
end{sphinxVerbatim}
28%|██▊ | 78/280 [00:26<00:55, 3.63it/s]
- more-to-come:
- class:
stderr
- 28%|██▊ | 79/280 [00:26<00:51, 3.89it/s]
</pre>
- 28%|██▊ | 79/280 [00:26<00:51, 3.89it/s]
end{sphinxVerbatim}
28%|██▊ | 79/280 [00:26<00:51, 3.89it/s]
- more-to-come:
- class:
stderr
- 29%|██▊ | 80/280 [00:26<00:48, 4.10it/s]
</pre>
- 29%|██▊ | 80/280 [00:26<00:48, 4.10it/s]
end{sphinxVerbatim}
29%|██▊ | 80/280 [00:26<00:48, 4.10it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 81/280 [00:26<00:47, 4.15it/s]
</pre>
- 29%|██▉ | 81/280 [00:26<00:47, 4.15it/s]
end{sphinxVerbatim}
29%|██▉ | 81/280 [00:26<00:47, 4.15it/s]
- more-to-come:
- class:
stderr
- 29%|██▉ | 82/280 [00:27<00:46, 4.27it/s]
</pre>
- 29%|██▉ | 82/280 [00:27<00:46, 4.27it/s]
end{sphinxVerbatim}
29%|██▉ | 82/280 [00:27<00:46, 4.27it/s]
- more-to-come:
- class:
stderr
- 30%|██▉ | 83/280 [00:27<00:43, 4.49it/s]
</pre>
- 30%|██▉ | 83/280 [00:27<00:43, 4.49it/s]
end{sphinxVerbatim}
30%|██▉ | 83/280 [00:27<00:43, 4.49it/s]
- more-to-come:
- class:
stderr
- 30%|███ | 84/280 [00:27<00:44, 4.40it/s]
</pre>
- 30%|███ | 84/280 [00:27<00:44, 4.40it/s]
end{sphinxVerbatim}
30%|███ | 84/280 [00:27<00:44, 4.40it/s]
- more-to-come:
- class:
stderr
- 30%|███ | 85/280 [00:28<01:06, 2.95it/s]
</pre>
- 30%|███ | 85/280 [00:28<01:06, 2.95it/s]
end{sphinxVerbatim}
30%|███ | 85/280 [00:28<01:06, 2.95it/s]
- more-to-come:
- class:
stderr
- 31%|███ | 86/280 [00:28<00:58, 3.33it/s]
</pre>
- 31%|███ | 86/280 [00:28<00:58, 3.33it/s]
end{sphinxVerbatim}
31%|███ | 86/280 [00:28<00:58, 3.33it/s]
- more-to-come:
- class:
stderr
- 31%|███ | 87/280 [00:28<00:51, 3.74it/s]
</pre>
- 31%|███ | 87/280 [00:28<00:51, 3.74it/s]
end{sphinxVerbatim}
31%|███ | 87/280 [00:28<00:51, 3.74it/s]
- more-to-come:
- class:
stderr
- 31%|███▏ | 88/280 [00:28<00:49, 3.87it/s]
</pre>
- 31%|███▏ | 88/280 [00:28<00:49, 3.87it/s]
end{sphinxVerbatim}
31%|███▏ | 88/280 [00:28<00:49, 3.87it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 89/280 [00:28<00:47, 4.02it/s]
</pre>
- 32%|███▏ | 89/280 [00:28<00:47, 4.02it/s]
end{sphinxVerbatim}
32%|███▏ | 89/280 [00:28<00:47, 4.02it/s]
- more-to-come:
- class:
stderr
- 32%|███▏ | 90/280 [00:29<00:48, 3.96it/s]
</pre>
- 32%|███▏ | 90/280 [00:29<00:48, 3.96it/s]
end{sphinxVerbatim}
32%|███▏ | 90/280 [00:29<00:48, 3.96it/s]
- more-to-come:
- class:
stderr
- 32%|███▎ | 91/280 [00:29<00:46, 4.10it/s]
</pre>
- 32%|███▎ | 91/280 [00:29<00:46, 4.10it/s]
end{sphinxVerbatim}
32%|███▎ | 91/280 [00:29<00:46, 4.10it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 92/280 [00:30<01:22, 2.27it/s]
</pre>
- 33%|███▎ | 92/280 [00:30<01:22, 2.27it/s]
end{sphinxVerbatim}
33%|███▎ | 92/280 [00:30<01:22, 2.27it/s]
- more-to-come:
- class:
stderr
- 33%|███▎ | 93/280 [00:30<01:09, 2.68it/s]
</pre>
- 33%|███▎ | 93/280 [00:30<01:09, 2.68it/s]
end{sphinxVerbatim}
33%|███▎ | 93/280 [00:30<01:09, 2.68it/s]
- more-to-come:
- class:
stderr
- 34%|███▎ | 94/280 [00:30<01:02, 2.98it/s]
</pre>
- 34%|███▎ | 94/280 [00:30<01:02, 2.98it/s]
end{sphinxVerbatim}
34%|███▎ | 94/280 [00:30<01:02, 2.98it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 95/280 [00:31<01:00, 3.08it/s]
</pre>
- 34%|███▍ | 95/280 [00:31<01:00, 3.08it/s]
end{sphinxVerbatim}
34%|███▍ | 95/280 [00:31<01:00, 3.08it/s]
- more-to-come:
- class:
stderr
- 34%|███▍ | 96/280 [00:31<01:03, 2.91it/s]
</pre>
- 34%|███▍ | 96/280 [00:31<01:03, 2.91it/s]
end{sphinxVerbatim}
34%|███▍ | 96/280 [00:31<01:03, 2.91it/s]
- more-to-come:
- class:
stderr
- 35%|███▍ | 97/280 [00:31<00:56, 3.23it/s]
</pre>
- 35%|███▍ | 97/280 [00:31<00:56, 3.23it/s]
end{sphinxVerbatim}
35%|███▍ | 97/280 [00:31<00:56, 3.23it/s]
- more-to-come:
- class:
stderr
- 35%|███▌ | 98/280 [00:32<00:57, 3.18it/s]
</pre>
- 35%|███▌ | 98/280 [00:32<00:57, 3.18it/s]
end{sphinxVerbatim}
35%|███▌ | 98/280 [00:32<00:57, 3.18it/s]
- more-to-come:
- class:
stderr
- 35%|███▌ | 99/280 [00:32<01:00, 2.99it/s]
</pre>
- 35%|███▌ | 99/280 [00:32<01:00, 2.99it/s]
end{sphinxVerbatim}
35%|███▌ | 99/280 [00:32<01:00, 2.99it/s]
- more-to-come:
- class:
stderr
- 36%|███▌ | 100/280 [00:32<00:59, 3.05it/s]
</pre>
- 36%|███▌ | 100/280 [00:32<00:59, 3.05it/s]
end{sphinxVerbatim}
36%|███▌ | 100/280 [00:32<00:59, 3.05it/s]
- more-to-come:
- class:
stderr
- 36%|███▌ | 101/280 [00:32<00:49, 3.61it/s]
</pre>
- 36%|███▌ | 101/280 [00:32<00:49, 3.61it/s]
end{sphinxVerbatim}
36%|███▌ | 101/280 [00:32<00:49, 3.61it/s]
- more-to-come:
- class:
stderr
- 36%|███▋ | 102/280 [00:33<00:44, 4.04it/s]
</pre>
- 36%|███▋ | 102/280 [00:33<00:44, 4.04it/s]
end{sphinxVerbatim}
36%|███▋ | 102/280 [00:33<00:44, 4.04it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 103/280 [00:33<00:39, 4.53it/s]
</pre>
- 37%|███▋ | 103/280 [00:33<00:39, 4.53it/s]
end{sphinxVerbatim}
37%|███▋ | 103/280 [00:33<00:39, 4.53it/s]
- more-to-come:
- class:
stderr
- 37%|███▋ | 104/280 [00:33<00:39, 4.45it/s]
</pre>
- 37%|███▋ | 104/280 [00:33<00:39, 4.45it/s]
end{sphinxVerbatim}
37%|███▋ | 104/280 [00:33<00:39, 4.45it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 105/280 [00:33<00:38, 4.57it/s]
</pre>
- 38%|███▊ | 105/280 [00:33<00:38, 4.57it/s]
end{sphinxVerbatim}
38%|███▊ | 105/280 [00:33<00:38, 4.57it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 106/280 [00:33<00:42, 4.07it/s]
</pre>
- 38%|███▊ | 106/280 [00:33<00:42, 4.07it/s]
end{sphinxVerbatim}
38%|███▊ | 106/280 [00:33<00:42, 4.07it/s]
- more-to-come:
- class:
stderr
- 38%|███▊ | 107/280 [00:34<00:41, 4.21it/s]
</pre>
- 38%|███▊ | 107/280 [00:34<00:41, 4.21it/s]
end{sphinxVerbatim}
38%|███▊ | 107/280 [00:34<00:41, 4.21it/s]
- more-to-come:
- class:
stderr
- 39%|███▊ | 108/280 [00:34<00:39, 4.36it/s]
</pre>
- 39%|███▊ | 108/280 [00:34<00:39, 4.36it/s]
end{sphinxVerbatim}
39%|███▊ | 108/280 [00:34<00:39, 4.36it/s]
- more-to-come:
- class:
stderr
- 39%|███▉ | 109/280 [00:34<00:39, 4.38it/s]
</pre>
- 39%|███▉ | 109/280 [00:34<00:39, 4.38it/s]
end{sphinxVerbatim}
39%|███▉ | 109/280 [00:34<00:39, 4.38it/s]
- more-to-come:
- class:
stderr
- 39%|███▉ | 110/280 [00:35<00:57, 2.95it/s]
</pre>
- 39%|███▉ | 110/280 [00:35<00:57, 2.95it/s]
end{sphinxVerbatim}
39%|███▉ | 110/280 [00:35<00:57, 2.95it/s]
- more-to-come:
- class:
stderr
- 40%|███▉ | 111/280 [00:35<00:52, 3.21it/s]
</pre>
- 40%|███▉ | 111/280 [00:35<00:52, 3.21it/s]
end{sphinxVerbatim}
40%|███▉ | 111/280 [00:35<00:52, 3.21it/s]
- more-to-come:
- class:
stderr
- 40%|████ | 112/280 [00:36<01:10, 2.38it/s]
</pre>
- 40%|████ | 112/280 [00:36<01:10, 2.38it/s]
end{sphinxVerbatim}
40%|████ | 112/280 [00:36<01:10, 2.38it/s]
- more-to-come:
- class:
stderr
- 40%|████ | 113/280 [00:36<01:03, 2.64it/s]
</pre>
- 40%|████ | 113/280 [00:36<01:03, 2.64it/s]
end{sphinxVerbatim}
40%|████ | 113/280 [00:36<01:03, 2.64it/s]
- more-to-come:
- class:
stderr
- 41%|████ | 114/280 [00:37<01:21, 2.03it/s]
</pre>
- 41%|████ | 114/280 [00:37<01:21, 2.03it/s]
end{sphinxVerbatim}
41%|████ | 114/280 [00:37<01:21, 2.03it/s]
- more-to-come:
- class:
stderr
- 41%|████ | 115/280 [00:37<01:10, 2.34it/s]
</pre>
- 41%|████ | 115/280 [00:37<01:10, 2.34it/s]
end{sphinxVerbatim}
41%|████ | 115/280 [00:37<01:10, 2.34it/s]
- more-to-come:
- class:
stderr
- 41%|████▏ | 116/280 [00:37<01:01, 2.69it/s]
</pre>
- 41%|████▏ | 116/280 [00:37<01:01, 2.69it/s]
end{sphinxVerbatim}
41%|████▏ | 116/280 [00:37<01:01, 2.69it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 117/280 [00:37<00:51, 3.19it/s]
</pre>
- 42%|████▏ | 117/280 [00:37<00:51, 3.19it/s]
end{sphinxVerbatim}
42%|████▏ | 117/280 [00:37<00:51, 3.19it/s]
- more-to-come:
- class:
stderr
- 42%|████▏ | 118/280 [00:38<00:52, 3.08it/s]
</pre>
- 42%|████▏ | 118/280 [00:38<00:52, 3.08it/s]
end{sphinxVerbatim}
42%|████▏ | 118/280 [00:38<00:52, 3.08it/s]
- more-to-come:
- class:
stderr
- 42%|████▎ | 119/280 [00:38<00:49, 3.22it/s]
</pre>
- 42%|████▎ | 119/280 [00:38<00:49, 3.22it/s]
end{sphinxVerbatim}
42%|████▎ | 119/280 [00:38<00:49, 3.22it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 120/280 [00:38<00:48, 3.31it/s]
</pre>
- 43%|████▎ | 120/280 [00:38<00:48, 3.31it/s]
end{sphinxVerbatim}
43%|████▎ | 120/280 [00:38<00:48, 3.31it/s]
- more-to-come:
- class:
stderr
- 43%|████▎ | 121/280 [00:39<00:47, 3.34it/s]
</pre>
- 43%|████▎ | 121/280 [00:39<00:47, 3.34it/s]
end{sphinxVerbatim}
43%|████▎ | 121/280 [00:39<00:47, 3.34it/s]
- more-to-come:
- class:
stderr
- 44%|████▎ | 122/280 [00:39<00:41, 3.77it/s]
</pre>
- 44%|████▎ | 122/280 [00:39<00:41, 3.77it/s]
end{sphinxVerbatim}
44%|████▎ | 122/280 [00:39<00:41, 3.77it/s]
- more-to-come:
- class:
stderr
- 44%|████▍ | 123/280 [00:39<00:39, 3.97it/s]
</pre>
- 44%|████▍ | 123/280 [00:39<00:39, 3.97it/s]
end{sphinxVerbatim}
44%|████▍ | 123/280 [00:39<00:39, 3.97it/s]
- more-to-come:
- class:
stderr
- 44%|████▍ | 124/280 [00:40<00:55, 2.79it/s]
</pre>
- 44%|████▍ | 124/280 [00:40<00:55, 2.79it/s]
end{sphinxVerbatim}
44%|████▍ | 124/280 [00:40<00:55, 2.79it/s]
- more-to-come:
- class:
stderr
- 45%|████▍ | 125/280 [00:40<00:47, 3.24it/s]
</pre>
- 45%|████▍ | 125/280 [00:40<00:47, 3.24it/s]
end{sphinxVerbatim}
45%|████▍ | 125/280 [00:40<00:47, 3.24it/s]
- more-to-come:
- class:
stderr
- 45%|████▌ | 126/280 [00:40<00:45, 3.39it/s]
</pre>
- 45%|████▌ | 126/280 [00:40<00:45, 3.39it/s]
end{sphinxVerbatim}
45%|████▌ | 126/280 [00:40<00:45, 3.39it/s]
- more-to-come:
- class:
stderr
- 45%|████▌ | 127/280 [00:40<00:43, 3.50it/s]
</pre>
- 45%|████▌ | 127/280 [00:40<00:43, 3.50it/s]
end{sphinxVerbatim}
45%|████▌ | 127/280 [00:40<00:43, 3.50it/s]
- more-to-come:
- class:
stderr
- 46%|████▌ | 128/280 [00:41<00:57, 2.66it/s]
</pre>
- 46%|████▌ | 128/280 [00:41<00:57, 2.66it/s]
end{sphinxVerbatim}
46%|████▌ | 128/280 [00:41<00:57, 2.66it/s]
- more-to-come:
- class:
stderr
- 46%|████▌ | 129/280 [00:41<00:49, 3.05it/s]
</pre>
- 46%|████▌ | 129/280 [00:41<00:49, 3.05it/s]
end{sphinxVerbatim}
46%|████▌ | 129/280 [00:41<00:49, 3.05it/s]
- more-to-come:
- class:
stderr
- 46%|████▋ | 130/280 [00:41<00:45, 3.27it/s]
</pre>
- 46%|████▋ | 130/280 [00:41<00:45, 3.27it/s]
end{sphinxVerbatim}
46%|████▋ | 130/280 [00:41<00:45, 3.27it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 131/280 [00:42<00:40, 3.64it/s]
</pre>
- 47%|████▋ | 131/280 [00:42<00:40, 3.64it/s]
end{sphinxVerbatim}
47%|████▋ | 131/280 [00:42<00:40, 3.64it/s]
- more-to-come:
- class:
stderr
- 47%|████▋ | 132/280 [00:42<00:38, 3.86it/s]
</pre>
- 47%|████▋ | 132/280 [00:42<00:38, 3.86it/s]
end{sphinxVerbatim}
47%|████▋ | 132/280 [00:42<00:38, 3.86it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 133/280 [00:42<00:35, 4.15it/s]
</pre>
- 48%|████▊ | 133/280 [00:42<00:35, 4.15it/s]
end{sphinxVerbatim}
48%|████▊ | 133/280 [00:42<00:35, 4.15it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 134/280 [00:43<00:47, 3.10it/s]
</pre>
- 48%|████▊ | 134/280 [00:43<00:47, 3.10it/s]
end{sphinxVerbatim}
48%|████▊ | 134/280 [00:43<00:47, 3.10it/s]
- more-to-come:
- class:
stderr
- 48%|████▊ | 135/280 [00:43<00:42, 3.40it/s]
</pre>
- 48%|████▊ | 135/280 [00:43<00:42, 3.40it/s]
end{sphinxVerbatim}
48%|████▊ | 135/280 [00:43<00:42, 3.40it/s]
- more-to-come:
- class:
stderr
- 49%|████▊ | 136/280 [00:43<00:38, 3.73it/s]
</pre>
- 49%|████▊ | 136/280 [00:43<00:38, 3.73it/s]
end{sphinxVerbatim}
49%|████▊ | 136/280 [00:43<00:38, 3.73it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 137/280 [00:43<00:35, 4.07it/s]
</pre>
- 49%|████▉ | 137/280 [00:43<00:35, 4.07it/s]
end{sphinxVerbatim}
49%|████▉ | 137/280 [00:43<00:35, 4.07it/s]
- more-to-come:
- class:
stderr
- 49%|████▉ | 138/280 [00:43<00:32, 4.31it/s]
</pre>
- 49%|████▉ | 138/280 [00:43<00:32, 4.31it/s]
end{sphinxVerbatim}
49%|████▉ | 138/280 [00:43<00:32, 4.31it/s]
- more-to-come:
- class:
stderr
- 50%|████▉ | 139/280 [00:44<00:33, 4.20it/s]
</pre>
- 50%|████▉ | 139/280 [00:44<00:33, 4.20it/s]
end{sphinxVerbatim}
50%|████▉ | 139/280 [00:44<00:33, 4.20it/s]
- more-to-come:
- class:
stderr
- 50%|█████ | 140/280 [00:44<00:47, 2.93it/s]
</pre>
- 50%|█████ | 140/280 [00:44<00:47, 2.93it/s]
end{sphinxVerbatim}
50%|█████ | 140/280 [00:44<00:47, 2.93it/s]
- more-to-come:
- class:
stderr
- 50%|█████ | 141/280 [00:45<00:50, 2.76it/s]
</pre>
- 50%|█████ | 141/280 [00:45<00:50, 2.76it/s]
end{sphinxVerbatim}
50%|█████ | 141/280 [00:45<00:50, 2.76it/s]
- more-to-come:
- class:
stderr
- 51%|█████ | 142/280 [00:45<00:45, 3.01it/s]
</pre>
- 51%|█████ | 142/280 [00:45<00:45, 3.01it/s]
end{sphinxVerbatim}
51%|█████ | 142/280 [00:45<00:45, 3.01it/s]
- more-to-come:
- class:
stderr
- 51%|█████ | 143/280 [00:45<00:56, 2.42it/s]
</pre>
- 51%|█████ | 143/280 [00:45<00:56, 2.42it/s]
end{sphinxVerbatim}
51%|█████ | 143/280 [00:45<00:56, 2.42it/s]
- more-to-come:
- class:
stderr
- 51%|█████▏ | 144/280 [00:46<00:50, 2.71it/s]
</pre>
- 51%|█████▏ | 144/280 [00:46<00:50, 2.71it/s]
end{sphinxVerbatim}
51%|█████▏ | 144/280 [00:46<00:50, 2.71it/s]
- more-to-come:
- class:
stderr
- 52%|█████▏ | 145/280 [00:46<00:44, 3.05it/s]
</pre>
- 52%|█████▏ | 145/280 [00:46<00:44, 3.05it/s]
end{sphinxVerbatim}
52%|█████▏ | 145/280 [00:46<00:44, 3.05it/s]
- more-to-come:
- class:
stderr
- 52%|█████▏ | 146/280 [00:46<00:43, 3.12it/s]
</pre>
- 52%|█████▏ | 146/280 [00:46<00:43, 3.12it/s]
end{sphinxVerbatim}
52%|█████▏ | 146/280 [00:46<00:43, 3.12it/s]
- more-to-come:
- class:
stderr
- 52%|█████▎ | 147/280 [00:47<00:48, 2.77it/s]
</pre>
- 52%|█████▎ | 147/280 [00:47<00:48, 2.77it/s]
end{sphinxVerbatim}
52%|█████▎ | 147/280 [00:47<00:48, 2.77it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 148/280 [00:47<00:41, 3.22it/s]
</pre>
- 53%|█████▎ | 148/280 [00:47<00:41, 3.22it/s]
end{sphinxVerbatim}
53%|█████▎ | 148/280 [00:47<00:41, 3.22it/s]
- more-to-come:
- class:
stderr
- 53%|█████▎ | 149/280 [00:47<00:37, 3.51it/s]
</pre>
- 53%|█████▎ | 149/280 [00:47<00:37, 3.51it/s]
end{sphinxVerbatim}
53%|█████▎ | 149/280 [00:47<00:37, 3.51it/s]
- more-to-come:
- class:
stderr
- 54%|█████▎ | 150/280 [00:47<00:34, 3.73it/s]
</pre>
- 54%|█████▎ | 150/280 [00:47<00:34, 3.73it/s]
end{sphinxVerbatim}
54%|█████▎ | 150/280 [00:47<00:34, 3.73it/s]
- more-to-come:
- class:
stderr
- 54%|█████▍ | 151/280 [00:48<00:31, 4.04it/s]
</pre>
- 54%|█████▍ | 151/280 [00:48<00:31, 4.04it/s]
end{sphinxVerbatim}
54%|█████▍ | 151/280 [00:48<00:31, 4.04it/s]
- more-to-come:
- class:
stderr
- 54%|█████▍ | 152/280 [00:48<00:47, 2.71it/s]
</pre>
- 54%|█████▍ | 152/280 [00:48<00:47, 2.71it/s]
end{sphinxVerbatim}
54%|█████▍ | 152/280 [00:48<00:47, 2.71it/s]
- more-to-come:
- class:
stderr
- 55%|█████▍ | 153/280 [00:49<00:56, 2.24it/s]
</pre>
- 55%|█████▍ | 153/280 [00:49<00:56, 2.24it/s]
end{sphinxVerbatim}
55%|█████▍ | 153/280 [00:49<00:56, 2.24it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 154/280 [00:49<00:47, 2.63it/s]
</pre>
- 55%|█████▌ | 154/280 [00:49<00:47, 2.63it/s]
end{sphinxVerbatim}
55%|█████▌ | 154/280 [00:49<00:47, 2.63it/s]
- more-to-come:
- class:
stderr
- 55%|█████▌ | 155/280 [00:49<00:41, 3.03it/s]
</pre>
- 55%|█████▌ | 155/280 [00:49<00:41, 3.03it/s]
end{sphinxVerbatim}
55%|█████▌ | 155/280 [00:49<00:41, 3.03it/s]
- more-to-come:
- class:
stderr
- 56%|█████▌ | 156/280 [00:49<00:36, 3.44it/s]
</pre>
- 56%|█████▌ | 156/280 [00:49<00:36, 3.44it/s]
end{sphinxVerbatim}
56%|█████▌ | 156/280 [00:49<00:36, 3.44it/s]
- more-to-come:
- class:
stderr
- 56%|█████▌ | 157/280 [00:50<00:48, 2.56it/s]
</pre>
- 56%|█████▌ | 157/280 [00:50<00:48, 2.56it/s]
end{sphinxVerbatim}
56%|█████▌ | 157/280 [00:50<00:48, 2.56it/s]
- more-to-come:
- class:
stderr
- 56%|█████▋ | 158/280 [00:50<00:43, 2.79it/s]
</pre>
- 56%|█████▋ | 158/280 [00:50<00:43, 2.79it/s]
end{sphinxVerbatim}
56%|█████▋ | 158/280 [00:50<00:43, 2.79it/s]
- more-to-come:
- class:
stderr
- 57%|█████▋ | 159/280 [00:51<00:37, 3.23it/s]
</pre>
- 57%|█████▋ | 159/280 [00:51<00:37, 3.23it/s]
end{sphinxVerbatim}
57%|█████▋ | 159/280 [00:51<00:37, 3.23it/s]
- more-to-come:
- class:
stderr
- 57%|█████▋ | 160/280 [00:51<00:32, 3.68it/s]
</pre>
- 57%|█████▋ | 160/280 [00:51<00:32, 3.68it/s]
end{sphinxVerbatim}
57%|█████▋ | 160/280 [00:51<00:32, 3.68it/s]
- more-to-come:
- class:
stderr
- 57%|█████▊ | 161/280 [00:51<00:43, 2.76it/s]
</pre>
- 57%|█████▊ | 161/280 [00:51<00:43, 2.76it/s]
end{sphinxVerbatim}
57%|█████▊ | 161/280 [00:51<00:43, 2.76it/s]
- more-to-come:
- class:
stderr
- 58%|█████▊ | 162/280 [00:51<00:35, 3.34it/s]
</pre>
- 58%|█████▊ | 162/280 [00:51<00:35, 3.34it/s]
end{sphinxVerbatim}
58%|█████▊ | 162/280 [00:51<00:35, 3.34it/s]
- more-to-come:
- class:
stderr
- 58%|█████▊ | 163/280 [00:52<00:33, 3.51it/s]
</pre>
- 58%|█████▊ | 163/280 [00:52<00:33, 3.51it/s]
end{sphinxVerbatim}
58%|█████▊ | 163/280 [00:52<00:33, 3.51it/s]
- more-to-come:
- class:
stderr
- 59%|█████▊ | 164/280 [00:52<00:28, 4.05it/s]
</pre>
- 59%|█████▊ | 164/280 [00:52<00:28, 4.05it/s]
end{sphinxVerbatim}
59%|█████▊ | 164/280 [00:52<00:28, 4.05it/s]
- more-to-come:
- class:
stderr
- 59%|█████▉ | 165/280 [00:52<00:28, 4.01it/s]
</pre>
- 59%|█████▉ | 165/280 [00:52<00:28, 4.01it/s]
end{sphinxVerbatim}
59%|█████▉ | 165/280 [00:52<00:28, 4.01it/s]
- more-to-come:
- class:
stderr
- 59%|█████▉ | 166/280 [00:52<00:28, 4.03it/s]
</pre>
- 59%|█████▉ | 166/280 [00:52<00:28, 4.03it/s]
end{sphinxVerbatim}
59%|█████▉ | 166/280 [00:52<00:28, 4.03it/s]
- more-to-come:
- class:
stderr
- 60%|█████▉ | 167/280 [00:53<00:26, 4.24it/s]
</pre>
- 60%|█████▉ | 167/280 [00:53<00:26, 4.24it/s]
end{sphinxVerbatim}
60%|█████▉ | 167/280 [00:53<00:26, 4.24it/s]
- more-to-come:
- class:
stderr
- 60%|██████ | 168/280 [00:53<00:36, 3.10it/s]
</pre>
- 60%|██████ | 168/280 [00:53<00:36, 3.10it/s]
end{sphinxVerbatim}
60%|██████ | 168/280 [00:53<00:36, 3.10it/s]
- more-to-come:
- class:
stderr
- 60%|██████ | 169/280 [00:54<00:40, 2.72it/s]
</pre>
- 60%|██████ | 169/280 [00:54<00:40, 2.72it/s]
end{sphinxVerbatim}
60%|██████ | 169/280 [00:54<00:40, 2.72it/s]
- more-to-come:
- class:
stderr
- 61%|██████ | 170/280 [00:54<00:49, 2.20it/s]
</pre>
- 61%|██████ | 170/280 [00:54<00:49, 2.20it/s]
end{sphinxVerbatim}
61%|██████ | 170/280 [00:54<00:49, 2.20it/s]
- more-to-come:
- class:
stderr
- 61%|██████ | 171/280 [00:55<00:56, 1.94it/s]
</pre>
- 61%|██████ | 171/280 [00:55<00:56, 1.94it/s]
end{sphinxVerbatim}
61%|██████ | 171/280 [00:55<00:56, 1.94it/s]
- more-to-come:
- class:
stderr
- 61%|██████▏ | 172/280 [00:55<00:46, 2.33it/s]
</pre>
- 61%|██████▏ | 172/280 [00:55<00:46, 2.33it/s]
end{sphinxVerbatim}
61%|██████▏ | 172/280 [00:55<00:46, 2.33it/s]
- more-to-come:
- class:
stderr
- 62%|██████▏ | 173/280 [00:55<00:37, 2.84it/s]
</pre>
- 62%|██████▏ | 173/280 [00:55<00:37, 2.84it/s]
end{sphinxVerbatim}
62%|██████▏ | 173/280 [00:55<00:37, 2.84it/s]
- more-to-come:
- class:
stderr
- 62%|██████▏ | 174/280 [00:56<00:34, 3.11it/s]
</pre>
- 62%|██████▏ | 174/280 [00:56<00:34, 3.11it/s]
end{sphinxVerbatim}
62%|██████▏ | 174/280 [00:56<00:34, 3.11it/s]
- more-to-come:
- class:
stderr
- 62%|██████▎ | 175/280 [00:56<00:43, 2.44it/s]
</pre>
- 62%|██████▎ | 175/280 [00:56<00:43, 2.44it/s]
end{sphinxVerbatim}
62%|██████▎ | 175/280 [00:56<00:43, 2.44it/s]
- more-to-come:
- class:
stderr
- 63%|██████▎ | 176/280 [00:56<00:36, 2.84it/s]
</pre>
- 63%|██████▎ | 176/280 [00:56<00:36, 2.84it/s]
end{sphinxVerbatim}
63%|██████▎ | 176/280 [00:56<00:36, 2.84it/s]
- more-to-come:
- class:
stderr
- 63%|██████▎ | 177/280 [00:57<00:31, 3.24it/s]
</pre>
- 63%|██████▎ | 177/280 [00:57<00:31, 3.24it/s]
end{sphinxVerbatim}
63%|██████▎ | 177/280 [00:57<00:31, 3.24it/s]
- more-to-come:
- class:
stderr
- 64%|██████▎ | 178/280 [00:57<00:28, 3.56it/s]
</pre>
- 64%|██████▎ | 178/280 [00:57<00:28, 3.56it/s]
end{sphinxVerbatim}
64%|██████▎ | 178/280 [00:57<00:28, 3.56it/s]
- more-to-come:
- class:
stderr
- 64%|██████▍ | 179/280 [00:57<00:26, 3.85it/s]
</pre>
- 64%|██████▍ | 179/280 [00:57<00:26, 3.85it/s]
end{sphinxVerbatim}
64%|██████▍ | 179/280 [00:57<00:26, 3.85it/s]
- more-to-come:
- class:
stderr
- 64%|██████▍ | 180/280 [00:57<00:24, 4.04it/s]
</pre>
- 64%|██████▍ | 180/280 [00:57<00:24, 4.04it/s]
end{sphinxVerbatim}
64%|██████▍ | 180/280 [00:57<00:24, 4.04it/s]
- more-to-come:
- class:
stderr
- 65%|██████▍ | 181/280 [00:57<00:22, 4.38it/s]
</pre>
- 65%|██████▍ | 181/280 [00:57<00:22, 4.38it/s]
end{sphinxVerbatim}
65%|██████▍ | 181/280 [00:57<00:22, 4.38it/s]
- more-to-come:
- class:
stderr
- 65%|██████▌ | 182/280 [00:58<00:22, 4.41it/s]
</pre>
- 65%|██████▌ | 182/280 [00:58<00:22, 4.41it/s]
end{sphinxVerbatim}
65%|██████▌ | 182/280 [00:58<00:22, 4.41it/s]
- more-to-come:
- class:
stderr
- 65%|██████▌ | 183/280 [00:58<00:34, 2.82it/s]
</pre>
- 65%|██████▌ | 183/280 [00:58<00:34, 2.82it/s]
end{sphinxVerbatim}
65%|██████▌ | 183/280 [00:58<00:34, 2.82it/s]
- more-to-come:
- class:
stderr
- 66%|██████▌ | 184/280 [00:59<00:42, 2.27it/s]
</pre>
- 66%|██████▌ | 184/280 [00:59<00:42, 2.27it/s]
end{sphinxVerbatim}
66%|██████▌ | 184/280 [00:59<00:42, 2.27it/s]
- more-to-come:
- class:
stderr
- 66%|██████▌ | 185/280 [00:59<00:34, 2.72it/s]
</pre>
- 66%|██████▌ | 185/280 [00:59<00:34, 2.72it/s]
end{sphinxVerbatim}
66%|██████▌ | 185/280 [00:59<00:34, 2.72it/s]
- more-to-come:
- class:
stderr
- 66%|██████▋ | 186/280 [00:59<00:32, 2.86it/s]
</pre>
- 66%|██████▋ | 186/280 [00:59<00:32, 2.86it/s]
end{sphinxVerbatim}
66%|██████▋ | 186/280 [00:59<00:32, 2.86it/s]
- more-to-come:
- class:
stderr
- 67%|██████▋ | 187/280 [01:00<00:32, 2.84it/s]
</pre>
- 67%|██████▋ | 187/280 [01:00<00:32, 2.84it/s]
end{sphinxVerbatim}
67%|██████▋ | 187/280 [01:00<00:32, 2.84it/s]
- more-to-come:
- class:
stderr
- 67%|██████▋ | 188/280 [01:00<00:34, 2.69it/s]
</pre>
- 67%|██████▋ | 188/280 [01:00<00:34, 2.69it/s]
end{sphinxVerbatim}
67%|██████▋ | 188/280 [01:00<00:34, 2.69it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 189/280 [01:01<00:32, 2.76it/s]
</pre>
- 68%|██████▊ | 189/280 [01:01<00:32, 2.76it/s]
end{sphinxVerbatim}
68%|██████▊ | 189/280 [01:01<00:32, 2.76it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 190/280 [01:01<00:28, 3.11it/s]
</pre>
- 68%|██████▊ | 190/280 [01:01<00:28, 3.11it/s]
end{sphinxVerbatim}
68%|██████▊ | 190/280 [01:01<00:28, 3.11it/s]
- more-to-come:
- class:
stderr
- 68%|██████▊ | 191/280 [01:01<00:32, 2.73it/s]
</pre>
- 68%|██████▊ | 191/280 [01:01<00:32, 2.73it/s]
end{sphinxVerbatim}
68%|██████▊ | 191/280 [01:01<00:32, 2.73it/s]
- more-to-come:
- class:
stderr
- 69%|██████▊ | 192/280 [01:01<00:28, 3.09it/s]
</pre>
- 69%|██████▊ | 192/280 [01:01<00:28, 3.09it/s]
end{sphinxVerbatim}
69%|██████▊ | 192/280 [01:01<00:28, 3.09it/s]
- more-to-come:
- class:
stderr
- 69%|██████▉ | 193/280 [01:02<00:25, 3.45it/s]
</pre>
- 69%|██████▉ | 193/280 [01:02<00:25, 3.45it/s]
end{sphinxVerbatim}
69%|██████▉ | 193/280 [01:02<00:25, 3.45it/s]
- more-to-come:
- class:
stderr
- 69%|██████▉ | 194/280 [01:02<00:26, 3.30it/s]
</pre>
- 69%|██████▉ | 194/280 [01:02<00:26, 3.30it/s]
end{sphinxVerbatim}
69%|██████▉ | 194/280 [01:02<00:26, 3.30it/s]
- more-to-come:
- class:
stderr
- 70%|██████▉ | 195/280 [01:03<00:34, 2.45it/s]
</pre>
- 70%|██████▉ | 195/280 [01:03<00:34, 2.45it/s]
end{sphinxVerbatim}
70%|██████▉ | 195/280 [01:03<00:34, 2.45it/s]
- more-to-come:
- class:
stderr
- 70%|███████ | 196/280 [01:03<00:33, 2.52it/s]
</pre>
- 70%|███████ | 196/280 [01:03<00:33, 2.52it/s]
end{sphinxVerbatim}
70%|███████ | 196/280 [01:03<00:33, 2.52it/s]
- more-to-come:
- class:
stderr
- 70%|███████ | 197/280 [01:03<00:29, 2.85it/s]
</pre>
- 70%|███████ | 197/280 [01:03<00:29, 2.85it/s]
end{sphinxVerbatim}
70%|███████ | 197/280 [01:03<00:29, 2.85it/s]
- more-to-come:
- class:
stderr
- 71%|███████ | 198/280 [01:04<00:26, 3.12it/s]
</pre>
- 71%|███████ | 198/280 [01:04<00:26, 3.12it/s]
end{sphinxVerbatim}
71%|███████ | 198/280 [01:04<00:26, 3.12it/s]
- more-to-come:
- class:
stderr
- 71%|███████ | 199/280 [01:04<00:22, 3.54it/s]
</pre>
- 71%|███████ | 199/280 [01:04<00:22, 3.54it/s]
end{sphinxVerbatim}
71%|███████ | 199/280 [01:04<00:22, 3.54it/s]
- more-to-come:
- class:
stderr
- 71%|███████▏ | 200/280 [01:04<00:21, 3.72it/s]
</pre>
- 71%|███████▏ | 200/280 [01:04<00:21, 3.72it/s]
end{sphinxVerbatim}
71%|███████▏ | 200/280 [01:04<00:21, 3.72it/s]
- more-to-come:
- class:
stderr
- 72%|███████▏ | 201/280 [01:04<00:20, 3.83it/s]
</pre>
- 72%|███████▏ | 201/280 [01:04<00:20, 3.83it/s]
end{sphinxVerbatim}
72%|███████▏ | 201/280 [01:04<00:20, 3.83it/s]
- more-to-come:
- class:
stderr
- 72%|███████▏ | 202/280 [01:04<00:19, 3.90it/s]
</pre>
- 72%|███████▏ | 202/280 [01:04<00:19, 3.90it/s]
end{sphinxVerbatim}
72%|███████▏ | 202/280 [01:04<00:19, 3.90it/s]
- more-to-come:
- class:
stderr
- 72%|███████▎ | 203/280 [01:05<00:18, 4.25it/s]
</pre>
- 72%|███████▎ | 203/280 [01:05<00:18, 4.25it/s]
end{sphinxVerbatim}
72%|███████▎ | 203/280 [01:05<00:18, 4.25it/s]
- more-to-come:
- class:
stderr
- 73%|███████▎ | 204/280 [01:05<00:18, 4.20it/s]
</pre>
- 73%|███████▎ | 204/280 [01:05<00:18, 4.20it/s]
end{sphinxVerbatim}
73%|███████▎ | 204/280 [01:05<00:18, 4.20it/s]
- more-to-come:
- class:
stderr
- 73%|███████▎ | 205/280 [01:05<00:24, 3.12it/s]
</pre>
- 73%|███████▎ | 205/280 [01:05<00:24, 3.12it/s]
end{sphinxVerbatim}
73%|███████▎ | 205/280 [01:05<00:24, 3.12it/s]
- more-to-come:
- class:
stderr
- 74%|███████▎ | 206/280 [01:06<00:21, 3.50it/s]
</pre>
- 74%|███████▎ | 206/280 [01:06<00:21, 3.50it/s]
end{sphinxVerbatim}
74%|███████▎ | 206/280 [01:06<00:21, 3.50it/s]
- more-to-come:
- class:
stderr
- 74%|███████▍ | 207/280 [01:06<00:18, 4.01it/s]
</pre>
- 74%|███████▍ | 207/280 [01:06<00:18, 4.01it/s]
end{sphinxVerbatim}
74%|███████▍ | 207/280 [01:06<00:18, 4.01it/s]
- more-to-come:
- class:
stderr
- 74%|███████▍ | 208/280 [01:06<00:16, 4.24it/s]
</pre>
- 74%|███████▍ | 208/280 [01:06<00:16, 4.24it/s]
end{sphinxVerbatim}
74%|███████▍ | 208/280 [01:06<00:16, 4.24it/s]
- more-to-come:
- class:
stderr
- 75%|███████▍ | 209/280 [01:06<00:19, 3.62it/s]
</pre>
- 75%|███████▍ | 209/280 [01:06<00:19, 3.62it/s]
end{sphinxVerbatim}
75%|███████▍ | 209/280 [01:06<00:19, 3.62it/s]
- more-to-come:
- class:
stderr
- 75%|███████▌ | 210/280 [01:07<00:23, 2.94it/s]
</pre>
- 75%|███████▌ | 210/280 [01:07<00:23, 2.94it/s]
end{sphinxVerbatim}
75%|███████▌ | 210/280 [01:07<00:23, 2.94it/s]
- more-to-come:
- class:
stderr
- 75%|███████▌ | 211/280 [01:07<00:19, 3.50it/s]
</pre>
- 75%|███████▌ | 211/280 [01:07<00:19, 3.50it/s]
end{sphinxVerbatim}
75%|███████▌ | 211/280 [01:07<00:19, 3.50it/s]
- more-to-come:
- class:
stderr
- 76%|███████▌ | 212/280 [01:07<00:17, 3.99it/s]
</pre>
- 76%|███████▌ | 212/280 [01:07<00:17, 3.99it/s]
end{sphinxVerbatim}
76%|███████▌ | 212/280 [01:07<00:17, 3.99it/s]
- more-to-come:
- class:
stderr
- 76%|███████▌ | 213/280 [01:07<00:14, 4.48it/s]
</pre>
- 76%|███████▌ | 213/280 [01:07<00:14, 4.48it/s]
end{sphinxVerbatim}
76%|███████▌ | 213/280 [01:07<00:14, 4.48it/s]
- more-to-come:
- class:
stderr
- 76%|███████▋ | 214/280 [01:08<00:25, 2.57it/s]
</pre>
- 76%|███████▋ | 214/280 [01:08<00:25, 2.57it/s]
end{sphinxVerbatim}
76%|███████▋ | 214/280 [01:08<00:25, 2.57it/s]
- more-to-come:
- class:
stderr
- 77%|███████▋ | 215/280 [01:08<00:22, 2.83it/s]
</pre>
- 77%|███████▋ | 215/280 [01:08<00:22, 2.83it/s]
end{sphinxVerbatim}
77%|███████▋ | 215/280 [01:08<00:22, 2.83it/s]
- more-to-come:
- class:
stderr
- 77%|███████▋ | 216/280 [01:09<00:23, 2.75it/s]
</pre>
- 77%|███████▋ | 216/280 [01:09<00:23, 2.75it/s]
end{sphinxVerbatim}
77%|███████▋ | 216/280 [01:09<00:23, 2.75it/s]
- more-to-come:
- class:
stderr
- 78%|███████▊ | 217/280 [01:09<00:21, 2.98it/s]
</pre>
- 78%|███████▊ | 217/280 [01:09<00:21, 2.98it/s]
end{sphinxVerbatim}
78%|███████▊ | 217/280 [01:09<00:21, 2.98it/s]
- more-to-come:
- class:
stderr
- 78%|███████▊ | 218/280 [01:09<00:18, 3.44it/s]
</pre>
- 78%|███████▊ | 218/280 [01:09<00:18, 3.44it/s]
end{sphinxVerbatim}
78%|███████▊ | 218/280 [01:09<00:18, 3.44it/s]
- more-to-come:
- class:
stderr
- 78%|███████▊ | 219/280 [01:09<00:16, 3.74it/s]
</pre>
- 78%|███████▊ | 219/280 [01:09<00:16, 3.74it/s]
end{sphinxVerbatim}
78%|███████▊ | 219/280 [01:09<00:16, 3.74it/s]
- more-to-come:
- class:
stderr
- 79%|███████▊ | 220/280 [01:10<00:15, 3.90it/s]
</pre>
- 79%|███████▊ | 220/280 [01:10<00:15, 3.90it/s]
end{sphinxVerbatim}
79%|███████▊ | 220/280 [01:10<00:15, 3.90it/s]
- more-to-come:
- class:
stderr
- 79%|███████▉ | 221/280 [01:10<00:17, 3.42it/s]
</pre>
- 79%|███████▉ | 221/280 [01:10<00:17, 3.42it/s]
end{sphinxVerbatim}
79%|███████▉ | 221/280 [01:10<00:17, 3.42it/s]
- more-to-come:
- class:
stderr
- 79%|███████▉ | 222/280 [01:10<00:16, 3.58it/s]
</pre>
- 79%|███████▉ | 222/280 [01:10<00:16, 3.58it/s]
end{sphinxVerbatim}
79%|███████▉ | 222/280 [01:10<00:16, 3.58it/s]
- more-to-come:
- class:
stderr
- 80%|███████▉ | 223/280 [01:11<00:15, 3.78it/s]
</pre>
- 80%|███████▉ | 223/280 [01:11<00:15, 3.78it/s]
end{sphinxVerbatim}
80%|███████▉ | 223/280 [01:11<00:15, 3.78it/s]
- more-to-come:
- class:
stderr
- 80%|████████ | 224/280 [01:11<00:23, 2.42it/s]
</pre>
- 80%|████████ | 224/280 [01:11<00:23, 2.42it/s]
end{sphinxVerbatim}
80%|████████ | 224/280 [01:11<00:23, 2.42it/s]
- more-to-come:
- class:
stderr
- 80%|████████ | 225/280 [01:11<00:19, 2.89it/s]
</pre>
- 80%|████████ | 225/280 [01:11<00:19, 2.89it/s]
end{sphinxVerbatim}
80%|████████ | 225/280 [01:11<00:19, 2.89it/s]
- more-to-come:
- class:
stderr
- 81%|████████ | 226/280 [01:12<00:16, 3.21it/s]
</pre>
- 81%|████████ | 226/280 [01:12<00:16, 3.21it/s]
end{sphinxVerbatim}
81%|████████ | 226/280 [01:12<00:16, 3.21it/s]
- more-to-come:
- class:
stderr
- 81%|████████ | 227/280 [01:12<00:15, 3.47it/s]
</pre>
- 81%|████████ | 227/280 [01:12<00:15, 3.47it/s]
end{sphinxVerbatim}
81%|████████ | 227/280 [01:12<00:15, 3.47it/s]
- more-to-come:
- class:
stderr
- 81%|████████▏ | 228/280 [01:13<00:19, 2.64it/s]
</pre>
- 81%|████████▏ | 228/280 [01:13<00:19, 2.64it/s]
end{sphinxVerbatim}
81%|████████▏ | 228/280 [01:13<00:19, 2.64it/s]
- more-to-come:
- class:
stderr
- 82%|████████▏ | 229/280 [01:13<00:18, 2.81it/s]
</pre>
- 82%|████████▏ | 229/280 [01:13<00:18, 2.81it/s]
end{sphinxVerbatim}
82%|████████▏ | 229/280 [01:13<00:18, 2.81it/s]
- more-to-come:
- class:
stderr
- 82%|████████▏ | 230/280 [01:13<00:15, 3.27it/s]
</pre>
- 82%|████████▏ | 230/280 [01:13<00:15, 3.27it/s]
end{sphinxVerbatim}
82%|████████▏ | 230/280 [01:13<00:15, 3.27it/s]
- more-to-come:
- class:
stderr
- 82%|████████▎ | 231/280 [01:13<00:13, 3.56it/s]
</pre>
- 82%|████████▎ | 231/280 [01:13<00:13, 3.56it/s]
end{sphinxVerbatim}
82%|████████▎ | 231/280 [01:13<00:13, 3.56it/s]
- more-to-come:
- class:
stderr
- 83%|████████▎ | 232/280 [01:13<00:12, 3.84it/s]
</pre>
- 83%|████████▎ | 232/280 [01:13<00:12, 3.84it/s]
end{sphinxVerbatim}
83%|████████▎ | 232/280 [01:13<00:12, 3.84it/s]
- more-to-come:
- class:
stderr
- 83%|████████▎ | 233/280 [01:14<00:14, 3.25it/s]
</pre>
- 83%|████████▎ | 233/280 [01:14<00:14, 3.25it/s]
end{sphinxVerbatim}
83%|████████▎ | 233/280 [01:14<00:14, 3.25it/s]
- more-to-come:
- class:
stderr
- 84%|████████▎ | 234/280 [01:14<00:13, 3.47it/s]
</pre>
- 84%|████████▎ | 234/280 [01:14<00:13, 3.47it/s]
end{sphinxVerbatim}
84%|████████▎ | 234/280 [01:14<00:13, 3.47it/s]
- more-to-come:
- class:
stderr
- 84%|████████▍ | 235/280 [01:14<00:12, 3.66it/s]
</pre>
- 84%|████████▍ | 235/280 [01:14<00:12, 3.66it/s]
end{sphinxVerbatim}
84%|████████▍ | 235/280 [01:14<00:12, 3.66it/s]
- more-to-come:
- class:
stderr
- 84%|████████▍ | 236/280 [01:15<00:14, 3.09it/s]
</pre>
- 84%|████████▍ | 236/280 [01:15<00:14, 3.09it/s]
end{sphinxVerbatim}
84%|████████▍ | 236/280 [01:15<00:14, 3.09it/s]
- more-to-come:
- class:
stderr
- 85%|████████▍ | 237/280 [01:15<00:12, 3.58it/s]
</pre>
- 85%|████████▍ | 237/280 [01:15<00:12, 3.58it/s]
end{sphinxVerbatim}
85%|████████▍ | 237/280 [01:15<00:12, 3.58it/s]
- more-to-come:
- class:
stderr
- 85%|████████▌ | 238/280 [01:15<00:10, 3.99it/s]
</pre>
- 85%|████████▌ | 238/280 [01:15<00:10, 3.99it/s]
end{sphinxVerbatim}
85%|████████▌ | 238/280 [01:15<00:10, 3.99it/s]
- more-to-come:
- class:
stderr
- 85%|████████▌ | 239/280 [01:16<00:16, 2.54it/s]
</pre>
- 85%|████████▌ | 239/280 [01:16<00:16, 2.54it/s]
end{sphinxVerbatim}
85%|████████▌ | 239/280 [01:16<00:16, 2.54it/s]
- more-to-come:
- class:
stderr
- 86%|████████▌ | 240/280 [01:17<00:18, 2.15it/s]
</pre>
- 86%|████████▌ | 240/280 [01:17<00:18, 2.15it/s]
end{sphinxVerbatim}
86%|████████▌ | 240/280 [01:17<00:18, 2.15it/s]
- more-to-come:
- class:
stderr
- 86%|████████▌ | 241/280 [01:17<00:16, 2.32it/s]
</pre>
- 86%|████████▌ | 241/280 [01:17<00:16, 2.32it/s]
end{sphinxVerbatim}
86%|████████▌ | 241/280 [01:17<00:16, 2.32it/s]
- more-to-come:
- class:
stderr
- 86%|████████▋ | 242/280 [01:17<00:13, 2.76it/s]
</pre>
- 86%|████████▋ | 242/280 [01:17<00:13, 2.76it/s]
end{sphinxVerbatim}
86%|████████▋ | 242/280 [01:17<00:13, 2.76it/s]
- more-to-come:
- class:
stderr
- 87%|████████▋ | 243/280 [01:17<00:11, 3.11it/s]
</pre>
- 87%|████████▋ | 243/280 [01:17<00:11, 3.11it/s]
end{sphinxVerbatim}
87%|████████▋ | 243/280 [01:17<00:11, 3.11it/s]
- more-to-come:
- class:
stderr
- 87%|████████▋ | 244/280 [01:18<00:14, 2.55it/s]
</pre>
- 87%|████████▋ | 244/280 [01:18<00:14, 2.55it/s]
end{sphinxVerbatim}
87%|████████▋ | 244/280 [01:18<00:14, 2.55it/s]
- more-to-come:
- class:
stderr
- 88%|████████▊ | 245/280 [01:18<00:15, 2.31it/s]
</pre>
- 88%|████████▊ | 245/280 [01:18<00:15, 2.31it/s]
end{sphinxVerbatim}
88%|████████▊ | 245/280 [01:18<00:15, 2.31it/s]
- more-to-come:
- class:
stderr
- 88%|████████▊ | 246/280 [01:19<00:13, 2.52it/s]
</pre>
- 88%|████████▊ | 246/280 [01:19<00:13, 2.52it/s]
end{sphinxVerbatim}
88%|████████▊ | 246/280 [01:19<00:13, 2.52it/s]
- more-to-come:
- class:
stderr
- 88%|████████▊ | 247/280 [01:19<00:10, 3.03it/s]
</pre>
- 88%|████████▊ | 247/280 [01:19<00:10, 3.03it/s]
end{sphinxVerbatim}
88%|████████▊ | 247/280 [01:19<00:10, 3.03it/s]
- more-to-come:
- class:
stderr
- 89%|████████▊ | 248/280 [01:20<00:14, 2.27it/s]
</pre>
- 89%|████████▊ | 248/280 [01:20<00:14, 2.27it/s]
end{sphinxVerbatim}
89%|████████▊ | 248/280 [01:20<00:14, 2.27it/s]
- more-to-come:
- class:
stderr
- 89%|████████▉ | 249/280 [01:20<00:11, 2.70it/s]
</pre>
- 89%|████████▉ | 249/280 [01:20<00:11, 2.70it/s]
end{sphinxVerbatim}
89%|████████▉ | 249/280 [01:20<00:11, 2.70it/s]
- more-to-come:
- class:
stderr
- 89%|████████▉ | 250/280 [01:20<00:10, 2.92it/s]
</pre>
- 89%|████████▉ | 250/280 [01:20<00:10, 2.92it/s]
end{sphinxVerbatim}
89%|████████▉ | 250/280 [01:20<00:10, 2.92it/s]
- more-to-come:
- class:
stderr
- 90%|████████▉ | 251/280 [01:20<00:08, 3.37it/s]
</pre>
- 90%|████████▉ | 251/280 [01:20<00:08, 3.37it/s]
end{sphinxVerbatim}
90%|████████▉ | 251/280 [01:20<00:08, 3.37it/s]
- more-to-come:
- class:
stderr
- 90%|█████████ | 252/280 [01:20<00:07, 3.70it/s]
</pre>
- 90%|█████████ | 252/280 [01:20<00:07, 3.70it/s]
end{sphinxVerbatim}
90%|█████████ | 252/280 [01:20<00:07, 3.70it/s]
- more-to-come:
- class:
stderr
- 90%|█████████ | 253/280 [01:21<00:06, 4.21it/s]
</pre>
- 90%|█████████ | 253/280 [01:21<00:06, 4.21it/s]
end{sphinxVerbatim}
90%|█████████ | 253/280 [01:21<00:06, 4.21it/s]
- more-to-come:
- class:
stderr
- 91%|█████████ | 254/280 [01:21<00:06, 3.99it/s]
</pre>
- 91%|█████████ | 254/280 [01:21<00:06, 3.99it/s]
end{sphinxVerbatim}
91%|█████████ | 254/280 [01:21<00:06, 3.99it/s]
- more-to-come:
- class:
stderr
- 91%|█████████ | 255/280 [01:21<00:05, 4.36it/s]
</pre>
- 91%|█████████ | 255/280 [01:21<00:05, 4.36it/s]
end{sphinxVerbatim}
91%|█████████ | 255/280 [01:21<00:05, 4.36it/s]
- more-to-come:
- class:
stderr
- 91%|█████████▏| 256/280 [01:21<00:05, 4.36it/s]
</pre>
- 91%|█████████▏| 256/280 [01:21<00:05, 4.36it/s]
end{sphinxVerbatim}
91%|█████████▏| 256/280 [01:21<00:05, 4.36it/s]
- more-to-come:
- class:
stderr
- 92%|█████████▏| 257/280 [01:22<00:07, 3.18it/s]
</pre>
- 92%|█████████▏| 257/280 [01:22<00:07, 3.18it/s]
end{sphinxVerbatim}
92%|█████████▏| 257/280 [01:22<00:07, 3.18it/s]
- more-to-come:
- class:
stderr
- 92%|█████████▏| 258/280 [01:22<00:05, 3.73it/s]
</pre>
- 92%|█████████▏| 258/280 [01:22<00:05, 3.73it/s]
end{sphinxVerbatim}
92%|█████████▏| 258/280 [01:22<00:05, 3.73it/s]
- more-to-come:
- class:
stderr
- 92%|█████████▎| 259/280 [01:22<00:05, 3.55it/s]
</pre>
- 92%|█████████▎| 259/280 [01:22<00:05, 3.55it/s]
end{sphinxVerbatim}
92%|█████████▎| 259/280 [01:22<00:05, 3.55it/s]
- more-to-come:
- class:
stderr
- 93%|█████████▎| 260/280 [01:23<00:05, 3.78it/s]
</pre>
- 93%|█████████▎| 260/280 [01:23<00:05, 3.78it/s]
end{sphinxVerbatim}
93%|█████████▎| 260/280 [01:23<00:05, 3.78it/s]
- more-to-come:
- class:
stderr
- 93%|█████████▎| 261/280 [01:23<00:06, 2.82it/s]
</pre>
- 93%|█████████▎| 261/280 [01:23<00:06, 2.82it/s]
end{sphinxVerbatim}
93%|█████████▎| 261/280 [01:23<00:06, 2.82it/s]
- more-to-come:
- class:
stderr
- 94%|█████████▎| 262/280 [01:23<00:05, 3.22it/s]
</pre>
- 94%|█████████▎| 262/280 [01:23<00:05, 3.22it/s]
end{sphinxVerbatim}
94%|█████████▎| 262/280 [01:23<00:05, 3.22it/s]
- more-to-come:
- class:
stderr
- 94%|█████████▍| 263/280 [01:24<00:04, 3.43it/s]
</pre>
- 94%|█████████▍| 263/280 [01:24<00:04, 3.43it/s]
end{sphinxVerbatim}
94%|█████████▍| 263/280 [01:24<00:04, 3.43it/s]
- more-to-come:
- class:
stderr
- 94%|█████████▍| 264/280 [01:24<00:04, 3.49it/s]
</pre>
- 94%|█████████▍| 264/280 [01:24<00:04, 3.49it/s]
end{sphinxVerbatim}
94%|█████████▍| 264/280 [01:24<00:04, 3.49it/s]
- more-to-come:
- class:
stderr
- 95%|█████████▍| 265/280 [01:24<00:05, 2.68it/s]
</pre>
- 95%|█████████▍| 265/280 [01:24<00:05, 2.68it/s]
end{sphinxVerbatim}
95%|█████████▍| 265/280 [01:24<00:05, 2.68it/s]
- more-to-come:
- class:
stderr
- 95%|█████████▌| 266/280 [01:25<00:05, 2.73it/s]
</pre>
- 95%|█████████▌| 266/280 [01:25<00:05, 2.73it/s]
end{sphinxVerbatim}
95%|█████████▌| 266/280 [01:25<00:05, 2.73it/s]
- more-to-come:
- class:
stderr
- 95%|█████████▌| 267/280 [01:25<00:04, 3.04it/s]
</pre>
- 95%|█████████▌| 267/280 [01:25<00:04, 3.04it/s]
end{sphinxVerbatim}
95%|█████████▌| 267/280 [01:25<00:04, 3.04it/s]
- more-to-come:
- class:
stderr
- 96%|█████████▌| 268/280 [01:26<00:05, 2.17it/s]
</pre>
- 96%|█████████▌| 268/280 [01:26<00:05, 2.17it/s]
end{sphinxVerbatim}
96%|█████████▌| 268/280 [01:26<00:05, 2.17it/s]
- more-to-come:
- class:
stderr
- 96%|█████████▌| 269/280 [01:26<00:04, 2.65it/s]
</pre>
- 96%|█████████▌| 269/280 [01:26<00:04, 2.65it/s]
end{sphinxVerbatim}
96%|█████████▌| 269/280 [01:26<00:04, 2.65it/s]
- more-to-come:
- class:
stderr
- 96%|█████████▋| 270/280 [01:26<00:03, 3.15it/s]
</pre>
- 96%|█████████▋| 270/280 [01:26<00:03, 3.15it/s]
end{sphinxVerbatim}
96%|█████████▋| 270/280 [01:26<00:03, 3.15it/s]
- more-to-come:
- class:
stderr
- 97%|█████████▋| 271/280 [01:26<00:02, 3.01it/s]
</pre>
- 97%|█████████▋| 271/280 [01:26<00:02, 3.01it/s]
end{sphinxVerbatim}
97%|█████████▋| 271/280 [01:26<00:02, 3.01it/s]
- more-to-come:
- class:
stderr
- 97%|█████████▋| 272/280 [01:27<00:02, 2.69it/s]
</pre>
- 97%|█████████▋| 272/280 [01:27<00:02, 2.69it/s]
end{sphinxVerbatim}
97%|█████████▋| 272/280 [01:27<00:02, 2.69it/s]
- more-to-come:
- class:
stderr
- 98%|█████████▊| 273/280 [01:27<00:02, 3.03it/s]
</pre>
- 98%|█████████▊| 273/280 [01:27<00:02, 3.03it/s]
end{sphinxVerbatim}
98%|█████████▊| 273/280 [01:27<00:02, 3.03it/s]
- more-to-come:
- class:
stderr
- 98%|█████████▊| 274/280 [01:27<00:01, 3.45it/s]
</pre>
- 98%|█████████▊| 274/280 [01:27<00:01, 3.45it/s]
end{sphinxVerbatim}
98%|█████████▊| 274/280 [01:27<00:01, 3.45it/s]
- more-to-come:
- class:
stderr
- 98%|█████████▊| 275/280 [01:28<00:01, 3.50it/s]
</pre>
- 98%|█████████▊| 275/280 [01:28<00:01, 3.50it/s]
end{sphinxVerbatim}
98%|█████████▊| 275/280 [01:28<00:01, 3.50it/s]
- more-to-come:
- class:
stderr
- 99%|█████████▊| 276/280 [01:28<00:01, 3.76it/s]
</pre>
- 99%|█████████▊| 276/280 [01:28<00:01, 3.76it/s]
end{sphinxVerbatim}
99%|█████████▊| 276/280 [01:28<00:01, 3.76it/s]
- more-to-come:
- class:
stderr
- 99%|█████████▉| 277/280 [01:28<00:00, 3.96it/s]
</pre>
- 99%|█████████▉| 277/280 [01:28<00:00, 3.96it/s]
end{sphinxVerbatim}
99%|█████████▉| 277/280 [01:28<00:00, 3.96it/s]
- more-to-come:
- class:
stderr
- 99%|█████████▉| 278/280 [01:28<00:00, 4.12it/s]
</pre>
- 99%|█████████▉| 278/280 [01:28<00:00, 4.12it/s]
end{sphinxVerbatim}
99%|█████████▉| 278/280 [01:28<00:00, 4.12it/s]
- 100%|█████████▉| 279/280 [01:29<00:00, 4.21it/s]
</pre>
- 100%|█████████▉| 279/280 [01:29<00:00, 4.21it/s]
end{sphinxVerbatim}
100%|█████████▉| 279/280 [01:29<00:00, 4.21it/s]
- 100%|██████████| 280/280 [01:29<00:00, 3.46it/s]
</pre>
- 100%|██████████| 280/280 [01:29<00:00, 3.46it/s]
end{sphinxVerbatim}
100%|██████████| 280/280 [01:29<00:00, 3.46it/s]
- 100%|██████████| 280/280 [01:29<00:00, 3.13it/s]
</pre>
- 100%|██████████| 280/280 [01:29<00:00, 3.13it/s]
end{sphinxVerbatim}
100%|██████████| 280/280 [01:29<00:00, 3.13it/s]
Use the model with pymagglobal¶
Finally, we load the model and compare it to other models by using pymagglobal.
[5]:
from paleokalmag import PaleoKalmag
archkalmag14k = PaleoKalmag(
'./ArchKalmag14k_smoothed.npz',
name='ArchKalmag14k',
)
[6]:
from matplotlib import pyplot as plt
from pymagglobal import Model, dipole_series
plt.rcParams['font.size'] = '14'
fig, ax = plt.subplots(1, 1, figsize=(10, 6))
a14ktimes = np.linspace(-12000, 1900, 1001)
dip, a16, a84 = dipole_series(a14ktimes, archkalmag14k)
ax.plot(a14ktimes, dip, color='C0', zorder=2, label=archkalmag14k.name)
ax.fill_between(
a14ktimes,
a16,
a84,
color='C0',
alpha=0.4,
zorder=0,
)
arch10k = Model('ARCH10k.1')
# different times due to different valid interval
a10ktimes = np.linspace(-8000, 1900, 1001)
dip = dipole_series(a10ktimes, arch10k)
ax.plot(a10ktimes, dip, color='C1', zorder=2, label=arch10k.name)
ax.legend();
ax.set_ylabel(r'Dipole moment [$10^{22}$Am$^2$]');
ax.set_xlabel(r'time [yrs.]');
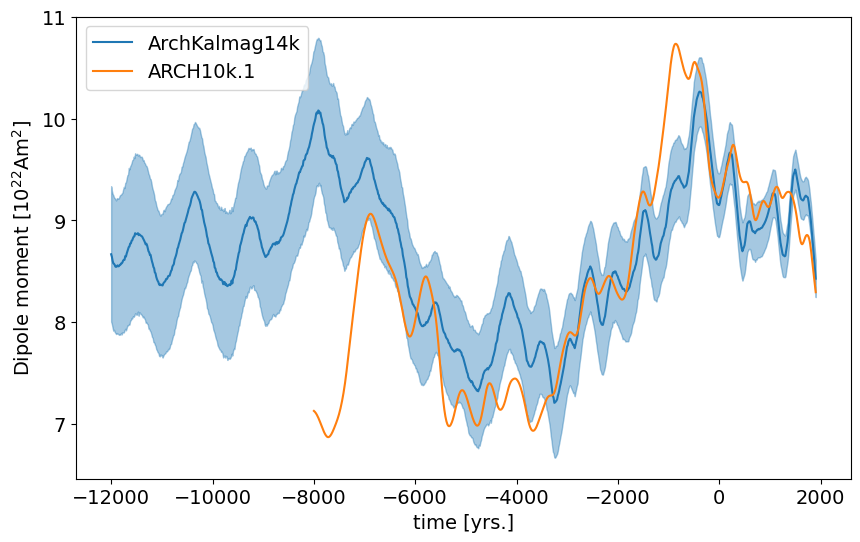